Port halfway to fabric, still few packages left
Signed-off-by: liach <liach@users.noreply.github.com>
This commit is contained in:
parent
0ec938690c
commit
527f9b63c2
1
FeatureManager-fabric
Submodule
1
FeatureManager-fabric
Submodule
@ -0,0 +1 @@
|
||||
Subproject commit 9ed441098a5132d93b3cdb01cea1a2dcecaf2eb9
|
23
TerraForgedAPI-fabric/README.md
Normal file
23
TerraForgedAPI-fabric/README.md
Normal file
@ -0,0 +1,23 @@
|
||||
# TerraForgedAPI
|
||||
|
||||
### Dependency
|
||||
```groovy
|
||||
repositories {
|
||||
maven {
|
||||
url "https://io.terraforged.com/repository/maven/"
|
||||
}
|
||||
}
|
||||
|
||||
dependencies {
|
||||
implementation "com.terraforged:TerraForgedAPI:1.15.2-0.0.1"
|
||||
}
|
||||
```
|
||||
|
||||
### Usage
|
||||
|
||||
TerraForged fires a number of setup events each time its chunk generator is created. These events expose certain
|
||||
components of the generator allowing for world-gen content to be configured, modified, or added to.
|
||||
|
||||
See the `com.terraforged.api.TerraEvent` class for the available events.
|
||||
|
||||
All events are fired on the `FORGE` event bus.
|
59
TerraForgedAPI-fabric/build.gradle
Normal file
59
TerraForgedAPI-fabric/build.gradle
Normal file
@ -0,0 +1,59 @@
|
||||
buildscript {
|
||||
repositories {
|
||||
maven { url "https://maven.fabricmc.net/" }
|
||||
jcenter()
|
||||
}
|
||||
dependencies {
|
||||
classpath "net.fabricmc:fabric-loom:0.2.7-SNAPSHOT"
|
||||
}
|
||||
}
|
||||
|
||||
apply plugin: "fabric-loom"
|
||||
apply plugin: "maven-publish"
|
||||
apply plugin: "eclipse"
|
||||
|
||||
version = "${mc_version}-${mod_version}"
|
||||
archivesBaseName = "TerraForgedAPI-fabric"
|
||||
|
||||
repositories {
|
||||
jcenter()
|
||||
mavenCentral()
|
||||
}
|
||||
|
||||
dependencies {
|
||||
minecraft "com.mojang:minecraft:${mc_version}"
|
||||
mappings "net.fabricmc:yarn:${mc_version}+build.${yarn_build}:v2"
|
||||
modImplementation "net.fabricmc:fabric-loader:${fabric_loader_version}"
|
||||
modImplementation "net.fabricmc.fabric-api:fabric-api:${fabric_api_version}"
|
||||
compile project(":Noise2D")
|
||||
compile project(":FeatureManager-fabric")
|
||||
compile project(":TerraForgedCore")
|
||||
}
|
||||
|
||||
jar {
|
||||
from("$buildDir/classes/java/main")
|
||||
from(project(":Noise2D").buildDir.getPath() + "/classes/java/main")
|
||||
from(project(":FeatureManager-fabric").buildDir.getPath() + "/classes/java/main")
|
||||
from(project(":TerraForgedCore").buildDir.getPath() + "/classes/java/main")
|
||||
}
|
||||
|
||||
publishing {
|
||||
publications {
|
||||
mavenJava(MavenPublication) {
|
||||
artifact jar
|
||||
}
|
||||
}
|
||||
|
||||
if (System.getenv("MAVEN_USER") != null && System.getenv("MAVEN_PASS") != null) {
|
||||
repositories {
|
||||
maven {
|
||||
credentials {
|
||||
username System.getenv("MAVEN_USER")
|
||||
password System.getenv("MAVEN_PASS")
|
||||
}
|
||||
name = "nexus"
|
||||
url = "https://io.terraforged.com/repository/maven/"
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
@ -0,0 +1,121 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.api.biome;
|
||||
|
||||
import com.terraforged.core.world.biome.BiomeType;
|
||||
import net.minecraft.tag.Tag;
|
||||
import net.minecraft.tag.TagContainer;
|
||||
import net.minecraft.util.Identifier;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
|
||||
import java.util.Collection;
|
||||
import java.util.EnumMap;
|
||||
import java.util.Map;
|
||||
import java.util.Optional;
|
||||
|
||||
public class BiomeTags {
|
||||
|
||||
private static int generation;
|
||||
private static TagContainer<Biome> collection = new TagContainer<>(
|
||||
path -> Optional.empty(),
|
||||
"",
|
||||
false,
|
||||
""
|
||||
);
|
||||
|
||||
private static final Map<BiomeType, BiomeWrapper> tags = new EnumMap<>(BiomeType.class);
|
||||
|
||||
public static final Tag<Biome> ALPINE = tag(BiomeType.ALPINE);
|
||||
public static final Tag<Biome> COLD_STEPPE = tag(BiomeType.COLD_STEPPE);
|
||||
public static final Tag<Biome> DESERT = tag(BiomeType.DESERT);
|
||||
public static final Tag<Biome> GRASSLAND = tag(BiomeType.GRASSLAND);
|
||||
public static final Tag<Biome> SAVANNA = tag(BiomeType.SAVANNA);
|
||||
public static final Tag<Biome> STEPPE = tag(BiomeType.STEPPE);
|
||||
public static final Tag<Biome> TAIGA = tag(BiomeType.TAIGA);
|
||||
public static final Tag<Biome> TEMPERATE_FOREST = tag(BiomeType.TEMPERATE_FOREST);
|
||||
public static final Tag<Biome> TEMPERATE_RAINFOREST = tag(BiomeType.TEMPERATE_RAINFOREST);
|
||||
public static final Tag<Biome> TROPICAL_RAINFOREST = tag(BiomeType.TROPICAL_RAINFOREST);
|
||||
public static final Tag<Biome> TUNDRA = tag(BiomeType.TUNDRA);
|
||||
|
||||
public static Tag<Biome> getTag(BiomeType type) {
|
||||
return tags.get(type);
|
||||
}
|
||||
|
||||
public static void setCollection(TagContainer<Biome> collection) {
|
||||
BiomeTags.collection = collection;
|
||||
BiomeTags.generation++;
|
||||
}
|
||||
|
||||
private static BiomeWrapper tag(BiomeType type) {
|
||||
BiomeWrapper wrapper = tag(type.name().toLowerCase());
|
||||
tags.put(type, wrapper);
|
||||
return wrapper;
|
||||
}
|
||||
|
||||
private static BiomeWrapper tag(String name) {
|
||||
return new BiomeWrapper(new Identifier("terraforged", name));
|
||||
}
|
||||
|
||||
private static class BiomeWrapper extends Tag<Biome> {
|
||||
|
||||
private Tag<Biome> cachedTag = null;
|
||||
private int lastKnownGeneration = -1;
|
||||
|
||||
public BiomeWrapper(Identifier name) {
|
||||
super(name);
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean contains(Biome biome) {
|
||||
if (this.lastKnownGeneration != BiomeTags.generation) {
|
||||
this.cachedTag = BiomeTags.collection.getOrCreate(this.getId());
|
||||
this.lastKnownGeneration = BiomeTags.generation;
|
||||
}
|
||||
|
||||
return this.cachedTag.contains(biome);
|
||||
}
|
||||
|
||||
@Override
|
||||
public Collection<Biome> values() {
|
||||
if (this.lastKnownGeneration != BiomeTags.generation) {
|
||||
this.cachedTag = BiomeTags.collection.getOrCreate(this.getId());
|
||||
this.lastKnownGeneration = BiomeTags.generation;
|
||||
}
|
||||
|
||||
return this.cachedTag.values();
|
||||
}
|
||||
|
||||
@Override
|
||||
public Collection<Tag.Entry<Biome>> entries() {
|
||||
if (this.lastKnownGeneration != BiomeTags.generation) {
|
||||
this.cachedTag = BiomeTags.collection.getOrCreate(this.getId());
|
||||
this.lastKnownGeneration = BiomeTags.generation;
|
||||
}
|
||||
|
||||
return this.cachedTag.entries();
|
||||
}
|
||||
}
|
||||
}
|
@ -0,0 +1,52 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.api.biome;
|
||||
|
||||
import net.minecraft.world.biome.Biome;
|
||||
|
||||
public abstract class BiomeVariant extends Biome {
|
||||
|
||||
protected BiomeVariant(Settings biomeBuilder) {
|
||||
super(biomeBuilder);
|
||||
}
|
||||
|
||||
@Override
|
||||
public int getGrassColorAt(double x, double z) {
|
||||
return getBase().getGrassColorAt(x, z);
|
||||
}
|
||||
|
||||
@Override
|
||||
public int getFoliageColor() {
|
||||
return getBase().getFoliageColor();
|
||||
}
|
||||
|
||||
@Override
|
||||
public int getSkyColor() {
|
||||
return getBase().getSkyColor();
|
||||
}
|
||||
|
||||
public abstract Biome getBase();
|
||||
}
|
@ -0,0 +1,67 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.api.biome.modifier;
|
||||
|
||||
import com.terraforged.core.cell.Cell;
|
||||
import com.terraforged.core.util.Seed;
|
||||
import com.terraforged.core.world.climate.Climate;
|
||||
import com.terraforged.core.world.terrain.Terrain;
|
||||
import me.dags.noise.Module;
|
||||
import me.dags.noise.Source;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
|
||||
public abstract class AbstractMaxHeightModifier extends AbstractOffsetModifier {
|
||||
|
||||
private final float minHeight;
|
||||
private final float maxHeight;
|
||||
private final float range;
|
||||
private final Module variance;
|
||||
|
||||
public AbstractMaxHeightModifier(Seed seed, Climate climate, int scale, int octaves, float variance, float minHeight, float maxHeight) {
|
||||
super(climate);
|
||||
this.minHeight = minHeight;
|
||||
this.maxHeight = maxHeight;
|
||||
this.range = maxHeight - minHeight;
|
||||
this.variance = Source.perlin(seed.next(), scale, octaves).scale(variance);
|
||||
}
|
||||
|
||||
@Override
|
||||
protected final Biome modify(Biome in, Cell<Terrain> cell, int x, int z, float ox, float oz) {
|
||||
float var = variance.getValue(x, z);
|
||||
float value = cell.value + var;
|
||||
if (value < minHeight) {
|
||||
return in;
|
||||
}
|
||||
if (value > maxHeight) {
|
||||
return getModifiedBiome(in, cell, x, z, ox, oz);
|
||||
}
|
||||
float alpha = (value - minHeight) / range;
|
||||
cell.biomeEdge *= alpha;
|
||||
return in;
|
||||
}
|
||||
|
||||
protected abstract Biome getModifiedBiome(Biome in, Cell<Terrain> cell, int x, int z, float ox, float oz);
|
||||
}
|
@ -0,0 +1,49 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.api.biome.modifier;
|
||||
|
||||
import com.terraforged.core.cell.Cell;
|
||||
import com.terraforged.core.world.climate.Climate;
|
||||
import com.terraforged.core.world.terrain.Terrain;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
|
||||
public abstract class AbstractOffsetModifier implements BiomeModifier {
|
||||
|
||||
private final Climate climate;
|
||||
|
||||
public AbstractOffsetModifier(Climate climate) {
|
||||
this.climate = climate;
|
||||
}
|
||||
|
||||
@Override
|
||||
public Biome modify(Biome in, Cell<Terrain> cell, int x, int z) {
|
||||
float dx = climate.getOffsetX(x, z, 50);
|
||||
float dz = climate.getOffsetX(x, z, 50);
|
||||
return modify(in, cell, x, z, x + dx, z + dz);
|
||||
}
|
||||
|
||||
protected abstract Biome modify(Biome in, Cell<Terrain> cell, int x, int z, float ox, float oz);
|
||||
}
|
@ -0,0 +1,45 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.api.biome.modifier;
|
||||
|
||||
import com.terraforged.core.cell.Cell;
|
||||
import com.terraforged.core.world.terrain.Terrain;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
|
||||
public interface BiomeModifier extends Comparable<BiomeModifier> {
|
||||
|
||||
int priority();
|
||||
|
||||
boolean test(Biome biome);
|
||||
|
||||
Biome modify(Biome in, Cell<Terrain> cell, int x, int z);
|
||||
|
||||
@Override
|
||||
default int compareTo(BiomeModifier other) {
|
||||
// reverse order
|
||||
return Integer.compare(other.priority(), priority());
|
||||
}
|
||||
}
|
@ -0,0 +1,31 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.api.biome.modifier;
|
||||
|
||||
public interface ModifierManager {
|
||||
|
||||
void register(BiomeModifier modifier);
|
||||
}
|
@ -0,0 +1,48 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.api.chunk;
|
||||
|
||||
import net.minecraft.world.chunk.Chunk;
|
||||
import net.minecraft.world.gen.ChunkRandom;
|
||||
|
||||
public class ChunkContext {
|
||||
|
||||
public final int chunkX;
|
||||
public final int chunkZ;
|
||||
public final int blockX;
|
||||
public final int blockZ;
|
||||
public final Chunk chunk;
|
||||
public final ChunkRandom random = new ChunkRandom();
|
||||
|
||||
public ChunkContext(Chunk chunk) {
|
||||
this.chunk = chunk;
|
||||
this.chunkX = chunk.getPos().x;
|
||||
this.chunkZ = chunk.getPos().z;
|
||||
this.blockX = chunkX << 4;
|
||||
this.blockZ = chunkZ << 4;
|
||||
this.random.setSeed(chunkX, chunkZ);
|
||||
}
|
||||
}
|
@ -0,0 +1,308 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.api.chunk;
|
||||
|
||||
import it.unimi.dsi.fastutil.longs.LongSet;
|
||||
import it.unimi.dsi.fastutil.shorts.ShortList;
|
||||
import net.minecraft.block.Block;
|
||||
import net.minecraft.block.BlockState;
|
||||
import net.minecraft.block.entity.BlockEntity;
|
||||
import net.minecraft.entity.Entity;
|
||||
import net.minecraft.fluid.Fluid;
|
||||
import net.minecraft.fluid.FluidState;
|
||||
import net.minecraft.nbt.CompoundTag;
|
||||
import net.minecraft.structure.StructureStart;
|
||||
import net.minecraft.util.hit.BlockHitResult;
|
||||
import net.minecraft.util.math.BlockPos;
|
||||
import net.minecraft.util.math.ChunkPos;
|
||||
import net.minecraft.util.math.Vec3d;
|
||||
import net.minecraft.util.shape.VoxelShape;
|
||||
import net.minecraft.world.Heightmap;
|
||||
import net.minecraft.world.RayTraceContext;
|
||||
import net.minecraft.world.TickScheduler;
|
||||
import net.minecraft.world.biome.source.BiomeArray;
|
||||
import net.minecraft.world.chunk.Chunk;
|
||||
import net.minecraft.world.chunk.ChunkSection;
|
||||
import net.minecraft.world.chunk.ChunkStatus;
|
||||
import net.minecraft.world.chunk.UpgradeData;
|
||||
import net.minecraft.world.gen.GenerationStep;
|
||||
|
||||
import java.util.BitSet;
|
||||
import java.util.Collection;
|
||||
import java.util.Map;
|
||||
import java.util.Set;
|
||||
import java.util.stream.Stream;
|
||||
|
||||
public interface ChunkDelegate extends Chunk {
|
||||
|
||||
Chunk getDelegate();
|
||||
|
||||
@Override
|
||||
//@Nullable
|
||||
default BlockState setBlockState(BlockPos pos, BlockState state, boolean isMoving) {
|
||||
return getDelegate().setBlockState(pos, state, isMoving);
|
||||
}
|
||||
|
||||
@Override
|
||||
default void setBlockEntity(BlockPos pos, BlockEntity tileEntityIn) {
|
||||
getDelegate().setBlockEntity(pos, tileEntityIn);
|
||||
}
|
||||
|
||||
@Override
|
||||
default void addEntity(Entity entityIn) {
|
||||
getDelegate().addEntity(entityIn);
|
||||
}
|
||||
|
||||
@Override
|
||||
//@Nullable
|
||||
default ChunkSection getHighestNonEmptySection() {
|
||||
return getDelegate().getHighestNonEmptySection();
|
||||
}
|
||||
|
||||
@Override
|
||||
default int getHighestNonEmptySectionYOffset() {
|
||||
return getDelegate().getHighestNonEmptySectionYOffset();
|
||||
}
|
||||
|
||||
@Override
|
||||
default Set<BlockPos> getBlockEntityPositions() {
|
||||
return getDelegate().getBlockEntityPositions();
|
||||
}
|
||||
|
||||
@Override
|
||||
default ChunkSection[] getSectionArray() {
|
||||
return getDelegate().getSectionArray();
|
||||
}
|
||||
|
||||
|
||||
@Override
|
||||
default Collection<Map.Entry<Heightmap.Type, Heightmap>> getHeightmaps() {
|
||||
return getDelegate().getHeightmaps();
|
||||
}
|
||||
|
||||
@Override
|
||||
default void setHeightmap(Heightmap.Type type, long[] data) {
|
||||
getDelegate().setHeightmap(type, data);
|
||||
}
|
||||
|
||||
@Override
|
||||
default Heightmap getHeightmap(Heightmap.Type type) {
|
||||
return getDelegate().getHeightmap(type);
|
||||
}
|
||||
|
||||
@Override
|
||||
default int sampleHeightmap(Heightmap.Type heightmapType, int x, int z) {
|
||||
return getDelegate().sampleHeightmap(heightmapType, x, z);
|
||||
}
|
||||
|
||||
@Override
|
||||
default ChunkPos getPos() {
|
||||
return getDelegate().getPos();
|
||||
}
|
||||
|
||||
@Override
|
||||
default void setLastSaveTime(long saveTime) {
|
||||
getDelegate().setLastSaveTime(saveTime);
|
||||
}
|
||||
|
||||
@Override
|
||||
default Map<String, StructureStart> getStructureStarts() {
|
||||
return getDelegate().getStructureStarts();
|
||||
}
|
||||
|
||||
@Override
|
||||
default void setStructureStarts(Map<String, StructureStart> structureStartsIn) {
|
||||
getDelegate().setStructureStarts(structureStartsIn);
|
||||
}
|
||||
|
||||
@Override
|
||||
default boolean method_12228(int startY, int endY) {
|
||||
return getDelegate().method_12228(startY, endY);
|
||||
}
|
||||
|
||||
@Override
|
||||
//@Nullable
|
||||
default BiomeArray getBiomeArray() {
|
||||
return getDelegate().getBiomeArray();
|
||||
}
|
||||
|
||||
@Override
|
||||
default void setLightOn(boolean modified) {
|
||||
getDelegate().setLightOn(modified);
|
||||
}
|
||||
|
||||
@Override
|
||||
default boolean isLightOn() {
|
||||
return getDelegate().isLightOn();
|
||||
}
|
||||
|
||||
@Override
|
||||
default ChunkStatus getStatus() {
|
||||
return getDelegate().getStatus();
|
||||
}
|
||||
|
||||
@Override
|
||||
default void removeBlockEntity(BlockPos pos) {
|
||||
getDelegate().removeBlockEntity(pos);
|
||||
}
|
||||
|
||||
@Override
|
||||
default void markBlockForPostProcessing(BlockPos pos) {
|
||||
getDelegate().markBlockForPostProcessing(pos);
|
||||
}
|
||||
|
||||
@Override
|
||||
default ShortList[] getPostProcessingLists() {
|
||||
return getDelegate().getPostProcessingLists();
|
||||
}
|
||||
|
||||
@Override
|
||||
default void markBlockForPostProcessing(short packedPosition, int index) {
|
||||
getDelegate().markBlockForPostProcessing(packedPosition, index);
|
||||
}
|
||||
|
||||
@Override
|
||||
default void addPendingBlockEntityTag(CompoundTag nbt) {
|
||||
getDelegate().addPendingBlockEntityTag(nbt);
|
||||
}
|
||||
|
||||
@Override
|
||||
//@Nullable
|
||||
default CompoundTag method_20598(BlockPos pos) {
|
||||
return getDelegate().method_20598(pos);
|
||||
}
|
||||
|
||||
@Override
|
||||
//@Nullable
|
||||
default CompoundTag getBlockEntityTagAt(BlockPos pos) {
|
||||
return getDelegate().getBlockEntityTagAt(pos);
|
||||
}
|
||||
|
||||
@Override
|
||||
default Stream<BlockPos> getLightSourcesStream() {
|
||||
return getDelegate().getLightSourcesStream();
|
||||
}
|
||||
|
||||
@Override
|
||||
default TickScheduler<Block> getBlockTickScheduler() {
|
||||
return getDelegate().getBlockTickScheduler();
|
||||
}
|
||||
|
||||
@Override
|
||||
default TickScheduler<Fluid> getFluidTickScheduler() {
|
||||
return getDelegate().getFluidTickScheduler();
|
||||
}
|
||||
|
||||
@Override
|
||||
default BitSet getCarvingMask(GenerationStep.Carver type) {
|
||||
return getDelegate().getCarvingMask(type);
|
||||
}
|
||||
|
||||
@Override
|
||||
default void setShouldSave(boolean shouldSave) {
|
||||
getDelegate().setShouldSave(shouldSave);
|
||||
}
|
||||
|
||||
@Override
|
||||
default boolean needsSaving() {
|
||||
return getDelegate().needsSaving();
|
||||
}
|
||||
|
||||
@Override
|
||||
default void setStructureStart(String structure, StructureStart start) {
|
||||
getDelegate().setStructureStart(structure, start);
|
||||
}
|
||||
|
||||
@Override
|
||||
default int getLuminance(BlockPos pos) {
|
||||
return getDelegate().getLuminance(pos);
|
||||
}
|
||||
|
||||
@Override
|
||||
default UpgradeData getUpgradeData() {
|
||||
return getDelegate().getUpgradeData();
|
||||
}
|
||||
|
||||
@Override
|
||||
default void setInhabitedTime(long inhabitedTime) {
|
||||
getDelegate().setInhabitedTime(inhabitedTime);
|
||||
}
|
||||
|
||||
@Override
|
||||
default long getInhabitedTime() {
|
||||
return getDelegate().getInhabitedTime();
|
||||
}
|
||||
|
||||
@Override
|
||||
default BlockEntity getBlockEntity(BlockPos pos) {
|
||||
return getDelegate().getBlockEntity(pos);
|
||||
}
|
||||
|
||||
@Override
|
||||
default BlockState getBlockState(BlockPos pos) {
|
||||
return getDelegate().getBlockState(pos);
|
||||
}
|
||||
|
||||
@Override
|
||||
default FluidState getFluidState(BlockPos pos) {
|
||||
return getDelegate().getFluidState(pos);
|
||||
}
|
||||
|
||||
@Override
|
||||
default StructureStart getStructureStart(String structure) {
|
||||
return getDelegate().getStructureStart(structure);
|
||||
}
|
||||
|
||||
@Override
|
||||
default LongSet getStructureReferences(String structure) {
|
||||
return getDelegate().getStructureReferences(structure);
|
||||
}
|
||||
|
||||
@Override
|
||||
default void addStructureReference(String structure, long reference) {
|
||||
getDelegate().addStructureReference(structure, reference);
|
||||
}
|
||||
|
||||
@Override
|
||||
default Map<String, LongSet> getStructureReferences() {
|
||||
return getDelegate().getStructureReferences();
|
||||
}
|
||||
|
||||
@Override
|
||||
default void setStructureReferences(Map<String, LongSet> structureReferences) {
|
||||
getDelegate().setStructureReferences(structureReferences);
|
||||
}
|
||||
|
||||
@Override
|
||||
default int getMaxLightLevel() {
|
||||
return getDelegate().getMaxLightLevel();
|
||||
}
|
||||
|
||||
@Override
|
||||
default int getHeight() {
|
||||
return getDelegate().getHeight();
|
||||
}
|
||||
}
|
@ -0,0 +1,63 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.api.chunk.column;
|
||||
|
||||
import com.terraforged.api.chunk.surface.ChunkSurfaceBuffer;
|
||||
import me.dags.noise.Source;
|
||||
import me.dags.noise.source.FastSource;
|
||||
import net.minecraft.block.BlockState;
|
||||
import net.minecraft.util.math.BlockPos;
|
||||
import net.minecraft.world.chunk.Chunk;
|
||||
import net.minecraft.world.chunk.Chunk;
|
||||
|
||||
public interface ColumnDecorator {
|
||||
|
||||
FastSource variance = (FastSource) Source.perlin(0, 100, 1);
|
||||
|
||||
void decorate(Chunk chunk, DecoratorContext context, int x, int y, int z);
|
||||
|
||||
default void decorate(ChunkSurfaceBuffer buffer, DecoratorContext context, int x, int y, int z) {
|
||||
decorate(buffer.getDelegate(), context, x, y, z);
|
||||
}
|
||||
|
||||
default void setState(Chunk chunk, int x, int y, int z, BlockState state, boolean moving) {
|
||||
chunk.setBlockState(new BlockPos(x, y, z), state, moving);
|
||||
}
|
||||
|
||||
default void fillDown(DecoratorContext context, Chunk chunk, int x, int z, int from, int to, BlockState state) {
|
||||
for (int dy = from; dy > to; dy--) {
|
||||
chunk.setBlockState(context.pos.set(x, dy, z), state, false);
|
||||
}
|
||||
}
|
||||
|
||||
static float getNoise(float x, float z, int seed, float scale, float bias) {
|
||||
return (variance.getValue(x, z, seed) * scale) + bias;
|
||||
}
|
||||
|
||||
static float getNoise(float x, float z, int seed, int scale, int bias) {
|
||||
return getNoise(x, z, seed, scale / 255F, bias / 255F);
|
||||
}
|
||||
}
|
@ -0,0 +1,54 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.api.chunk.column;
|
||||
|
||||
import com.terraforged.api.chunk.ChunkContext;
|
||||
import com.terraforged.core.cell.Cell;
|
||||
import com.terraforged.core.world.climate.Climate;
|
||||
import com.terraforged.core.world.heightmap.Levels;
|
||||
import com.terraforged.core.world.terrain.Terrain;
|
||||
import com.terraforged.core.world.terrain.Terrains;
|
||||
import net.minecraft.util.math.BlockPos;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
import net.minecraft.world.chunk.Chunk;
|
||||
|
||||
public class DecoratorContext extends ChunkContext {
|
||||
|
||||
public final Levels levels;
|
||||
public final Climate climate;
|
||||
public final Terrains terrains;
|
||||
public final BlockPos.Mutable pos = new BlockPos.Mutable();
|
||||
|
||||
public Biome biome;
|
||||
public Cell<Terrain> cell;
|
||||
|
||||
public DecoratorContext(Chunk chunk, Levels levels, Terrains terrain, Climate climate) {
|
||||
super(chunk);
|
||||
this.levels = levels;
|
||||
this.climate = climate;
|
||||
this.terrains = terrain;
|
||||
}
|
||||
}
|
@ -0,0 +1,47 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.api.chunk.column;
|
||||
|
||||
import java.util.List;
|
||||
|
||||
public class DecoratorManager {
|
||||
|
||||
private final List<ColumnDecorator> baseDecorators;
|
||||
private final List<ColumnDecorator> featureDecorators;
|
||||
|
||||
public DecoratorManager(List<ColumnDecorator> baseDecorators, List<ColumnDecorator> featureDecorators) {
|
||||
this.baseDecorators = baseDecorators;
|
||||
this.featureDecorators = featureDecorators;
|
||||
}
|
||||
|
||||
public void registerBaseDecorator(ColumnDecorator decorator) {
|
||||
baseDecorators.add(decorator);
|
||||
}
|
||||
|
||||
public void registerFeatureDecorator(ColumnDecorator decorator) {
|
||||
featureDecorators.add(decorator);
|
||||
}
|
||||
}
|
@ -0,0 +1,34 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.api.chunk.surface;
|
||||
|
||||
import net.minecraft.world.biome.Biome;
|
||||
|
||||
public class CachedSurface {
|
||||
|
||||
public Biome biome;
|
||||
public Surface surface;
|
||||
}
|
@ -0,0 +1,72 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.api.chunk.surface;
|
||||
|
||||
import com.terraforged.api.chunk.ChunkDelegate;
|
||||
import net.minecraft.block.BlockState;
|
||||
import net.minecraft.util.math.BlockPos;
|
||||
import net.minecraft.world.chunk.Chunk;
|
||||
|
||||
public class ChunkSurfaceBuffer implements ChunkDelegate {
|
||||
|
||||
private int surfaceTop;
|
||||
private int surfaceBottom;
|
||||
private final Chunk delegate;
|
||||
|
||||
public ChunkSurfaceBuffer(Chunk chunk) {
|
||||
this.delegate = chunk;
|
||||
}
|
||||
|
||||
@Override
|
||||
public Chunk getDelegate() {
|
||||
return delegate;
|
||||
}
|
||||
|
||||
//@Nullable
|
||||
@Override
|
||||
public BlockState setBlockState(BlockPos pos, BlockState state, boolean isMoving) {
|
||||
if (pos.getY() > surfaceTop) {
|
||||
surfaceTop = pos.getY();
|
||||
}
|
||||
if (pos.getY() < surfaceBottom) {
|
||||
surfaceBottom = pos.getY();
|
||||
}
|
||||
return getDelegate().setBlockState(pos, state, isMoving);
|
||||
}
|
||||
|
||||
public int getSurfaceTop() {
|
||||
return surfaceTop;
|
||||
}
|
||||
|
||||
public int getSurfaceBottom() {
|
||||
return surfaceBottom;
|
||||
}
|
||||
|
||||
public void setSurfaceLevel(int y) {
|
||||
surfaceTop = y;
|
||||
surfaceBottom = y;
|
||||
}
|
||||
}
|
@ -0,0 +1,52 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.api.chunk.surface;
|
||||
|
||||
|
||||
import com.terraforged.api.chunk.surface.builder.Combiner;
|
||||
import net.minecraft.block.BlockState;
|
||||
import net.minecraft.world.chunk.Chunk;
|
||||
|
||||
public interface Surface {
|
||||
|
||||
void buildSurface(int x, int z, int height, SurfaceContext ctx);
|
||||
|
||||
default void fill(int x, int z, int start, int end, SurfaceContext ctx, Chunk chunk, BlockState state) {
|
||||
if (start < end) {
|
||||
for (int y = start; y < end; y++) {
|
||||
chunk.setBlockState(ctx.pos.set(x, y, z), state, false);
|
||||
}
|
||||
} else if (start > end) {
|
||||
for (int y = start; y > end; y--) {
|
||||
chunk.setBlockState(ctx.pos.set(x, y, z), state, false);
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
default Surface then(Surface next) {
|
||||
return new Combiner(this, next);
|
||||
}
|
||||
}
|
@ -0,0 +1,53 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.api.chunk.surface;
|
||||
|
||||
import com.terraforged.api.chunk.column.DecoratorContext;
|
||||
import com.terraforged.core.world.climate.Climate;
|
||||
import com.terraforged.core.world.heightmap.Levels;
|
||||
import com.terraforged.core.world.terrain.Terrains;
|
||||
import net.minecraft.block.BlockState;
|
||||
import net.minecraft.world.chunk.Chunk;
|
||||
import net.minecraft.world.gen.chunk.ChunkGeneratorConfig;
|
||||
|
||||
public class SurfaceContext extends DecoratorContext {
|
||||
|
||||
public final BlockState solid;
|
||||
public final BlockState fluid;
|
||||
public final int seaLevel;
|
||||
public final long seed;
|
||||
public final CachedSurface cached = new CachedSurface();
|
||||
|
||||
public double noise;
|
||||
|
||||
public SurfaceContext(Chunk chunk, Levels levels, Terrains terrain, Climate climate, ChunkGeneratorConfig settings, long seed) {
|
||||
super(chunk, levels, terrain, climate);
|
||||
this.solid = settings.getDefaultBlock();
|
||||
this.fluid = settings.getDefaultFluid();
|
||||
this.seed = seed;
|
||||
this.seaLevel = levels.waterLevel;
|
||||
}
|
||||
}
|
@ -0,0 +1,60 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.api.chunk.surface;
|
||||
|
||||
import com.terraforged.api.chunk.surface.builder.Delegate;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
|
||||
import java.util.HashMap;
|
||||
import java.util.Map;
|
||||
|
||||
public class SurfaceManager {
|
||||
|
||||
private final Map<Biome, Surface> surfaces = new HashMap<>();
|
||||
|
||||
public SurfaceManager replace(Biome biome, Surface surface) {
|
||||
surfaces.put(biome, surface);
|
||||
return this;
|
||||
}
|
||||
|
||||
public SurfaceManager extend(Biome biome, Surface surface) {
|
||||
Surface result = getOrCreateSurface(biome).then(surface);
|
||||
return replace(biome, result);
|
||||
}
|
||||
|
||||
public Surface getSurface(SurfaceContext context) {
|
||||
if (context.biome == context.cached.biome) {
|
||||
return context.cached.surface;
|
||||
}
|
||||
context.cached.biome = context.biome;
|
||||
context.cached.surface = getOrCreateSurface(context.biome);
|
||||
return context.cached.surface;
|
||||
}
|
||||
|
||||
public Surface getOrCreateSurface(Biome biome) {
|
||||
return surfaces.computeIfAbsent(biome, Delegate.FUNC);
|
||||
}
|
||||
}
|
@ -0,0 +1,46 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.api.chunk.surface.builder;
|
||||
|
||||
import com.terraforged.api.chunk.surface.Surface;
|
||||
import com.terraforged.api.chunk.surface.SurfaceContext;
|
||||
|
||||
public class Combiner implements Surface {
|
||||
|
||||
private final Surface first;
|
||||
private final Surface second;
|
||||
|
||||
public Combiner(Surface first, Surface second) {
|
||||
this.first = first;
|
||||
this.second = second;
|
||||
}
|
||||
|
||||
@Override
|
||||
public void buildSurface(int x, int z, int height, SurfaceContext ctx) {
|
||||
first.buildSurface(x, z, height, ctx);
|
||||
second.buildSurface(x, z, height, ctx);
|
||||
}
|
||||
}
|
@ -0,0 +1,67 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.api.chunk.surface.builder;
|
||||
|
||||
import com.terraforged.api.chunk.surface.Surface;
|
||||
import com.terraforged.api.chunk.surface.SurfaceContext;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
import net.minecraft.world.gen.surfacebuilders.ConfiguredSurfaceBuilder;
|
||||
|
||||
import java.util.function.Function;
|
||||
|
||||
public class Delegate implements Surface {
|
||||
|
||||
public static final Function<Biome, Surface> FUNC = Delegate::new;
|
||||
|
||||
private final ConfiguredSurfaceBuilder<?> surfaceBuilder;
|
||||
|
||||
private Delegate(Biome biome) {
|
||||
this(biome.getSurfaceBuilder());
|
||||
}
|
||||
|
||||
private Delegate(ConfiguredSurfaceBuilder<?> surfaceBuilder) {
|
||||
this.surfaceBuilder = surfaceBuilder;
|
||||
}
|
||||
|
||||
@Override
|
||||
public void buildSurface(int x, int z, int height, SurfaceContext context) {
|
||||
surfaceBuilder.setSeed(context.seed);
|
||||
|
||||
surfaceBuilder.buildSurface(
|
||||
context.random,
|
||||
context.chunk,
|
||||
context.biome,
|
||||
x,
|
||||
z,
|
||||
height,
|
||||
context.noise,
|
||||
context.solid,
|
||||
context.fluid,
|
||||
context.seaLevel,
|
||||
context.seed
|
||||
);
|
||||
}
|
||||
}
|
@ -0,0 +1,107 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.api.event;
|
||||
|
||||
import com.terraforged.api.biome.modifier.ModifierManager;
|
||||
import com.terraforged.api.chunk.column.DecoratorManager;
|
||||
import com.terraforged.api.chunk.surface.SurfaceManager;
|
||||
import com.terraforged.api.material.geology.GeologyManager;
|
||||
import com.terraforged.api.material.layer.LayerManager;
|
||||
import com.terraforged.core.world.GeneratorContext;
|
||||
import com.terraforged.core.world.terrain.provider.TerrainProvider;
|
||||
import com.terraforged.feature.modifier.FeatureModifiers;
|
||||
import net.fabricmc.fabric.api.event.Event;
|
||||
import net.fabricmc.fabric.api.event.EventFactory;
|
||||
|
||||
@FunctionalInterface
|
||||
public interface SetupEvent<T> {
|
||||
/**
|
||||
* Can be used to register custom biome Surface decorators
|
||||
*/
|
||||
Event<SetupEvent<SurfaceManager>> SURFACE = EventFactory.createArrayBacked(SetupEvent.class, handlers -> (manager, context) -> {
|
||||
for (SetupEvent<SurfaceManager> handler : handlers) {
|
||||
handler.handle(manager, context);
|
||||
}
|
||||
});
|
||||
/**
|
||||
* Register additional layer blocks (such as Snow Layers)
|
||||
*/
|
||||
Event<SetupEvent<LayerManager>> LAYERS = EventFactory.createArrayBacked(SetupEvent.class, handlers -> (manager, context) -> {
|
||||
for (SetupEvent<LayerManager> handler : handlers) {
|
||||
handler.handle(manager, context);
|
||||
}
|
||||
});
|
||||
/**
|
||||
* Register custom Strata and Geologies
|
||||
*/
|
||||
Event<SetupEvent<GeologyManager>> GEOLOGY = EventFactory.createArrayBacked(SetupEvent.class, handlers -> (manager, context) -> {
|
||||
for (SetupEvent<GeologyManager> handler : handlers) {
|
||||
handler.handle(manager, context);
|
||||
}
|
||||
});
|
||||
/**
|
||||
* Register custom BiomeModifiers
|
||||
*/
|
||||
Event<SetupEvent<ModifierManager>> BIOME_MODIFIER = EventFactory.createArrayBacked(SetupEvent.class, handlers -> (manager, context) -> {
|
||||
for (SetupEvent<ModifierManager> handler : handlers) {
|
||||
handler.handle(manager, context);
|
||||
}
|
||||
});
|
||||
/**
|
||||
* Register custom FeatureModifiers
|
||||
*/
|
||||
Event<SetupEvent<FeatureModifiers>> FEATURES = EventFactory.createArrayBacked(SetupEvent.class, handlers -> (manager, context) -> {
|
||||
for (SetupEvent<FeatureModifiers> handler : handlers) {
|
||||
handler.handle(manager, context);
|
||||
}
|
||||
});
|
||||
/**
|
||||
* Register custom Terrain Populators
|
||||
*/
|
||||
Event<SetupEvent<TerrainProvider>> TERRAIN = EventFactory.createArrayBacked(SetupEvent.class, handlers -> (manager, context) -> {
|
||||
for (SetupEvent<TerrainProvider> handler : handlers) {
|
||||
handler.handle(manager, context);
|
||||
}
|
||||
});
|
||||
/**
|
||||
* Register custom ColumnDecorators
|
||||
* - base decorators process chunk columns early in the generation process (before biome surfaces etc)
|
||||
* - feature decorators process chunk columns late in the generation process (after all features have been placed)
|
||||
*/
|
||||
Event<SetupEvent<DecoratorManager>> DECORATORS = EventFactory.createArrayBacked(SetupEvent.class, handlers -> (manager, context) -> {
|
||||
for (SetupEvent<DecoratorManager> handler : handlers) {
|
||||
handler.handle(manager, context);
|
||||
}
|
||||
});
|
||||
|
||||
/**
|
||||
* Handle the setup event.
|
||||
*
|
||||
* @param manager the Manager/Service being setup
|
||||
* @param context the generator setup context
|
||||
*/
|
||||
void handle(T manager, GeneratorContext context);
|
||||
}
|
@ -0,0 +1,50 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.api.material;
|
||||
|
||||
import net.fabricmc.fabric.api.tag.TagRegistry;
|
||||
import net.minecraft.block.Block;
|
||||
import net.minecraft.tag.BlockTags;
|
||||
import net.minecraft.tag.Tag;
|
||||
import net.minecraft.util.Identifier;
|
||||
|
||||
public class MaterialTags {
|
||||
|
||||
public static final Tag<Block> WG_ROCK = tag("rock");
|
||||
public static final Tag<Block> WG_EARTH = tag("earth");
|
||||
public static final Tag<Block> WG_CLAY = tag("clay");
|
||||
public static final Tag<Block> WG_SEDIMENT = tag("sediment");
|
||||
public static final Tag<Block> WG_ORE = tag("ore");
|
||||
public static final Tag<Block> WG_ERODIBLE = tag("erodible");
|
||||
|
||||
public static void init() {
|
||||
|
||||
}
|
||||
|
||||
private static Tag<Block> tag(String name) {
|
||||
return TagRegistry.create(new Identifier("terraforged", name), BlockTags::getContainer);
|
||||
}
|
||||
}
|
@ -0,0 +1,58 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.api.material.geology;
|
||||
|
||||
import com.terraforged.core.world.geology.Geology;
|
||||
import com.terraforged.core.world.geology.Strata;
|
||||
import net.minecraft.block.BlockState;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
|
||||
public interface GeologyManager {
|
||||
|
||||
StrataGenerator getStrataGenerator();
|
||||
|
||||
/**
|
||||
* Register a global strata group (applies to any biome that does not have specific geology defined.
|
||||
*/
|
||||
void register(Strata<BlockState> strata);
|
||||
|
||||
/**
|
||||
* Register a biome specific strata group.
|
||||
*/
|
||||
default void register(Biome biome, Strata<BlockState> strata) {
|
||||
register(biome, strata, false);
|
||||
}
|
||||
|
||||
/**
|
||||
* Register a biome specific strata group.
|
||||
*/
|
||||
void register(Biome biome, Strata<BlockState> strata, boolean inheritGlobal);
|
||||
|
||||
/**
|
||||
* Register/replace a biome-specific geology group
|
||||
*/
|
||||
void register(Biome biome, Geology<BlockState> geology);
|
||||
}
|
@ -0,0 +1,64 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.api.material.geology;
|
||||
|
||||
import me.dags.noise.util.NoiseUtil;
|
||||
|
||||
public class StrataConfig {
|
||||
|
||||
public Config soil = new Config(0, 1, 0.1F, 0.25F);
|
||||
public Config sediment = new Config(0, 2, 0.05F, 0.15F);
|
||||
public Config clay = new Config(0, 2, 0.05F, 0.1F);
|
||||
public Config rock = new Config(10, 30, 0.1F, 1.5F);
|
||||
|
||||
public static class Config {
|
||||
|
||||
public int minLayers;
|
||||
public int maxLayers;
|
||||
public float minDepth;
|
||||
public float maxDepth;
|
||||
|
||||
public Config() {
|
||||
}
|
||||
|
||||
public Config(int minLayers, int maxLayers, float minDepth, float maxDepth) {
|
||||
this.minLayers = minLayers;
|
||||
this.maxLayers = maxLayers;
|
||||
this.minDepth = minDepth;
|
||||
this.maxDepth = maxDepth;
|
||||
}
|
||||
|
||||
public int getLayers(float value) {
|
||||
int range = maxLayers - minLayers;
|
||||
return minLayers + NoiseUtil.round(value * range);
|
||||
}
|
||||
|
||||
public float getDepth(float value) {
|
||||
float range = maxDepth - minDepth;
|
||||
return minDepth + value * range;
|
||||
}
|
||||
}
|
||||
}
|
@ -0,0 +1,34 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.api.material.geology;
|
||||
|
||||
import com.terraforged.core.world.geology.Strata;
|
||||
import net.minecraft.block.BlockState;
|
||||
|
||||
public interface StrataGenerator {
|
||||
|
||||
Strata<BlockState> generate(int seed, int scale, StrataConfig config);
|
||||
}
|
@ -0,0 +1,53 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.api.material.layer;
|
||||
|
||||
import net.minecraft.block.Block;
|
||||
import net.minecraft.block.Blocks;
|
||||
|
||||
import java.util.HashMap;
|
||||
import java.util.Map;
|
||||
|
||||
public class LayerManager {
|
||||
|
||||
private final Map<Block, LayerMaterial> layers = new HashMap<>();
|
||||
|
||||
public LayerManager() {
|
||||
register(LayerMaterial.of(Blocks.SNOW_BLOCK, Blocks.SNOW));
|
||||
}
|
||||
|
||||
public void register(LayerMaterial material) {
|
||||
register(material.getLayerType(), material);
|
||||
}
|
||||
|
||||
public void register(Block block, LayerMaterial material) {
|
||||
layers.put(block, material);
|
||||
}
|
||||
|
||||
public LayerMaterial getMaterial(Block block) {
|
||||
return layers.get(block);
|
||||
}
|
||||
}
|
@ -0,0 +1,135 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.api.material.layer;
|
||||
|
||||
import me.dags.noise.util.NoiseUtil;
|
||||
import net.minecraft.block.Block;
|
||||
import net.minecraft.block.BlockState;
|
||||
import net.minecraft.block.Blocks;
|
||||
import net.minecraft.state.property.Property;
|
||||
import net.minecraft.state.property.Properties;
|
||||
|
||||
public class LayerMaterial {
|
||||
|
||||
private static final BlockState AIR = Blocks.AIR.getDefaultState();
|
||||
|
||||
private final int min;
|
||||
private final int max;
|
||||
private final BlockState fullState;
|
||||
private final BlockState layerState;
|
||||
private final Property<Integer> layerProperty;
|
||||
|
||||
private LayerMaterial(BlockState fullState, BlockState layerState, Property<Integer> layerProperty) {
|
||||
this.layerProperty = layerProperty;
|
||||
this.min = min(layerProperty);
|
||||
this.max = max(layerProperty);
|
||||
this.layerState = layerState;
|
||||
this.fullState = fullState;
|
||||
}
|
||||
|
||||
public Block getLayerType() {
|
||||
return layerState.getBlock();
|
||||
}
|
||||
|
||||
public BlockState getFull() {
|
||||
return fullState;
|
||||
}
|
||||
|
||||
public BlockState getState(float value) {
|
||||
return getState(getLevel(value));
|
||||
}
|
||||
|
||||
public BlockState getState(int level) {
|
||||
if (level < min) {
|
||||
return LayerMaterial.AIR;
|
||||
}
|
||||
if (level >= max) {
|
||||
return fullState;
|
||||
}
|
||||
return layerState.with(layerProperty, level);
|
||||
}
|
||||
|
||||
public int getMin() {
|
||||
return min;
|
||||
}
|
||||
|
||||
public int getMax() {
|
||||
return max;
|
||||
}
|
||||
|
||||
public int getLevel(float depth) {
|
||||
if (depth > 1) {
|
||||
depth = getDepth(depth);
|
||||
} else if (depth < 0) {
|
||||
depth = 0;
|
||||
}
|
||||
return NoiseUtil.round(depth * max);
|
||||
}
|
||||
|
||||
public float getDepth(float height) {
|
||||
return height - (int) height;
|
||||
}
|
||||
|
||||
private static int min(Property<Integer> property) {
|
||||
return property.getValues().stream().min(Integer::compareTo).orElse(0);
|
||||
}
|
||||
|
||||
private static int max(Property<Integer> property) {
|
||||
return property.getValues().stream().max(Integer::compareTo).orElse(0);
|
||||
}
|
||||
|
||||
public static LayerMaterial of(Block block) {
|
||||
return of(block, Properties.LAYERS);
|
||||
}
|
||||
|
||||
public static LayerMaterial of(Block block, Property<Integer> property) {
|
||||
return of(block.getDefaultState(), block.getDefaultState(), property);
|
||||
}
|
||||
|
||||
public static LayerMaterial of(Block full, Block layer) {
|
||||
return of(full.getDefaultState(), layer.getDefaultState());
|
||||
}
|
||||
|
||||
public static LayerMaterial of(Block full, Block layer, Property<Integer> property) {
|
||||
return of(full.getDefaultState(), layer.getDefaultState(), property);
|
||||
}
|
||||
|
||||
public static LayerMaterial of(BlockState layer) {
|
||||
return of(layer, Properties.LAYERS);
|
||||
}
|
||||
|
||||
public static LayerMaterial of(BlockState layer, Property<Integer> property) {
|
||||
return of(layer.with(property, max(property)), layer);
|
||||
}
|
||||
|
||||
public static LayerMaterial of(BlockState full, BlockState layer) {
|
||||
return of(full, layer, Properties.LAYERS);
|
||||
}
|
||||
|
||||
public static LayerMaterial of(BlockState full, BlockState layer, Property<Integer> property) {
|
||||
return new LayerMaterial(full, layer, property);
|
||||
}
|
||||
}
|
@ -0,0 +1,65 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.api.material.state;
|
||||
|
||||
import net.minecraft.block.BlockState;
|
||||
|
||||
import java.util.ArrayList;
|
||||
import java.util.List;
|
||||
import java.util.function.Supplier;
|
||||
|
||||
public class CachedState extends StateSupplier {
|
||||
|
||||
private static final List<CachedState> all = new ArrayList<>();
|
||||
|
||||
private final Supplier<BlockState> supplier;
|
||||
|
||||
private volatile BlockState reference = null;
|
||||
|
||||
public CachedState(Supplier<BlockState> supplier) {
|
||||
this.supplier = supplier;
|
||||
all.add(this);
|
||||
}
|
||||
|
||||
@Override
|
||||
public BlockState get() {
|
||||
BlockState state = reference;
|
||||
if (state == null) {
|
||||
state = supplier.get();
|
||||
reference = state;
|
||||
}
|
||||
return state;
|
||||
}
|
||||
|
||||
public CachedState clear() {
|
||||
reference = null;
|
||||
return this;
|
||||
}
|
||||
|
||||
public static void clearAll() {
|
||||
all.forEach(CachedState::clear);
|
||||
}
|
||||
}
|
@ -0,0 +1,58 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.api.material.state;
|
||||
|
||||
import net.minecraft.block.Block;
|
||||
import net.minecraft.block.BlockState;
|
||||
import net.minecraft.util.Identifier;
|
||||
import net.minecraft.util.registry.Registry;
|
||||
|
||||
public class DefaultState extends StateSupplier {
|
||||
|
||||
private final Identifier name;
|
||||
|
||||
private DefaultState(Identifier name) {
|
||||
this.name = name;
|
||||
}
|
||||
|
||||
public CachedState cache() {
|
||||
return new CachedState(this);
|
||||
}
|
||||
|
||||
@Override
|
||||
public BlockState get() {
|
||||
Block block = Registry.BLOCK.get(name);
|
||||
return block.getDefaultState();
|
||||
}
|
||||
|
||||
public static DefaultState of(String name) {
|
||||
return of(new Identifier(name));
|
||||
}
|
||||
|
||||
public static DefaultState of(Identifier name) {
|
||||
return new DefaultState(name);
|
||||
}
|
||||
}
|
@ -0,0 +1,42 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.api.material.state;
|
||||
|
||||
import net.minecraft.block.Block;
|
||||
import net.minecraft.block.BlockState;
|
||||
|
||||
import java.util.function.Supplier;
|
||||
|
||||
public abstract class StateSupplier implements Supplier<BlockState> {
|
||||
|
||||
public Block getBlock() {
|
||||
return get().getBlock();
|
||||
}
|
||||
|
||||
public BlockState getDefaultState() {
|
||||
return getBlock().getDefaultState();
|
||||
}
|
||||
}
|
@ -0,0 +1,48 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.api.material.state;
|
||||
|
||||
public class States {
|
||||
|
||||
public static final StateSupplier BEDROCK = DefaultState.of("minecraft:bedrock").cache();
|
||||
public static final StateSupplier COARSE_DIRT = DefaultState.of("minecraft:coarse_dirt").cache();
|
||||
public static final StateSupplier DIRT = DefaultState.of("minecraft:dirt").cache();
|
||||
public static final StateSupplier GRASS_BLOCK = DefaultState.of("minecraft:grass_block").cache();
|
||||
public static final StateSupplier CLAY = DefaultState.of("minecraft:clay").cache();
|
||||
public static final StateSupplier GRAVEL = DefaultState.of("minecraft:gravel").cache();
|
||||
public static final StateSupplier LAVA = DefaultState.of("minecraft:lava").cache();
|
||||
public static final StateSupplier PACKED_ICE = DefaultState.of("minecraft:packed_ice").cache();
|
||||
public static final StateSupplier RED_SANDSTONE = DefaultState.of("minecraft:red_sandstone").cache();
|
||||
public static final StateSupplier SAND = DefaultState.of("minecraft:sand").cache();
|
||||
public static final StateSupplier SANDSTONE = DefaultState.of("minecraft:sandstone").cache();
|
||||
public static final StateSupplier SNOW_BLOCK = DefaultState.of("minecraft:snow_block").cache();
|
||||
public static final StateSupplier STONE = DefaultState.of("minecraft:stone").cache();
|
||||
public static final StateSupplier WATER = DefaultState.of("minecraft:water").cache();
|
||||
|
||||
public static void invalidate() {
|
||||
CachedState.clearAll();
|
||||
}
|
||||
}
|
21
TerraForgedAPI-fabric/src/main/resources/license.txt
Normal file
21
TerraForgedAPI-fabric/src/main/resources/license.txt
Normal file
@ -0,0 +1,21 @@
|
||||
MIT License
|
||||
|
||||
Copyright (c) 2020 TerraForged
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
of this software and associated documentation files (the "Software"), to deal
|
||||
in the Software without restriction, including without limitation the rights
|
||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the Software is
|
||||
furnished to do so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in all
|
||||
copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
SOFTWARE.
|
56
TerraForgedMod-fabric/README.md
Normal file
56
TerraForgedMod-fabric/README.md
Normal file
@ -0,0 +1,56 @@
|
||||
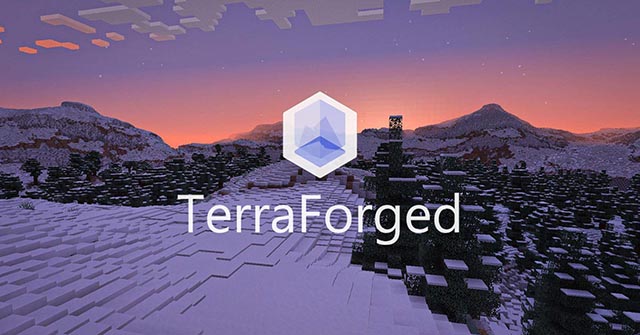
|
||||
|
||||
#### About:
|
||||
TerraForged is an ambitious new terrain generator mod for Minecraft (Java Edition) attempting to
|
||||
create more immersive, inspiring worlds to explore and build in. Featuring an overhaul of the
|
||||
vanilla generation system, custom terrain shapes, simulated erosion, better rivers, custom
|
||||
decorations, tonnes of configuration options, and more!
|
||||
|
||||
#### Website(s):
|
||||
[https://terraforged.com](https://terraforged.com)
|
||||
[https://github.com/TerraForged](https://github.com/TerraForged)
|
||||
[https://curseforge.com/../TerraForged](https://www.curseforge.com/minecraft/mc-mods/terraforged)
|
||||
|
||||
#### Installation:
|
||||
1. Install forge for the target version of Minecraft (ie 1.15.2)
|
||||
2. Add the TerraForged mod jar to your profile's mods folder
|
||||
3. Select the '`TerraForged`' world-type when creating a new world
|
||||
|
||||
#### Features:
|
||||
- Varied and immersive terrain
|
||||
- Erosion and improved rivers
|
||||
- Custom features and decoration
|
||||
- Extensive configuration options & in-game GUI
|
||||
|
||||
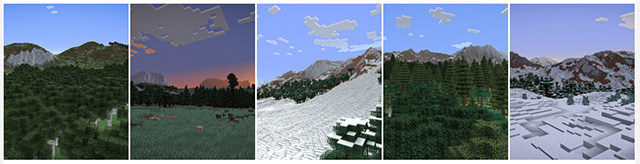
|
||||
|
||||
#### FAQ:
|
||||
1) "Is this compatible with mod xyz?"
|
||||
_Probably! (to some degree) - TerraForged is designed to work with many of the same world-gen systems
|
||||
that the majority of block & biome providing mods use. Certain biomes' terrain may not always look
|
||||
exactly as their author designed but should otherwise be compatible. Feel free to report any other
|
||||
compatibility issues on the issue tracker._
|
||||
|
||||
2) "How can I use this on my server?"
|
||||
_When Forge supports it, you can simply set level-type=terraforged in your server.properties file. In
|
||||
the meantime, you will need to create the world in single player and then copy that to your server
|
||||
directory. (In both cases, TerraForged must be installed on the client and the server)._
|
||||
|
||||
3) "Will I need a super-computer to run this?!"
|
||||
_No, not really - while this world generator will be a bit slower than vanilla's (on account of it
|
||||
doing more work to make things look nice), it would only be apparent when first generating a chunk -
|
||||
they might load in slower when moving to new parts of the world, but game performance should
|
||||
otherwise be normal. A 4-core CPU should be able to handle this just fine._
|
||||
|
||||
4) "Can this be ported Fabric/Bukkit/Spigot/Sponge?"
|
||||
_If someone would like to take this task on, yes - a large part of the TerraForged codebase is already
|
||||
platform independent. There are certain client-side features in the forge-mod that would not translate
|
||||
onto server-only APIs, but the core experience could certainly be ported. I don't intend to work on
|
||||
this directly but others are very welcome._
|
||||
|
||||
5) "Will this be back-ported to older Forge versions?"
|
||||
_Not by myself, no - My aim is to keep current with Forge. I'm simply not prolific enough a modder to
|
||||
write and maintain for multiple versions (hats off to those who do!). Again though, others are welcome
|
||||
to back-port it, if inclined to do so._
|
||||
|
||||
[View more questions on Github](https://github.com/TerraForged/TerraForged/issues?q=label:question)
|
84
TerraForgedMod-fabric/build.gradle
Normal file
84
TerraForgedMod-fabric/build.gradle
Normal file
@ -0,0 +1,84 @@
|
||||
buildscript {
|
||||
repositories {
|
||||
maven { url "https://maven.fabricmc.net/" }
|
||||
jcenter()
|
||||
}
|
||||
dependencies {
|
||||
classpath "net.fabricmc:fabric-loom:0.2.7-SNAPSHOT"
|
||||
}
|
||||
}
|
||||
|
||||
apply plugin: "fabric-loom"
|
||||
apply plugin: "maven-publish"
|
||||
apply plugin: "eclipse"
|
||||
|
||||
version = "${mc_version}-${mod_version}${getClassifier()}"
|
||||
archivesBaseName = "TerraForged"
|
||||
|
||||
repositories {
|
||||
jcenter()
|
||||
mavenCentral()
|
||||
}
|
||||
|
||||
dependencies {
|
||||
minecraft "com.mojang:minecraft:${mc_version}"
|
||||
mappings "net.fabricmc:yarn:${mc_version}+build.${yarn_build}:v2"
|
||||
modImplementation "net.fabricmc:fabric-loader:${fabric_loader_version}"
|
||||
modImplementation "net.fabricmc.fabric-api:fabric-api:${fabric_api_version}"
|
||||
compile project(":Noise2D")
|
||||
compile (project(":TerraForgedCore")) {
|
||||
transitive false
|
||||
}
|
||||
compile (project(":FeatureManager-fabric")) {
|
||||
transitive false
|
||||
}
|
||||
compile (project(":TerraForgedAPI-fabric")) {
|
||||
transitive false
|
||||
}
|
||||
}
|
||||
|
||||
task collectClasses(type: Copy) {
|
||||
configurations.collectMany { it.allDependencies }.findAll{ it instanceof ProjectDependency }.each {
|
||||
ProjectDependency project = (ProjectDependency) it
|
||||
from("$project.dependencyProject.buildDir/classes/java/main")
|
||||
}
|
||||
into("build/classes/java/main")
|
||||
}
|
||||
|
||||
task collectResources(type: Copy) {
|
||||
configurations.collectMany { it.allDependencies }.findAll{ it instanceof ProjectDependency }.each {
|
||||
ProjectDependency project = (ProjectDependency) it
|
||||
from("$project.dependencyProject.buildDir/resources/main")
|
||||
}
|
||||
into("build/resources/main")
|
||||
}
|
||||
|
||||
processResources {
|
||||
dependsOn(collectResources)
|
||||
|
||||
filesMatching("**/fabric.mod.json") {
|
||||
expand(
|
||||
"version": "${mod_version}${getClassifier()}",
|
||||
"mc_version": mc_version
|
||||
)
|
||||
}
|
||||
}
|
||||
|
||||
classes {
|
||||
dependsOn(collectClasses)
|
||||
}
|
||||
|
||||
publish {
|
||||
dependsOn remapJar
|
||||
}
|
||||
|
||||
static def getClassifier() {
|
||||
if (System.getenv("GIT_TAG_NAME") != null) {
|
||||
return ""
|
||||
}
|
||||
def buildNumber = System.getenv("BUILD_NUMBER")
|
||||
if (buildNumber != null) {
|
||||
return "-${buildNumber}"
|
||||
}
|
||||
return ""
|
||||
}
|
172
TerraForgedMod-fabric/gradlew
vendored
Normal file
172
TerraForgedMod-fabric/gradlew
vendored
Normal file
@ -0,0 +1,172 @@
|
||||
#!/usr/bin/env sh
|
||||
|
||||
##############################################################################
|
||||
##
|
||||
## Gradle start up script for UN*X
|
||||
##
|
||||
##############################################################################
|
||||
|
||||
# Attempt to set APP_HOME
|
||||
# Resolve links: $0 may be a link
|
||||
PRG="$0"
|
||||
# Need this for relative symlinks.
|
||||
while [ -h "$PRG" ] ; do
|
||||
ls=`ls -ld "$PRG"`
|
||||
link=`expr "$ls" : '.*-> \(.*\)$'`
|
||||
if expr "$link" : '/.*' > /dev/null; then
|
||||
PRG="$link"
|
||||
else
|
||||
PRG=`dirname "$PRG"`"/$link"
|
||||
fi
|
||||
done
|
||||
SAVED="`pwd`"
|
||||
cd "`dirname \"$PRG\"`/" >/dev/null
|
||||
APP_HOME="`pwd -P`"
|
||||
cd "$SAVED" >/dev/null
|
||||
|
||||
APP_NAME="Gradle"
|
||||
APP_BASE_NAME=`basename "$0"`
|
||||
|
||||
# Add default JVM options here. You can also use JAVA_OPTS and GRADLE_OPTS to pass JVM options to this script.
|
||||
DEFAULT_JVM_OPTS=""
|
||||
|
||||
# Use the maximum available, or set MAX_FD != -1 to use that value.
|
||||
MAX_FD="maximum"
|
||||
|
||||
warn () {
|
||||
echo "$*"
|
||||
}
|
||||
|
||||
die () {
|
||||
echo
|
||||
echo "$*"
|
||||
echo
|
||||
exit 1
|
||||
}
|
||||
|
||||
# OS specific support (must be 'true' or 'false').
|
||||
cygwin=false
|
||||
msys=false
|
||||
darwin=false
|
||||
nonstop=false
|
||||
case "`uname`" in
|
||||
CYGWIN* )
|
||||
cygwin=true
|
||||
;;
|
||||
Darwin* )
|
||||
darwin=true
|
||||
;;
|
||||
MINGW* )
|
||||
msys=true
|
||||
;;
|
||||
NONSTOP* )
|
||||
nonstop=true
|
||||
;;
|
||||
esac
|
||||
|
||||
CLASSPATH=$APP_HOME/gradle/wrapper/gradle-wrapper.jar
|
||||
|
||||
# Determine the Java command to use to start the JVM.
|
||||
if [ -n "$JAVA_HOME" ] ; then
|
||||
if [ -x "$JAVA_HOME/jre/sh/java" ] ; then
|
||||
# IBM's JDK on AIX uses strange locations for the executables
|
||||
JAVACMD="$JAVA_HOME/jre/sh/java"
|
||||
else
|
||||
JAVACMD="$JAVA_HOME/bin/java"
|
||||
fi
|
||||
if [ ! -x "$JAVACMD" ] ; then
|
||||
die "ERROR: JAVA_HOME is set to an invalid directory: $JAVA_HOME
|
||||
|
||||
Please set the JAVA_HOME variable in your environment to match the
|
||||
location of your Java installation."
|
||||
fi
|
||||
else
|
||||
JAVACMD="java"
|
||||
which java >/dev/null 2>&1 || die "ERROR: JAVA_HOME is not set and no 'java' command could be found in your PATH.
|
||||
|
||||
Please set the JAVA_HOME variable in your environment to match the
|
||||
location of your Java installation."
|
||||
fi
|
||||
|
||||
# Increase the maximum file descriptors if we can.
|
||||
if [ "$cygwin" = "false" -a "$darwin" = "false" -a "$nonstop" = "false" ] ; then
|
||||
MAX_FD_LIMIT=`ulimit -H -n`
|
||||
if [ $? -eq 0 ] ; then
|
||||
if [ "$MAX_FD" = "maximum" -o "$MAX_FD" = "max" ] ; then
|
||||
MAX_FD="$MAX_FD_LIMIT"
|
||||
fi
|
||||
ulimit -n $MAX_FD
|
||||
if [ $? -ne 0 ] ; then
|
||||
warn "Could not set maximum file descriptor limit: $MAX_FD"
|
||||
fi
|
||||
else
|
||||
warn "Could not query maximum file descriptor limit: $MAX_FD_LIMIT"
|
||||
fi
|
||||
fi
|
||||
|
||||
# For Darwin, add options to specify how the application appears in the dock
|
||||
if $darwin; then
|
||||
GRADLE_OPTS="$GRADLE_OPTS \"-Xdock:name=$APP_NAME\" \"-Xdock:icon=$APP_HOME/media/gradle.icns\""
|
||||
fi
|
||||
|
||||
# For Cygwin, switch paths to Windows format before running java
|
||||
if $cygwin ; then
|
||||
APP_HOME=`cygpath --path --mixed "$APP_HOME"`
|
||||
CLASSPATH=`cygpath --path --mixed "$CLASSPATH"`
|
||||
JAVACMD=`cygpath --unix "$JAVACMD"`
|
||||
|
||||
# We build the pattern for arguments to be converted via cygpath
|
||||
ROOTDIRSRAW=`find -L / -maxdepth 1 -mindepth 1 -type d 2>/dev/null`
|
||||
SEP=""
|
||||
for dir in $ROOTDIRSRAW ; do
|
||||
ROOTDIRS="$ROOTDIRS$SEP$dir"
|
||||
SEP="|"
|
||||
done
|
||||
OURCYGPATTERN="(^($ROOTDIRS))"
|
||||
# Add a user-defined pattern to the cygpath arguments
|
||||
if [ "$GRADLE_CYGPATTERN" != "" ] ; then
|
||||
OURCYGPATTERN="$OURCYGPATTERN|($GRADLE_CYGPATTERN)"
|
||||
fi
|
||||
# Now convert the arguments - kludge to limit ourselves to /bin/sh
|
||||
i=0
|
||||
for arg in "$@" ; do
|
||||
CHECK=`echo "$arg"|egrep -c "$OURCYGPATTERN" -`
|
||||
CHECK2=`echo "$arg"|egrep -c "^-"` ### Determine if an option
|
||||
|
||||
if [ $CHECK -ne 0 ] && [ $CHECK2 -eq 0 ] ; then ### Added a condition
|
||||
eval `echo args$i`=`cygpath --path --ignore --mixed "$arg"`
|
||||
else
|
||||
eval `echo args$i`="\"$arg\""
|
||||
fi
|
||||
i=$((i+1))
|
||||
done
|
||||
case $i in
|
||||
(0) set -- ;;
|
||||
(1) set -- "$args0" ;;
|
||||
(2) set -- "$args0" "$args1" ;;
|
||||
(3) set -- "$args0" "$args1" "$args2" ;;
|
||||
(4) set -- "$args0" "$args1" "$args2" "$args3" ;;
|
||||
(5) set -- "$args0" "$args1" "$args2" "$args3" "$args4" ;;
|
||||
(6) set -- "$args0" "$args1" "$args2" "$args3" "$args4" "$args5" ;;
|
||||
(7) set -- "$args0" "$args1" "$args2" "$args3" "$args4" "$args5" "$args6" ;;
|
||||
(8) set -- "$args0" "$args1" "$args2" "$args3" "$args4" "$args5" "$args6" "$args7" ;;
|
||||
(9) set -- "$args0" "$args1" "$args2" "$args3" "$args4" "$args5" "$args6" "$args7" "$args8" ;;
|
||||
esac
|
||||
fi
|
||||
|
||||
# Escape application args
|
||||
save () {
|
||||
for i do printf %s\\n "$i" | sed "s/'/'\\\\''/g;1s/^/'/;\$s/\$/' \\\\/" ; done
|
||||
echo " "
|
||||
}
|
||||
APP_ARGS=$(save "$@")
|
||||
|
||||
# Collect all arguments for the java command, following the shell quoting and substitution rules
|
||||
eval set -- $DEFAULT_JVM_OPTS $JAVA_OPTS $GRADLE_OPTS "\"-Dorg.gradle.appname=$APP_BASE_NAME\"" -classpath "\"$CLASSPATH\"" org.gradle.wrapper.GradleWrapperMain "$APP_ARGS"
|
||||
|
||||
# by default we should be in the correct project dir, but when run from Finder on Mac, the cwd is wrong
|
||||
if [ "$(uname)" = "Darwin" ] && [ "$HOME" = "$PWD" ]; then
|
||||
cd "$(dirname "$0")"
|
||||
fi
|
||||
|
||||
exec "$JAVACMD" "$@"
|
84
TerraForgedMod-fabric/gradlew.bat
vendored
Normal file
84
TerraForgedMod-fabric/gradlew.bat
vendored
Normal file
@ -0,0 +1,84 @@
|
||||
@if "%DEBUG%" == "" @echo off
|
||||
@rem ##########################################################################
|
||||
@rem
|
||||
@rem Gradle startup script for Windows
|
||||
@rem
|
||||
@rem ##########################################################################
|
||||
|
||||
@rem Set local scope for the variables with windows NT shell
|
||||
if "%OS%"=="Windows_NT" setlocal
|
||||
|
||||
set DIRNAME=%~dp0
|
||||
if "%DIRNAME%" == "" set DIRNAME=.
|
||||
set APP_BASE_NAME=%~n0
|
||||
set APP_HOME=%DIRNAME%
|
||||
|
||||
@rem Add default JVM options here. You can also use JAVA_OPTS and GRADLE_OPTS to pass JVM options to this script.
|
||||
set DEFAULT_JVM_OPTS=
|
||||
|
||||
@rem Find java.exe
|
||||
if defined JAVA_HOME goto findJavaFromJavaHome
|
||||
|
||||
set JAVA_EXE=java.exe
|
||||
%JAVA_EXE% -version >NUL 2>&1
|
||||
if "%ERRORLEVEL%" == "0" goto init
|
||||
|
||||
echo.
|
||||
echo ERROR: JAVA_HOME is not set and no 'java' command could be found in your PATH.
|
||||
echo.
|
||||
echo Please set the JAVA_HOME variable in your environment to match the
|
||||
echo location of your Java installation.
|
||||
|
||||
goto fail
|
||||
|
||||
:findJavaFromJavaHome
|
||||
set JAVA_HOME=%JAVA_HOME:"=%
|
||||
set JAVA_EXE=%JAVA_HOME%/bin/java.exe
|
||||
|
||||
if exist "%JAVA_EXE%" goto init
|
||||
|
||||
echo.
|
||||
echo ERROR: JAVA_HOME is set to an invalid directory: %JAVA_HOME%
|
||||
echo.
|
||||
echo Please set the JAVA_HOME variable in your environment to match the
|
||||
echo location of your Java installation.
|
||||
|
||||
goto fail
|
||||
|
||||
:init
|
||||
@rem Get command-line arguments, handling Windows variants
|
||||
|
||||
if not "%OS%" == "Windows_NT" goto win9xME_args
|
||||
|
||||
:win9xME_args
|
||||
@rem Slurp the command line arguments.
|
||||
set CMD_LINE_ARGS=
|
||||
set _SKIP=2
|
||||
|
||||
:win9xME_args_slurp
|
||||
if "x%~1" == "x" goto execute
|
||||
|
||||
set CMD_LINE_ARGS=%*
|
||||
|
||||
:execute
|
||||
@rem Setup the command line
|
||||
|
||||
set CLASSPATH=%APP_HOME%\gradle\wrapper\gradle-wrapper.jar
|
||||
|
||||
@rem Execute Gradle
|
||||
"%JAVA_EXE%" %DEFAULT_JVM_OPTS% %JAVA_OPTS% %GRADLE_OPTS% "-Dorg.gradle.appname=%APP_BASE_NAME%" -classpath "%CLASSPATH%" org.gradle.wrapper.GradleWrapperMain %CMD_LINE_ARGS%
|
||||
|
||||
:end
|
||||
@rem End local scope for the variables with windows NT shell
|
||||
if "%ERRORLEVEL%"=="0" goto mainEnd
|
||||
|
||||
:fail
|
||||
rem Set variable GRADLE_EXIT_CONSOLE if you need the _script_ return code instead of
|
||||
rem the _cmd.exe /c_ return code!
|
||||
if not "" == "%GRADLE_EXIT_CONSOLE%" exit 1
|
||||
exit /b 1
|
||||
|
||||
:mainEnd
|
||||
if "%OS%"=="Windows_NT" endlocal
|
||||
|
||||
:omega
|
BIN
TerraForgedMod-fabric/psd/biomes.psd
Normal file
BIN
TerraForgedMod-fabric/psd/biomes.psd
Normal file
Binary file not shown.
BIN
TerraForgedMod-fabric/psd/icon.png
Normal file
BIN
TerraForgedMod-fabric/psd/icon.png
Normal file
Binary file not shown.
After Width: | Height: | Size: 27 KiB |
BIN
TerraForgedMod-fabric/psd/terraforged.png
Normal file
BIN
TerraForgedMod-fabric/psd/terraforged.png
Normal file
Binary file not shown.
After Width: | Height: | Size: 35 KiB |
BIN
TerraForgedMod-fabric/psd/terraforged.psd
Normal file
BIN
TerraForgedMod-fabric/psd/terraforged.psd
Normal file
Binary file not shown.
@ -0,0 +1,46 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod;
|
||||
|
||||
import org.apache.logging.log4j.LogManager;
|
||||
import org.apache.logging.log4j.Logger;
|
||||
|
||||
public class Log {
|
||||
|
||||
private static final Logger logger = LogManager.getLogger("TerraForged");
|
||||
|
||||
public static void info(String message, Object... args) {
|
||||
logger.info(message, args);
|
||||
}
|
||||
|
||||
public static void debug(String message, Object... args) {
|
||||
logger.debug(message, args);
|
||||
}
|
||||
|
||||
public static void err(String message, Object... args) {
|
||||
logger.error(message, args);
|
||||
}
|
||||
}
|
@ -0,0 +1,82 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod;
|
||||
|
||||
import com.terraforged.api.material.MaterialTags;
|
||||
import com.terraforged.core.util.concurrent.ThreadPool;
|
||||
import com.terraforged.feature.FeatureManager;
|
||||
import com.terraforged.mod.biome.tag.BiomeTagManager;
|
||||
import com.terraforged.mod.command.TerraCommand;
|
||||
import com.terraforged.mod.data.DataGen;
|
||||
import com.terraforged.mod.feature.tree.SaplingManager;
|
||||
import com.terraforged.mod.settings.SettingsHelper;
|
||||
import com.terraforged.mod.util.Environment;
|
||||
import net.fabricmc.api.ModInitializer;
|
||||
import net.fabricmc.fabric.api.event.server.ServerStartCallback;
|
||||
import net.fabricmc.fabric.api.event.server.ServerStopCallback;
|
||||
import net.fabricmc.fabric.api.registry.CommandRegistry;
|
||||
import net.fabricmc.fabric.api.resource.ResourceManagerHelper;
|
||||
import net.minecraft.resource.ResourceType;
|
||||
import net.minecraft.server.MinecraftServer;
|
||||
import net.minecraft.world.gen.feature.Feature;
|
||||
import net.minecraftforge.common.MinecraftForge;
|
||||
import net.minecraftforge.event.RegistryEvent;
|
||||
import net.minecraftforge.eventbus.api.SubscribeEvent;
|
||||
import net.minecraftforge.fml.common.Mod;
|
||||
import net.minecraftforge.fml.event.lifecycle.FMLCommonSetupEvent;
|
||||
import net.minecraftforge.fml.event.lifecycle.FMLDedicatedServerSetupEvent;
|
||||
import net.minecraftforge.fml.event.lifecycle.FMLLoadCompleteEvent;
|
||||
import net.minecraftforge.fml.event.server.FMLServerStoppingEvent;
|
||||
|
||||
/**
|
||||
* Author <dags@dags.me>
|
||||
*/
|
||||
public class TerraForgedMod implements ModInitializer {
|
||||
|
||||
@Override
|
||||
public void onInitialize() {
|
||||
Log.info("Common setup");
|
||||
ServerStopCallback.EVENT.register(TerraForgedMod::onShutdown);
|
||||
MaterialTags.init();
|
||||
TerraWorld.init();
|
||||
SaplingManager.init();
|
||||
if (Environment.isDev()) {
|
||||
DataGen.dumpData();
|
||||
}
|
||||
FeatureManager.registerTemplates();
|
||||
ResourceManagerHelper.get(ResourceType.SERVER_DATA).registerReloadListener(new BiomeTagManager());
|
||||
CommandRegistry.INSTANCE.register(false, TerraCommand::register);
|
||||
}
|
||||
|
||||
public static void server() {
|
||||
Log.info("Setting dedicated server");
|
||||
SettingsHelper.setDedicatedServer();
|
||||
}
|
||||
|
||||
private static void onShutdown(MinecraftServer server) {
|
||||
ThreadPool.shutdownCurrent();
|
||||
}
|
||||
}
|
@ -0,0 +1,126 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod;
|
||||
|
||||
import com.terraforged.core.world.terrain.Terrains;
|
||||
import com.terraforged.mod.biome.provider.BiomeProvider;
|
||||
import com.terraforged.mod.chunk.ChunkGeneratorFactory;
|
||||
import com.terraforged.mod.chunk.TerraChunkGenerator;
|
||||
import com.terraforged.mod.chunk.TerraContext;
|
||||
import com.terraforged.mod.chunk.TerraGenSettings;
|
||||
import com.terraforged.mod.chunk.test.TestChunkGenerator;
|
||||
import com.terraforged.mod.gui.SettingsScreen;
|
||||
import com.terraforged.mod.settings.SettingsHelper;
|
||||
import com.terraforged.mod.settings.TerraSettings;
|
||||
import com.terraforged.mod.util.nbt.NBTHelper;
|
||||
import net.minecraft.client.Minecraft;
|
||||
import net.minecraft.client.gui.screen.CreateWorldScreen;
|
||||
import net.minecraft.world.IWorld;
|
||||
import net.minecraft.world.World;
|
||||
import net.minecraft.world.WorldType;
|
||||
import net.minecraft.world.biome.provider.OverworldBiomeProviderSettings;
|
||||
import net.minecraft.world.dimension.DimensionType;
|
||||
import net.minecraft.world.gen.ChunkGenerator;
|
||||
import net.minecraft.world.gen.OverworldGenSettings;
|
||||
import net.minecraft.world.level.LevelGeneratorType;
|
||||
import net.minecraftforge.api.distmarker.Dist;
|
||||
import net.minecraftforge.api.distmarker.OnlyIn;
|
||||
|
||||
import java.util.HashSet;
|
||||
import java.util.Set;
|
||||
|
||||
public class TerraWorld extends LevelGeneratorType {
|
||||
public static final int VERSION = 1;
|
||||
|
||||
private static final Set<WorldType> types = new HashSet<>();
|
||||
public static final TerraWorld TERRA = new TerraWorld("terraforged", TerraChunkGenerator::new);
|
||||
public static final TerraWorld TEST = new TerraWorld("terratest", TestChunkGenerator::new);
|
||||
|
||||
private final ChunkGeneratorFactory<?> factory;
|
||||
|
||||
public TerraWorld(String name, ChunkGeneratorFactory<?> factory) {
|
||||
super(name);
|
||||
this.factory = factory;
|
||||
setCustomOptions(true);
|
||||
TerraWorld.types.add(this);
|
||||
}
|
||||
|
||||
@Override
|
||||
public double getHorizon(World world) {
|
||||
return 0;
|
||||
}
|
||||
|
||||
@Override
|
||||
public float getCloudHeight() {
|
||||
return 260.0F;
|
||||
}
|
||||
|
||||
@Override
|
||||
public ChunkGenerator<?> createChunkGenerator(World world) {
|
||||
if (world.getDimension().getType() != DimensionType.OVERWORLD) {
|
||||
return world.getDimension().createChunkGenerator();
|
||||
}
|
||||
|
||||
Log.debug("Creating {} generator", world.getDimension().getType());
|
||||
|
||||
int version = SettingsHelper.getVersion(world.getWorldInfo());
|
||||
TerraSettings settings = SettingsHelper.getSettings(world);
|
||||
SettingsHelper.syncSettings(world.getWorldInfo(), settings, version);
|
||||
|
||||
Terrains terrains = Terrains.create(settings);
|
||||
|
||||
OverworldGenSettings genSettings = new TerraGenSettings(settings.structures);
|
||||
OverworldBiomeProviderSettings biomeSettings = new OverworldBiomeProviderSettings(world.getWorldInfo());
|
||||
biomeSettings.setGeneratorSettings(genSettings);
|
||||
world.getWorldInfo().setGeneratorOptions(NBTHelper.serializeCompact(settings));
|
||||
|
||||
TerraContext context = new TerraContext(world, terrains, settings);
|
||||
BiomeProvider biomeProvider = new BiomeProvider(context);
|
||||
|
||||
return getGeneratorFactory().create(context, biomeProvider, genSettings);
|
||||
}
|
||||
|
||||
@Override
|
||||
@OnlyIn(Dist.CLIENT)
|
||||
public void onCustomizeButton(Minecraft mc, CreateWorldScreen gui) {
|
||||
mc.displayGuiScreen(new SettingsScreen(gui));
|
||||
}
|
||||
|
||||
public ChunkGeneratorFactory<?> getGeneratorFactory() {
|
||||
return factory;
|
||||
}
|
||||
|
||||
public static void init() {
|
||||
Log.info("Registered world type(s)");
|
||||
}
|
||||
|
||||
public static boolean isTerraWorld(IWorld world) {
|
||||
if (world instanceof World) {
|
||||
return types.contains(((World) world).getWorldType());
|
||||
}
|
||||
return false;
|
||||
}
|
||||
}
|
@ -0,0 +1,93 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.biome;
|
||||
|
||||
import com.terraforged.api.biome.BiomeVariant;
|
||||
import net.minecraft.entity.EntityCategory;
|
||||
import net.minecraft.entity.EntityType;
|
||||
import net.minecraft.util.math.BlockPos;
|
||||
import net.minecraft.world.WorldView;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
import net.minecraft.world.biome.Biomes;
|
||||
import net.minecraft.world.biome.DefaultBiomeFeatures;
|
||||
import net.minecraft.world.gen.surfacebuilder.SurfaceBuilder;
|
||||
|
||||
public class ColdSteppe extends BiomeVariant {
|
||||
|
||||
public ColdSteppe() {
|
||||
super((new Settings()).configureSurfaceBuilder(SurfaceBuilder.GIANT_TREE_TAIGA, SurfaceBuilder.GRASS_CONFIG)
|
||||
.precipitation(Precipitation.SNOW).category(Biome.Category.TAIGA).depth(0.2F).scale(0.25F).temperature(0.2F).downfall(0.1F)
|
||||
.waterColor(4159204).waterFogColor(329011).parent(null));
|
||||
DefaultBiomeFeatures.addLandCarvers(this);
|
||||
DefaultBiomeFeatures.addDefaultStructures(this);
|
||||
// DefaultBiomeFeatures.addLakes(this);
|
||||
DefaultBiomeFeatures.addDungeons(this);
|
||||
DefaultBiomeFeatures.addLargeFerns(this);
|
||||
DefaultBiomeFeatures.addMineables(this);
|
||||
DefaultBiomeFeatures.addDefaultOres(this);
|
||||
DefaultBiomeFeatures.addDefaultDisks(this);
|
||||
// DefaultBiomeFeatures.addTaigaConifers(this);
|
||||
// DefaultBiomeFeatures.addDefaultFlowers(this);
|
||||
DefaultBiomeFeatures.addForestGrass(this);
|
||||
DefaultBiomeFeatures.addSavannaGrass(this);
|
||||
// DefaultBiomeFeatures.addMushrooms(this);
|
||||
// DefaultBiomeFeatures.addReedsAndPumpkins(this);
|
||||
// DefaultBiomeFeatures.addSprings(this);
|
||||
DefaultBiomeFeatures.addSweetBerryBushes(this);
|
||||
DefaultBiomeFeatures.addFrozenTopLayer(this);
|
||||
this.addSpawn(EntityCategory.CREATURE, new Biome.SpawnEntry(EntityType.SHEEP, 12, 4, 4));
|
||||
this.addSpawn(EntityCategory.CREATURE, new Biome.SpawnEntry(EntityType.PIG, 10, 4, 4));
|
||||
this.addSpawn(EntityCategory.CREATURE, new Biome.SpawnEntry(EntityType.CHICKEN, 10, 4, 4));
|
||||
this.addSpawn(EntityCategory.CREATURE, new Biome.SpawnEntry(EntityType.COW, 8, 4, 4));
|
||||
this.addSpawn(EntityCategory.CREATURE, new Biome.SpawnEntry(EntityType.WOLF, 8, 4, 4));
|
||||
this.addSpawn(EntityCategory.CREATURE, new Biome.SpawnEntry(EntityType.RABBIT, 4, 2, 3));
|
||||
this.addSpawn(EntityCategory.CREATURE, new Biome.SpawnEntry(EntityType.FOX, 8, 2, 4));
|
||||
this.addSpawn(EntityCategory.AMBIENT, new Biome.SpawnEntry(EntityType.BAT, 10, 8, 8));
|
||||
this.addSpawn(EntityCategory.MONSTER, new Biome.SpawnEntry(EntityType.SPIDER, 100, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new Biome.SpawnEntry(EntityType.ZOMBIE, 95, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new Biome.SpawnEntry(EntityType.ZOMBIE_VILLAGER, 5, 1, 1));
|
||||
this.addSpawn(EntityCategory.MONSTER, new Biome.SpawnEntry(EntityType.SKELETON, 100, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new Biome.SpawnEntry(EntityType.CREEPER, 100, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new Biome.SpawnEntry(EntityType.SLIME, 100, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new Biome.SpawnEntry(EntityType.ENDERMAN, 10, 1, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new Biome.SpawnEntry(EntityType.WITCH, 5, 1, 1));
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean canSetSnow(WorldView worldIn, BlockPos pos) {
|
||||
return false;
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean canSetIce(WorldView worldIn, BlockPos pos) {
|
||||
return false;
|
||||
}
|
||||
|
||||
@Override
|
||||
public Biome getBase() {
|
||||
return Biomes.TAIGA;
|
||||
}
|
||||
}
|
@ -0,0 +1,109 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.biome;
|
||||
|
||||
import com.terraforged.api.biome.BiomeVariant;
|
||||
import net.minecraft.entity.EntityCategory;
|
||||
import net.minecraft.entity.EntityType;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
import net.minecraft.world.biome.Biomes;
|
||||
import net.minecraft.world.biome.DefaultBiomeFeatures;
|
||||
import net.minecraft.world.gen.GenerationStep;
|
||||
import net.minecraft.world.gen.decorator.CountChanceDecoratorConfig;
|
||||
import net.minecraft.world.gen.decorator.CountDecoratorConfig;
|
||||
import net.minecraft.world.gen.decorator.Decorator;
|
||||
import net.minecraft.world.gen.feature.Feature;
|
||||
import net.minecraft.world.gen.surfacebuilder.SurfaceBuilder;
|
||||
|
||||
public class Marshland extends BiomeVariant {
|
||||
|
||||
public Marshland() {
|
||||
super((new Settings()).configureSurfaceBuilder(SurfaceBuilder.SWAMP, SurfaceBuilder.GRASS_CONFIG).precipitation(Precipitation.RAIN)
|
||||
.category(Category.SWAMP).depth(0.2F).scale(0.2F).temperature(0.8F).downfall(0.4F).waterColor(6388580).waterFogColor(2302743)
|
||||
.parent(null));
|
||||
DefaultBiomeFeatures.addLandCarvers(this);
|
||||
DefaultBiomeFeatures.addDefaultStructures(this);
|
||||
DefaultBiomeFeatures.addDungeons(this);
|
||||
DefaultBiomeFeatures.addMineables(this);
|
||||
DefaultBiomeFeatures.addDefaultOres(this);
|
||||
DefaultBiomeFeatures.addDefaultDisks(this);
|
||||
DefaultBiomeFeatures.addDefaultFlowers(this);
|
||||
DefaultBiomeFeatures.addSavannaTallGrass(this);
|
||||
DefaultBiomeFeatures.addSavannaTallGrass(this);
|
||||
DefaultBiomeFeatures.addSavannaTallGrass(this);
|
||||
DefaultBiomeFeatures.addSavannaTallGrass(this);
|
||||
DefaultBiomeFeatures.addForestGrass(this);
|
||||
DefaultBiomeFeatures.addSavannaGrass(this);
|
||||
DefaultBiomeFeatures.addDefaultVegetation(this);
|
||||
Marshland.addSwampVegetation(this);
|
||||
DefaultBiomeFeatures.addFrozenTopLayer(this);
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.SHEEP, 12, 4, 4));
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.PIG, 10, 4, 4));
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.CHICKEN, 10, 4, 4));
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.COW, 8, 4, 4));
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.WOLF, 8, 4, 4));
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.RABBIT, 4, 2, 3));
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.FOX, 8, 2, 4));
|
||||
this.addSpawn(EntityCategory.AMBIENT, new SpawnEntry(EntityType.BAT, 10, 8, 8));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.SPIDER, 100, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.ZOMBIE, 95, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.ZOMBIE_VILLAGER, 5, 1, 1));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.SKELETON, 100, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.CREEPER, 100, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.SLIME, 100, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.ENDERMAN, 10, 1, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.WITCH, 5, 1, 1));
|
||||
}
|
||||
|
||||
public int getGrassColor(double p_225528_1_, double p_225528_3_) {
|
||||
double d0 = FOLIAGE_NOISE.sample(p_225528_1_ * 0.0225D, p_225528_3_ * 0.0225D, false);
|
||||
return d0 < -0.1D ? 5011004 : 6975545;
|
||||
}
|
||||
|
||||
public int getFoliageColor() {
|
||||
return 6975545;
|
||||
}
|
||||
|
||||
@Override
|
||||
public Biome getBase() {
|
||||
return Biomes.SWAMP;
|
||||
}
|
||||
|
||||
private static void addSwampVegetation(Biome biome) {
|
||||
biome.addFeature(GenerationStep.Feature.VEGETAL_DECORATION, Feature.RANDOM_PATCH.configure(DefaultBiomeFeatures.LILY_PAD_CONFIG)
|
||||
.createDecoratedFeature(Decorator.COUNT_HEIGHTMAP_DOUBLE.configure(new CountDecoratorConfig(4))));
|
||||
biome.addFeature(GenerationStep.Feature.VEGETAL_DECORATION, Feature.RANDOM_PATCH.configure(DefaultBiomeFeatures.DEAD_BUSH_CONFIG)
|
||||
.createDecoratedFeature(Decorator.COUNT_HEIGHTMAP_DOUBLE.configure(new CountDecoratorConfig(1))));
|
||||
biome.addFeature(GenerationStep.Feature.VEGETAL_DECORATION, Feature.RANDOM_PATCH.configure(DefaultBiomeFeatures.LILY_PAD_CONFIG)
|
||||
.createDecoratedFeature(Decorator.COUNT_HEIGHTMAP_DOUBLE.configure(new CountDecoratorConfig(4))));
|
||||
biome.addFeature(GenerationStep.Feature.VEGETAL_DECORATION,
|
||||
Feature.RANDOM_PATCH.configure(DefaultBiomeFeatures.BROWN_MUSHROOM_CONFIG)
|
||||
.createDecoratedFeature(Decorator.COUNT_CHANCE_HEIGHTMAP.configure(new CountChanceDecoratorConfig(8, 0.25F))));
|
||||
biome.addFeature(GenerationStep.Feature.VEGETAL_DECORATION,
|
||||
Feature.RANDOM_PATCH.configure(DefaultBiomeFeatures.RED_MUSHROOM_CONFIG)
|
||||
.createDecoratedFeature(Decorator.COUNT_CHANCE_HEIGHTMAP_DOUBLE.configure(new CountChanceDecoratorConfig(8, 0.125F))));
|
||||
}
|
||||
}
|
@ -0,0 +1,51 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.biome;
|
||||
|
||||
import com.terraforged.api.biome.BiomeVariant;
|
||||
import net.minecraft.util.Identifier;
|
||||
import net.minecraft.util.registry.Registry;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
|
||||
import java.util.ArrayList;
|
||||
|
||||
public class ModBiomes {
|
||||
|
||||
private static final ArrayList<BiomeVariant> biomes = new ArrayList<>();
|
||||
|
||||
public static final Biome COLD_STEPPE = register("cold_steppe", new ColdSteppe());
|
||||
public static final Biome SAVANNA_SCRUB = register("savanna_scrub", new SavannaScrub());
|
||||
public static final Biome SHATTERED_SAVANNA_SCRUB = register("shattered_savanna_scrub", new ShatteredSavannaScrub());
|
||||
public static final Biome SNOWY_TAIGA_SCRUB = register("snowy_taiga_scrub", new SnowyTaigaScrub());
|
||||
public static final Biome STEPPE = register("steppe", new Steppe());
|
||||
public static final Biome TAIGA_SCRUB = register("taiga_scrub", new TaigaScrub());
|
||||
public static final Biome WARM_BEACH = register("warm_beach", new WarmBeach());
|
||||
public static final Biome MARSHLAND = register("marshland", new Marshland());
|
||||
|
||||
private static Biome register(String name, BiomeVariant biome) {
|
||||
return Registry.register(Registry.BIOME, new Identifier("terraforged", name), biome);
|
||||
}
|
||||
}
|
@ -0,0 +1,114 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.biome;
|
||||
|
||||
import com.terraforged.api.biome.BiomeVariant;
|
||||
import net.minecraft.block.BlockState;
|
||||
import net.minecraft.block.Blocks;
|
||||
import net.minecraft.entity.EntityCategory;
|
||||
import net.minecraft.entity.EntityType;
|
||||
import net.minecraft.util.math.BlockPos;
|
||||
import net.minecraft.world.Heightmap;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
import net.minecraft.world.biome.Biomes;
|
||||
import net.minecraft.world.biome.DefaultBiomeFeatures;
|
||||
import net.minecraft.world.chunk.Chunk;
|
||||
import net.minecraft.world.gen.surfacebuilder.DefaultSurfaceBuilder;
|
||||
import net.minecraft.world.gen.surfacebuilder.SurfaceBuilder;
|
||||
import net.minecraft.world.gen.surfacebuilder.TernarySurfaceConfig;
|
||||
|
||||
import java.util.Random;
|
||||
|
||||
public class SavannaScrub extends BiomeVariant {
|
||||
|
||||
public SavannaScrub() {
|
||||
super((new Settings()).configureSurfaceBuilder(new Builder(), SurfaceBuilder.GRASS_CONFIG).precipitation(Precipitation.NONE)
|
||||
.category(Biome.Category.SAVANNA).depth(0.125F).scale(0.05F).temperature(1.2F).downfall(0.0F).waterColor(4159204)
|
||||
.waterFogColor(329011).parent(null));
|
||||
DefaultBiomeFeatures.addLandCarvers(this);
|
||||
DefaultBiomeFeatures.addDefaultStructures(this);
|
||||
// DefaultBiomeFeatures.addLakes(this);
|
||||
DefaultBiomeFeatures.addDungeons(this);
|
||||
DefaultBiomeFeatures.addSavannaTallGrass(this);
|
||||
DefaultBiomeFeatures.addMineables(this);
|
||||
DefaultBiomeFeatures.addDefaultOres(this);
|
||||
DefaultBiomeFeatures.addDefaultDisks(this);
|
||||
// DefaultBiomeFeatures.addSavannaTrees(this);
|
||||
DefaultBiomeFeatures.addExtraDefaultFlowers(this);
|
||||
|
||||
// func_222339_L - add grasses - add this a few times since there are no trees
|
||||
DefaultBiomeFeatures.addForestGrass(this);
|
||||
DefaultBiomeFeatures.addSavannaGrass(this);
|
||||
|
||||
DefaultBiomeFeatures.addDefaultMushrooms(this);
|
||||
DefaultBiomeFeatures.addDefaultVegetation(this);
|
||||
// DefaultBiomeFeatures.addSprings(this);
|
||||
// DefaultBiomeFeatures.addFreezeTopLayer(this);
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.SHEEP, 12, 4, 4));
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.PIG, 10, 4, 4));
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.CHICKEN, 10, 4, 4));
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.COW, 8, 4, 4));
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.HORSE, 1, 2, 6));
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.DONKEY, 1, 1, 1));
|
||||
this.addSpawn(EntityCategory.AMBIENT, new SpawnEntry(EntityType.BAT, 10, 8, 8));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.SPIDER, 100, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.ZOMBIE, 95, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.ZOMBIE_VILLAGER, 5, 1, 1));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.SKELETON, 100, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.CREEPER, 100, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.SLIME, 100, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.ENDERMAN, 10, 1, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.WITCH, 5, 1, 1));
|
||||
}
|
||||
|
||||
@Override
|
||||
public Biome getBase() {
|
||||
return Biomes.SAVANNA;
|
||||
}
|
||||
|
||||
private static class Builder extends DefaultSurfaceBuilder {
|
||||
|
||||
private Builder() {
|
||||
super(TernarySurfaceConfig::deserialize);
|
||||
}
|
||||
|
||||
@Override
|
||||
public void generate(Random random, Chunk chunkIn, Biome biomeIn, int x, int z, int startHeight, double noise, BlockState defaultBlock,
|
||||
BlockState defaultFluid, int seaLevel, long seed, TernarySurfaceConfig config) {
|
||||
super.generate(random, chunkIn, biomeIn, x, z, startHeight, noise, defaultBlock, defaultFluid, seaLevel, seed, config);
|
||||
BlockPos.Mutable pos = new BlockPos.Mutable();
|
||||
if (random.nextInt(5) > 0) {
|
||||
int dx = x & 15;
|
||||
int dz = z & 15;
|
||||
int y = chunkIn.sampleHeightmap(Heightmap.Type.MOTION_BLOCKING, dx, dz);
|
||||
if (y > seaLevel) {
|
||||
pos.set(dx, y, dz);
|
||||
//chunkIn.setBlockState(pos, Blocks.SAND.getDefaultState(), false); //todo need tweak
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
@ -0,0 +1,118 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.biome;
|
||||
|
||||
import com.terraforged.api.biome.BiomeVariant;
|
||||
import net.minecraft.block.BlockState;
|
||||
import net.minecraft.entity.EntityCategory;
|
||||
import net.minecraft.entity.EntityType;
|
||||
import net.minecraft.util.math.BlockPos;
|
||||
import net.minecraft.world.Heightmap;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
import net.minecraft.world.biome.Biomes;
|
||||
import net.minecraft.world.biome.DefaultBiomeFeatures;
|
||||
import net.minecraft.world.chunk.Chunk;
|
||||
import net.minecraft.world.gen.surfacebuilder.DefaultSurfaceBuilder;
|
||||
import net.minecraft.world.gen.surfacebuilder.SurfaceBuilder;
|
||||
import net.minecraft.world.gen.surfacebuilder.TernarySurfaceConfig;
|
||||
|
||||
import java.util.Random;
|
||||
|
||||
public class ShatteredSavannaScrub extends BiomeVariant {
|
||||
|
||||
public ShatteredSavannaScrub() {
|
||||
super((new Settings()).configureSurfaceBuilder(new Builder(), SurfaceBuilder.GRASS_CONFIG).precipitation(Precipitation.NONE)
|
||||
.category(Biome.Category.SAVANNA).depth(0.3625F).scale(1.225F).temperature(1.1F).downfall(0.0F).waterColor(4159204)
|
||||
.waterFogColor(329011).parent("terraforged:savanna_scrub"));
|
||||
DefaultBiomeFeatures.addLandCarvers(this);
|
||||
DefaultBiomeFeatures.addDefaultStructures(this);
|
||||
// DefaultBiomeFeatures.addLakes(this);
|
||||
DefaultBiomeFeatures.addDungeons(this);
|
||||
DefaultBiomeFeatures.addMineables(this);
|
||||
DefaultBiomeFeatures.addDefaultOres(this);
|
||||
DefaultBiomeFeatures.addDefaultDisks(this);
|
||||
// DefaultBiomeFeatures.addShatteredSavannaTrees(this);
|
||||
DefaultBiomeFeatures.addDefaultFlowers(this);
|
||||
|
||||
// func_222314_K - addGrasses - add this a few times since there are no trees
|
||||
DefaultBiomeFeatures.addForestGrass(this);
|
||||
DefaultBiomeFeatures.addSavannaGrass(this);
|
||||
|
||||
DefaultBiomeFeatures.addDefaultMushrooms(this);
|
||||
DefaultBiomeFeatures.addDefaultVegetation(this);
|
||||
// DefaultBiomeFeatures.addSprings(this);
|
||||
DefaultBiomeFeatures.addFrozenTopLayer(this);
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.SHEEP, 12, 4, 4));
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.PIG, 10, 4, 4));
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.CHICKEN, 10, 4, 4));
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.COW, 8, 4, 4));
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.HORSE, 1, 2, 6));
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.DONKEY, 1, 1, 1));
|
||||
this.addSpawn(EntityCategory.AMBIENT, new SpawnEntry(EntityType.BAT, 10, 8, 8));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.SPIDER, 100, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.ZOMBIE, 95, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.ZOMBIE_VILLAGER, 5, 1, 1));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.SKELETON, 100, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.CREEPER, 100, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.SLIME, 100, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.ENDERMAN, 10, 1, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.WITCH, 5, 1, 1));
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean hasParent() {
|
||||
return true;
|
||||
}
|
||||
|
||||
@Override
|
||||
public Biome getBase() {
|
||||
return Biomes.SHATTERED_SAVANNA;
|
||||
}
|
||||
|
||||
private static class Builder extends DefaultSurfaceBuilder {
|
||||
|
||||
private Builder() {
|
||||
super(TernarySurfaceConfig::deserialize);
|
||||
}
|
||||
|
||||
@Override
|
||||
public void generate(Random random, Chunk chunkIn, Biome biomeIn, int x, int z, int startHeight, double noise, BlockState defaultBlock,
|
||||
BlockState defaultFluid, int seaLevel, long seed, TernarySurfaceConfig config) {
|
||||
SurfaceBuilder.SHATTERED_SAVANNA
|
||||
.generate(random, chunkIn, biomeIn, x, z, startHeight, noise, defaultBlock, defaultFluid, seaLevel, seed, config);
|
||||
BlockPos.Mutable pos = new BlockPos.Mutable();
|
||||
if (random.nextInt(5) > 0) {
|
||||
int dx = x & 15;
|
||||
int dz = z & 15;
|
||||
int y = chunkIn.sampleHeightmap(Heightmap.Type.MOTION_BLOCKING, dx, dz);
|
||||
if (y > seaLevel) {
|
||||
pos.set(dx, y, dz);
|
||||
// chunkIn.setBlockState(pos, Blocks.SAND.getDefaultState(), false);
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
@ -0,0 +1,81 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.biome;
|
||||
|
||||
import com.terraforged.api.biome.BiomeVariant;
|
||||
import net.minecraft.entity.EntityCategory;
|
||||
import net.minecraft.entity.EntityType;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
import net.minecraft.world.biome.Biomes;
|
||||
import net.minecraft.world.biome.DefaultBiomeFeatures;
|
||||
import net.minecraft.world.gen.surfacebuilder.SurfaceBuilder;
|
||||
|
||||
public class SnowyTaigaScrub extends BiomeVariant {
|
||||
|
||||
public SnowyTaigaScrub() {
|
||||
super(new Settings().configureSurfaceBuilder(SurfaceBuilder.DEFAULT, SurfaceBuilder.GRASS_CONFIG).precipitation(Precipitation.SNOW)
|
||||
.category(Category.TAIGA).depth(0.2F).scale(0.2F).temperature(-0.5F).downfall(0.4F).waterColor(4020182).waterFogColor(329011)
|
||||
.parent(null));
|
||||
DefaultBiomeFeatures.addLandCarvers(this);
|
||||
DefaultBiomeFeatures.addDefaultStructures(this);
|
||||
// DefaultBiomeFeatures.addLakes(this);
|
||||
DefaultBiomeFeatures.addDungeons(this);
|
||||
DefaultBiomeFeatures.addLargeFerns(this);
|
||||
DefaultBiomeFeatures.addMineables(this);
|
||||
DefaultBiomeFeatures.addDefaultOres(this);
|
||||
DefaultBiomeFeatures.addDefaultDisks(this);
|
||||
// DefaultBiomeFeatures.addTaigaConifers(this);
|
||||
DefaultBiomeFeatures.addDefaultFlowers(this);
|
||||
DefaultBiomeFeatures.addTaigaGrass(this);
|
||||
DefaultBiomeFeatures.addForestGrass(this);
|
||||
DefaultBiomeFeatures.addDefaultMushrooms(this);
|
||||
DefaultBiomeFeatures.addDefaultVegetation(this);
|
||||
// DefaultBiomeFeatures.addSprings(this);
|
||||
DefaultBiomeFeatures.addSweetBerryBushesSnowy(this);
|
||||
DefaultBiomeFeatures.addFrozenTopLayer(this);
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.SHEEP, 12, 4, 4));
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.PIG, 10, 4, 4));
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.CHICKEN, 10, 4, 4));
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.COW, 8, 4, 4));
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.WOLF, 8, 4, 4));
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.RABBIT, 4, 2, 3));
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.FOX, 8, 2, 4));
|
||||
this.addSpawn(EntityCategory.AMBIENT, new SpawnEntry(EntityType.BAT, 10, 8, 8));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.SPIDER, 100, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.ZOMBIE, 95, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.ZOMBIE_VILLAGER, 5, 1, 1));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.SKELETON, 100, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.CREEPER, 100, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.SLIME, 100, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.ENDERMAN, 10, 1, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.WITCH, 5, 1, 1));
|
||||
}
|
||||
|
||||
@Override
|
||||
public Biome getBase() {
|
||||
return Biomes.SNOWY_TAIGA;
|
||||
}
|
||||
}
|
@ -0,0 +1,94 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.biome;
|
||||
|
||||
import com.terraforged.api.biome.BiomeVariant;
|
||||
import net.minecraft.entity.EntityCategory;
|
||||
import net.minecraft.entity.EntityType;
|
||||
import net.minecraft.util.math.BlockPos;
|
||||
import net.minecraft.world.WorldView;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
import net.minecraft.world.biome.Biomes;
|
||||
import net.minecraft.world.biome.DefaultBiomeFeatures;
|
||||
import net.minecraft.world.gen.surfacebuilder.SurfaceBuilder;
|
||||
|
||||
public class Steppe extends BiomeVariant {
|
||||
|
||||
protected Steppe() {
|
||||
super((new Settings()).configureSurfaceBuilder(SurfaceBuilder.GIANT_TREE_TAIGA, SurfaceBuilder.GRASS_CONFIG).precipitation(Precipitation.SNOW)
|
||||
.category(Category.SAVANNA).depth(0.2F).scale(0.2F).temperature(0.7F).downfall(0.1F).waterColor(4159204).waterFogColor(329011)
|
||||
.parent(null));
|
||||
DefaultBiomeFeatures.addLandCarvers(this);
|
||||
DefaultBiomeFeatures.addDefaultStructures(this);
|
||||
// DefaultBiomeFeatures.addLakes(this);
|
||||
DefaultBiomeFeatures.addDungeons(this);
|
||||
// DefaultBiomeFeatures.addTaigaRocks(this);
|
||||
// DefaultBiomeFeatures.addTaigaLargeFerns(this);
|
||||
DefaultBiomeFeatures.addMineables(this);
|
||||
DefaultBiomeFeatures.addDefaultOres(this);
|
||||
DefaultBiomeFeatures.addDefaultDisks(this);
|
||||
// extra grasses as no trees/ferns
|
||||
DefaultBiomeFeatures.addForestGrass(this);
|
||||
DefaultBiomeFeatures.addSavannaGrass(this);
|
||||
// DefaultBiomeFeatures.func_222285_H(this);
|
||||
// DefaultBiomeFeatures.addDefaultFlowers(this);
|
||||
// DefaultBiomeFeatures.addMushrooms(this);
|
||||
// DefaultBiomeFeatures.addReedsAndPumpkins(this);
|
||||
// DefaultBiomeFeatures.addSprings(this);
|
||||
DefaultBiomeFeatures.addFrozenTopLayer(this);
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.SHEEP, 12, 4, 4));
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.PIG, 10, 4, 4));
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.CHICKEN, 10, 4, 4));
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.COW, 8, 4, 4));
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.WOLF, 8, 4, 4));
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.RABBIT, 4, 2, 3));
|
||||
this.addSpawn(EntityCategory.AMBIENT, new SpawnEntry(EntityType.BAT, 10, 8, 8));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.SPIDER, 100, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.ZOMBIE, 100, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.SKELETON, 100, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.ZOMBIE_VILLAGER, 25, 1, 1));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.CREEPER, 100, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.SLIME, 100, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.ENDERMAN, 10, 1, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.WITCH, 5, 1, 1));
|
||||
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean canSetIce(WorldView world, BlockPos blockPos) {
|
||||
return false;
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean canSetSnow(WorldView worldView, BlockPos blockPos) {
|
||||
return false;
|
||||
}
|
||||
|
||||
@Override
|
||||
public Biome getBase() {
|
||||
return Biomes.SAVANNA;
|
||||
}
|
||||
}
|
@ -0,0 +1,85 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.biome;
|
||||
|
||||
import com.terraforged.api.biome.BiomeVariant;
|
||||
import net.minecraft.entity.EntityCategory;
|
||||
import net.minecraft.entity.EntityType;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
import net.minecraft.world.biome.Biomes;
|
||||
import net.minecraft.world.biome.DefaultBiomeFeatures;
|
||||
import net.minecraft.world.gen.surfacebuilder.SurfaceBuilder;
|
||||
|
||||
public class TaigaScrub extends BiomeVariant {
|
||||
|
||||
public TaigaScrub() {
|
||||
super((new Settings()).configureSurfaceBuilder(SurfaceBuilder.DEFAULT, SurfaceBuilder.GRASS_CONFIG).precipitation(Precipitation.RAIN)
|
||||
.category(Category.TAIGA).depth(0.2F).scale(0.2F).temperature(0.25F).downfall(0.8F).waterColor(4159204).waterFogColor(329011)
|
||||
.parent(null));
|
||||
DefaultBiomeFeatures.addLandCarvers(this);
|
||||
DefaultBiomeFeatures.addDefaultStructures(this);
|
||||
// DefaultBiomeFeatures.addLakes(this);
|
||||
DefaultBiomeFeatures.addDungeons(this);
|
||||
DefaultBiomeFeatures.addLargeFerns(this);
|
||||
DefaultBiomeFeatures.addMineables(this);
|
||||
DefaultBiomeFeatures.addDefaultOres(this);
|
||||
DefaultBiomeFeatures.addDefaultDisks(this);
|
||||
// DefaultBiomeFeatures.addTaigaConifers(this);
|
||||
DefaultBiomeFeatures.addDefaultFlowers(this);
|
||||
DefaultBiomeFeatures.addForestGrass(this);
|
||||
DefaultBiomeFeatures.addSavannaGrass(this);
|
||||
|
||||
// extra grass since there are no trees
|
||||
DefaultBiomeFeatures.addForestGrass(this);
|
||||
DefaultBiomeFeatures.addForestGrass(this);
|
||||
|
||||
DefaultBiomeFeatures.addDefaultVegetation(this);
|
||||
// DefaultBiomeFeatures.addSprings(this);
|
||||
DefaultBiomeFeatures.addSweetBerryBushes(this);
|
||||
DefaultBiomeFeatures.addFrozenTopLayer(this);
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.SHEEP, 12, 4, 4));
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.PIG, 10, 4, 4));
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.CHICKEN, 10, 4, 4));
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.COW, 8, 4, 4));
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.WOLF, 8, 4, 4));
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.RABBIT, 4, 2, 3));
|
||||
this.addSpawn(EntityCategory.CREATURE, new SpawnEntry(EntityType.FOX, 8, 2, 4));
|
||||
this.addSpawn(EntityCategory.AMBIENT, new SpawnEntry(EntityType.BAT, 10, 8, 8));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.SPIDER, 100, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.ZOMBIE, 95, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.ZOMBIE_VILLAGER, 5, 1, 1));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.SKELETON, 100, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.CREEPER, 100, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.SLIME, 100, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.ENDERMAN, 10, 1, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new SpawnEntry(EntityType.WITCH, 5, 1, 1));
|
||||
}
|
||||
|
||||
@Override
|
||||
public Biome getBase() {
|
||||
return Biomes.TAIGA;
|
||||
}
|
||||
}
|
@ -0,0 +1,79 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.biome;
|
||||
|
||||
import com.terraforged.api.biome.BiomeVariant;
|
||||
import net.minecraft.entity.EntityCategory;
|
||||
import net.minecraft.entity.EntityType;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
import net.minecraft.world.biome.Biomes;
|
||||
import net.minecraft.world.biome.DefaultBiomeFeatures;
|
||||
import net.minecraft.world.gen.feature.BuriedTreasureFeatureConfig;
|
||||
import net.minecraft.world.gen.feature.Feature;
|
||||
import net.minecraft.world.gen.feature.MineshaftFeature;
|
||||
import net.minecraft.world.gen.feature.MineshaftFeatureConfig;
|
||||
import net.minecraft.world.gen.surfacebuilder.SurfaceBuilder;
|
||||
|
||||
public class WarmBeach extends BiomeVariant {
|
||||
|
||||
public WarmBeach() {
|
||||
super((new Settings()).configureSurfaceBuilder(SurfaceBuilder.DEFAULT, SurfaceBuilder.SAND_CONFIG).precipitation(Precipitation.RAIN)
|
||||
.category(Category.BEACH).depth(-0.5F).scale(0.1F).temperature(1.5F).downfall(0.5F).waterColor(4445678).waterFogColor(270131)
|
||||
.parent(null));
|
||||
this.addStructureFeature(Feature.MINESHAFT.configure(new MineshaftFeatureConfig(0.004D, MineshaftFeature.Type.NORMAL)));
|
||||
this.addStructureFeature(Feature.BURIED_TREASURE.configure(new BuriedTreasureFeatureConfig(0.01F)));
|
||||
DefaultBiomeFeatures.addLandCarvers(this);
|
||||
DefaultBiomeFeatures.addDefaultStructures(this);
|
||||
DefaultBiomeFeatures.addDungeons(this);
|
||||
DefaultBiomeFeatures.addMineables(this);
|
||||
DefaultBiomeFeatures.addDefaultOres(this);
|
||||
DefaultBiomeFeatures.addDefaultFlowers(this);
|
||||
DefaultBiomeFeatures.addDefaultGrass(this);
|
||||
DefaultBiomeFeatures.addDefaultMushrooms(this);
|
||||
DefaultBiomeFeatures.addDefaultVegetation(this);
|
||||
DefaultBiomeFeatures.addFrozenTopLayer(this);
|
||||
this.addSpawn(EntityCategory.CREATURE, new Biome.SpawnEntry(EntityType.TURTLE, 5, 2, 5));
|
||||
this.addSpawn(EntityCategory.AMBIENT, new Biome.SpawnEntry(EntityType.BAT, 10, 8, 8));
|
||||
this.addSpawn(EntityCategory.MONSTER, new Biome.SpawnEntry(EntityType.SPIDER, 100, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new Biome.SpawnEntry(EntityType.ZOMBIE, 95, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new Biome.SpawnEntry(EntityType.ZOMBIE_VILLAGER, 5, 1, 1));
|
||||
this.addSpawn(EntityCategory.MONSTER, new Biome.SpawnEntry(EntityType.SKELETON, 100, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new Biome.SpawnEntry(EntityType.CREEPER, 100, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new Biome.SpawnEntry(EntityType.SLIME, 100, 4, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new Biome.SpawnEntry(EntityType.ENDERMAN, 10, 1, 4));
|
||||
this.addSpawn(EntityCategory.MONSTER, new Biome.SpawnEntry(EntityType.WITCH, 5, 1, 1));
|
||||
}
|
||||
|
||||
@Override
|
||||
public Biome.TemperatureGroup getTemperatureGroup() {
|
||||
return TemperatureGroup.WARM;
|
||||
}
|
||||
|
||||
@Override
|
||||
public Biome getBase() {
|
||||
return Biomes.WARM_OCEAN;
|
||||
}
|
||||
}
|
@ -0,0 +1,220 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.biome.map;
|
||||
|
||||
import com.google.common.collect.Sets;
|
||||
import com.google.gson.JsonArray;
|
||||
import com.google.gson.JsonObject;
|
||||
import com.terraforged.core.world.biome.BiomeType;
|
||||
import com.terraforged.mod.biome.ModBiomes;
|
||||
import com.terraforged.mod.biome.provider.BiomeHelper;
|
||||
import me.dags.noise.util.NoiseUtil;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
import net.minecraft.world.biome.Biomes;
|
||||
|
||||
import java.util.Collections;
|
||||
import java.util.HashSet;
|
||||
import java.util.Set;
|
||||
|
||||
public abstract class AbstractBiomeMap implements BiomeMap {
|
||||
|
||||
private final Biome[][] beach;
|
||||
private final Biome[][] river;
|
||||
private final Biome[][] wetland;
|
||||
private final Biome[][] ocean;
|
||||
private final Biome[][] deepOcean;
|
||||
|
||||
protected final DefaultBiome defaultLand = this::defaultBiome;
|
||||
protected final DefaultBiome defaultBeach = this::defaultBeach;
|
||||
protected final DefaultBiome defaultRiver = this::defaultRiver;
|
||||
protected final DefaultBiome defaultWetland = this::defaultWetland;
|
||||
protected final DefaultBiome defaultOcean = this::defaultOcean;
|
||||
protected final DefaultBiome defaultDeepOcean = this::defaultDeepOcean;
|
||||
|
||||
protected AbstractBiomeMap(BiomeMapBuilder builder) {
|
||||
river = builder.rivers();
|
||||
beach = builder.beaches();
|
||||
ocean = builder.oceans();
|
||||
wetland = builder.wetlands();
|
||||
deepOcean = builder.deepOceans();
|
||||
}
|
||||
|
||||
@Override
|
||||
public Biome getBeach(float temperature, float moisture, float shape) {
|
||||
return get(beach, getCategory(temperature), shape, temperature, defaultBeach);
|
||||
}
|
||||
|
||||
@Override
|
||||
public Biome getRiver(float temperature, float moisture, float shape) {
|
||||
return get(river, getCategory(temperature), shape, temperature, defaultRiver);
|
||||
}
|
||||
|
||||
@Override
|
||||
public Biome getWetland(float temperature, float moisture, float shape) {
|
||||
return get(wetland, getCategory(temperature), shape, temperature, defaultWetland);
|
||||
}
|
||||
|
||||
@Override
|
||||
public Biome getOcean(float temperature, float moisture, float shape) {
|
||||
return get(ocean, getCategory(temperature), shape, temperature, defaultOcean);
|
||||
}
|
||||
|
||||
@Override
|
||||
public Biome getDeepOcean(float temperature, float moisture, float shape) {
|
||||
return get(deepOcean, getCategory(temperature), shape, temperature, defaultDeepOcean);
|
||||
}
|
||||
|
||||
@Override
|
||||
public Set<Biome> getOceanBiomes(Biome.TemperatureGroup temp) {
|
||||
return Sets.newHashSet(ocean[temp.ordinal() - 1]);
|
||||
}
|
||||
|
||||
@Override
|
||||
public Set<Biome> getDeepOceanBiomes(Biome.TemperatureGroup temp) {
|
||||
return Sets.newHashSet(deepOcean[temp.ordinal() - 1]);
|
||||
}
|
||||
|
||||
@Override
|
||||
public Set<Biome> getRivers(Biome.TemperatureGroup temp) {
|
||||
return Sets.newHashSet(river[temp.ordinal() - 1]);
|
||||
}
|
||||
|
||||
@Override
|
||||
public JsonObject toJson() {
|
||||
JsonObject root = new JsonObject();
|
||||
root.add("rivers", collect(river));
|
||||
root.add("wetland", collect(wetland));
|
||||
root.add("beaches", collect(beach));
|
||||
root.add("oceans", collect(ocean));
|
||||
root.add("deepOceans", collect(deepOcean));
|
||||
return root;
|
||||
}
|
||||
|
||||
private JsonObject collect(Biome[][] biomes) {
|
||||
JsonObject root = new JsonObject();
|
||||
for (Biome.TemperatureGroup temp : Biome.TemperatureGroup.values()) {
|
||||
if (temp == Biome.TemperatureGroup.OCEAN) {
|
||||
continue;
|
||||
}
|
||||
JsonArray array = new JsonArray();
|
||||
Biome[] group = biomes[temp.ordinal() - 1];
|
||||
if (group != null) {
|
||||
Set<Biome> set = new HashSet<>();
|
||||
Collections.addAll(set, group);
|
||||
set.stream().map(BiomeHelper::getId).sorted().forEach(array::add);
|
||||
}
|
||||
root.add(temp.name(), array);
|
||||
}
|
||||
return root;
|
||||
}
|
||||
|
||||
|
||||
protected Biome.TemperatureGroup getCategory(float value) {
|
||||
if (value < 0.25) {
|
||||
return Biome.TemperatureGroup.COLD;
|
||||
}
|
||||
if (value > 0.75) {
|
||||
return Biome.TemperatureGroup.WARM;
|
||||
}
|
||||
return Biome.TemperatureGroup.MEDIUM;
|
||||
}
|
||||
|
||||
protected Biome defaultBeach(float temperature) {
|
||||
if (temperature < 0.25) {
|
||||
return Biomes.SNOWY_BEACH;
|
||||
}
|
||||
if (temperature > 0.75) {
|
||||
return ModBiomes.WARM_BEACH;
|
||||
}
|
||||
return Biomes.BEACH;
|
||||
}
|
||||
|
||||
protected Biome defaultRiver(float temperature) {
|
||||
if (temperature < 0.15) {
|
||||
return Biomes.FROZEN_RIVER;
|
||||
}
|
||||
return Biomes.RIVER;
|
||||
}
|
||||
|
||||
protected Biome defaultWetland(float temperature) {
|
||||
if (temperature < 0.15) {
|
||||
return ModBiomes.TAIGA_SCRUB;
|
||||
}
|
||||
return ModBiomes.MARSHLAND;
|
||||
}
|
||||
|
||||
protected Biome defaultOcean(float temperature) {
|
||||
if (temperature < 0.3) {
|
||||
return Biomes.FROZEN_OCEAN;
|
||||
}
|
||||
if (temperature > 0.7) {
|
||||
return Biomes.WARM_OCEAN;
|
||||
}
|
||||
return Biomes.OCEAN;
|
||||
}
|
||||
|
||||
protected Biome defaultDeepOcean(float temperature) {
|
||||
if (temperature < 0.3) {
|
||||
return Biomes.DEEP_FROZEN_OCEAN;
|
||||
}
|
||||
if (temperature > 0.7) {
|
||||
return Biomes.DEEP_WARM_OCEAN;
|
||||
}
|
||||
return Biomes.DEEP_OCEAN;
|
||||
}
|
||||
|
||||
protected Biome defaultBiome(float temperature) {
|
||||
if (temperature < 0.3) {
|
||||
return ModBiomes.TAIGA_SCRUB;
|
||||
}
|
||||
if (temperature > 0.7) {
|
||||
return ModBiomes.SAVANNA_SCRUB;
|
||||
}
|
||||
return Biomes.PLAINS;
|
||||
}
|
||||
|
||||
protected Biome get(Biome[][] group, Biome.TemperatureGroup category, float shape, float temp, DefaultBiome def) {
|
||||
return get(group, category.ordinal() - 1, shape, temp, def);
|
||||
}
|
||||
|
||||
protected Biome get(Biome[][] group, BiomeType type, float shape, float temp, DefaultBiome def) {
|
||||
return get(group, type.ordinal(), shape, temp, def);
|
||||
}
|
||||
|
||||
protected Biome get(Biome[][] group, int ordinal, float shape, float temp, DefaultBiome def) {
|
||||
if (ordinal >= group.length) {
|
||||
return def.getDefaultBiome(temp);
|
||||
}
|
||||
|
||||
Biome[] biomes = group[ordinal];
|
||||
if (biomes == null || biomes.length == 0) {
|
||||
return def.getDefaultBiome(temp);
|
||||
}
|
||||
|
||||
int index = NoiseUtil.round((biomes.length - 1) * shape);
|
||||
return biomes[index];
|
||||
}
|
||||
}
|
@ -0,0 +1,82 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.biome.map;
|
||||
|
||||
import com.google.common.collect.Sets;
|
||||
import com.google.gson.JsonArray;
|
||||
import com.google.gson.JsonObject;
|
||||
import com.terraforged.core.world.biome.BiomeType;
|
||||
import com.terraforged.mod.biome.provider.BiomeHelper;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
|
||||
import java.util.Arrays;
|
||||
import java.util.Collections;
|
||||
import java.util.List;
|
||||
import java.util.Set;
|
||||
|
||||
public class BasicBiomeMap extends AbstractBiomeMap {
|
||||
|
||||
private final Biome[][] biomeTypes;
|
||||
|
||||
public BasicBiomeMap(BiomeMapBuilder builder) {
|
||||
super(builder);
|
||||
biomeTypes = builder.biomeList();
|
||||
}
|
||||
|
||||
@Override
|
||||
public List<Biome> getAllBiomes(BiomeType type) {
|
||||
if (type.ordinal() >= biomeTypes.length) {
|
||||
return Collections.emptyList();
|
||||
}
|
||||
return Arrays.asList(biomeTypes[type.ordinal()]);
|
||||
}
|
||||
|
||||
@Override
|
||||
public Set<Biome> getBiomes(BiomeType type) {
|
||||
if (type.ordinal() >= biomeTypes.length) {
|
||||
return Collections.emptySet();
|
||||
}
|
||||
return Sets.newHashSet(biomeTypes[type.ordinal()]);
|
||||
}
|
||||
|
||||
@Override
|
||||
public Biome getBiome(BiomeType type, float temperature, float moisture, float shape) {
|
||||
return get(biomeTypes, type, shape, temperature, defaultLand);
|
||||
}
|
||||
|
||||
@Override
|
||||
public JsonObject toJson() {
|
||||
JsonObject groups = new JsonObject();
|
||||
for (BiomeType type : BiomeType.values()) {
|
||||
JsonArray group = new JsonArray();
|
||||
getBiomes(type).stream().map(BiomeHelper::getId).sorted().forEach(group::add);
|
||||
groups.add(type.name(), group);
|
||||
}
|
||||
JsonObject root = super.toJson();
|
||||
root.add("biomes", groups);
|
||||
return root;
|
||||
}
|
||||
}
|
@ -0,0 +1,38 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.biome.map;
|
||||
|
||||
import com.terraforged.core.util.grid.FixedGrid;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
|
||||
public class BiomeGroup {
|
||||
|
||||
public final FixedGrid<Biome> biomes;
|
||||
|
||||
public BiomeGroup(FixedGrid<Biome> biomes) {
|
||||
this.biomes = biomes;
|
||||
}
|
||||
}
|
@ -0,0 +1,81 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.biome.map;
|
||||
|
||||
import com.google.gson.JsonElement;
|
||||
import com.terraforged.core.cell.Cell;
|
||||
import com.terraforged.core.world.biome.BiomeType;
|
||||
import com.terraforged.core.world.terrain.Terrain;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
|
||||
import java.util.List;
|
||||
import java.util.Set;
|
||||
|
||||
public interface BiomeMap {
|
||||
|
||||
Biome getBeach(float temperature, float moisture, float shape);
|
||||
|
||||
Biome getRiver(float temperature, float moisture, float shape);
|
||||
|
||||
Biome getWetland(float temperature, float moisture, float shape);
|
||||
|
||||
Biome getOcean(float temperature, float moisture, float shape);
|
||||
|
||||
Biome getDeepOcean(float temperature, float moisture, float shape);
|
||||
|
||||
Biome getBiome(BiomeType type, float temperature, float moisture, float shape);
|
||||
|
||||
default Biome getBiome(Cell<Terrain> cell) {
|
||||
return getBiome(cell.biomeType, cell.temperature, cell.moisture, cell.biome);
|
||||
}
|
||||
|
||||
List<Biome> getAllBiomes(BiomeType type);
|
||||
|
||||
Set<Biome> getBiomes(BiomeType type);
|
||||
|
||||
Set<Biome> getRivers(Biome.TemperatureGroup temp);
|
||||
|
||||
Set<Biome> getOceanBiomes(Biome.TemperatureGroup temp);
|
||||
|
||||
Set<Biome> getDeepOceanBiomes(Biome.TemperatureGroup temp);
|
||||
|
||||
JsonElement toJson();
|
||||
|
||||
interface Builder {
|
||||
|
||||
Builder addBeach(Biome biome, int count);
|
||||
|
||||
Builder addRiver(Biome biome, int count);
|
||||
|
||||
Builder addWetland(Biome biome, int count);
|
||||
|
||||
Builder addOcean(Biome biome, int count);
|
||||
|
||||
Builder addBiome(BiomeType type, Biome biome, int count);
|
||||
|
||||
BiomeMap build();
|
||||
}
|
||||
}
|
@ -0,0 +1,188 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.biome.map;
|
||||
|
||||
import com.terraforged.core.util.grid.FixedGrid;
|
||||
import com.terraforged.core.world.biome.BiomeData;
|
||||
import com.terraforged.core.world.biome.BiomeType;
|
||||
import com.terraforged.mod.biome.provider.BiomeHelper;
|
||||
import com.terraforged.mod.util.ListUtils;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
|
||||
import java.util.ArrayList;
|
||||
import java.util.Collections;
|
||||
import java.util.Comparator;
|
||||
import java.util.EnumMap;
|
||||
import java.util.HashMap;
|
||||
import java.util.List;
|
||||
import java.util.Map;
|
||||
import java.util.function.Function;
|
||||
|
||||
public class BiomeMapBuilder implements BiomeMap.Builder {
|
||||
|
||||
private final Map<Biome.TemperatureGroup, List<Biome>> rivers = new HashMap<>();
|
||||
private final Map<Biome.TemperatureGroup, List<Biome>> wetlands = new HashMap<>();
|
||||
private final Map<Biome.TemperatureGroup, List<Biome>> beaches = new HashMap<>();
|
||||
private final Map<Biome.TemperatureGroup, List<Biome>> oceans = new HashMap<>();
|
||||
private final Map<Biome.TemperatureGroup, List<Biome>> deepOceans = new HashMap<>();
|
||||
private final Map<BiomeType, List<Biome>> map = new EnumMap<>(BiomeType.class);
|
||||
private final Map<Biome, BiomeData> dataMap = new HashMap<>();
|
||||
|
||||
private final int gridSize;
|
||||
private final Function<BiomeMapBuilder, BiomeMap> constructor;
|
||||
|
||||
BiomeMapBuilder(Function<BiomeMapBuilder, BiomeMap> constructor, int gridSize, List<BiomeData> biomes) {
|
||||
this.constructor = constructor;
|
||||
this.gridSize = gridSize;
|
||||
|
||||
for (BiomeData data : biomes) {
|
||||
dataMap.put((Biome) data.reference, data);
|
||||
}
|
||||
}
|
||||
|
||||
@Override
|
||||
public BiomeMapBuilder addOcean(Biome biome, int count) {
|
||||
Biome.TemperatureGroup category = BiomeHelper.getTemperatureGroup(biome);
|
||||
if (biome.getDepth() < -1) {
|
||||
add(deepOceans.computeIfAbsent(category, c -> new ArrayList<>()), biome, count);
|
||||
} else {
|
||||
add(oceans.computeIfAbsent(category, c -> new ArrayList<>()), biome, count);
|
||||
}
|
||||
return this;
|
||||
}
|
||||
|
||||
@Override
|
||||
public BiomeMap.Builder addBeach(Biome biome, int count) {
|
||||
Biome.TemperatureGroup category = BiomeHelper.getTemperatureGroup(biome);
|
||||
add(beaches.computeIfAbsent(category, c -> new ArrayList<>()), biome, count);
|
||||
return this;
|
||||
}
|
||||
|
||||
@Override
|
||||
public BiomeMapBuilder addRiver(Biome biome, int count) {
|
||||
Biome.TemperatureGroup category = BiomeHelper.getTemperatureGroup(biome);
|
||||
add(rivers.computeIfAbsent(category, c -> new ArrayList<>()), biome, count);
|
||||
return this;
|
||||
}
|
||||
|
||||
@Override
|
||||
public BiomeMapBuilder addWetland(Biome biome, int count) {
|
||||
Biome.TemperatureGroup category = BiomeHelper.getTemperatureGroup(biome);
|
||||
add(wetlands.computeIfAbsent(category, c -> new ArrayList<>()), biome, count);
|
||||
return this;
|
||||
}
|
||||
|
||||
@Override
|
||||
public BiomeMapBuilder addBiome(BiomeType type, Biome biome, int count) {
|
||||
add(map.computeIfAbsent(type, t -> new ArrayList<>()), biome, count);
|
||||
return this;
|
||||
}
|
||||
|
||||
@Override
|
||||
public BiomeMap build() {
|
||||
return constructor.apply(this);
|
||||
}
|
||||
|
||||
Biome[][] rivers() {
|
||||
return collectTemps(rivers);
|
||||
}
|
||||
|
||||
Biome[][] wetlands() {
|
||||
return collectTemps(wetlands);
|
||||
}
|
||||
|
||||
Biome[][] beaches() {
|
||||
return collectTemps(beaches);
|
||||
}
|
||||
|
||||
Biome[][] oceans() {
|
||||
return collectTemps(oceans);
|
||||
}
|
||||
|
||||
Biome[][] deepOceans() {
|
||||
return collectTemps(deepOceans);
|
||||
}
|
||||
|
||||
Biome[][] biomeList() {
|
||||
return collectTypes(map);
|
||||
}
|
||||
|
||||
BiomeGroup[] biomeGroups() {
|
||||
BiomeGroup[] biomes = new BiomeGroup[BiomeType.values().length];
|
||||
|
||||
Function<Biome, Float> moisture = b -> dataMap.get(b).rainfall;
|
||||
Function<Biome, Float> temperature = b -> dataMap.get(b).temperature;
|
||||
for (BiomeType type : BiomeType.values()) {
|
||||
List<Biome> list = map.getOrDefault(type, Collections.emptyList());
|
||||
if (list.isEmpty()) {
|
||||
continue;
|
||||
}
|
||||
FixedGrid<Biome> grid = FixedGrid.generate(gridSize, list, moisture, temperature);
|
||||
biomes[type.ordinal()] = new BiomeGroup(grid);
|
||||
}
|
||||
|
||||
return biomes;
|
||||
}
|
||||
|
||||
private void add(List<Biome> list, Biome biome, int count) {
|
||||
for (int i = 0; i < count; i++) {
|
||||
list.add(biome);
|
||||
}
|
||||
}
|
||||
|
||||
private Biome[][] collectTemps(Map<Biome.TemperatureGroup, List<Biome>> map) {
|
||||
Biome[][] biomes = new Biome[3][];
|
||||
for (Biome.TemperatureGroup category : Biome.TemperatureGroup.values()) {
|
||||
if (category == Biome.TemperatureGroup.OCEAN) {
|
||||
continue;
|
||||
}
|
||||
List<Biome> list = map.getOrDefault(category, Collections.emptyList());
|
||||
list = ListUtils.minimize(list);
|
||||
list.sort(Comparator.comparing(BiomeHelper::getId));
|
||||
biomes[category.ordinal() - 1] = list.toArray(new Biome[0]);
|
||||
}
|
||||
return biomes;
|
||||
}
|
||||
|
||||
private Biome[][] collectTypes(Map<BiomeType, List<Biome>> map) {
|
||||
Biome[][] biomes = new Biome[BiomeType.values().length][];
|
||||
for (BiomeType type : BiomeType.values()) {
|
||||
List<Biome> list = map.getOrDefault(type, Collections.emptyList());
|
||||
list = ListUtils.minimize(list);
|
||||
list.sort(Comparator.comparing(BiomeHelper::getId));
|
||||
biomes[type.ordinal()] = list.toArray(new Biome[0]);
|
||||
}
|
||||
return biomes;
|
||||
}
|
||||
|
||||
public static BiomeMap.Builder basic(List<BiomeData> biomes) {
|
||||
return new BiomeMapBuilder(BasicBiomeMap::new, 0, biomes);
|
||||
}
|
||||
|
||||
public static BiomeMap.Builder grid(int size, List<BiomeData> biomes) {
|
||||
return new BiomeMapBuilder(GridBiomeMap::new, size, biomes);
|
||||
}
|
||||
}
|
@ -0,0 +1,101 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.biome.map;
|
||||
|
||||
import com.terraforged.core.world.biome.BiomeData;
|
||||
import com.terraforged.mod.biome.provider.BiomeHelper;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
|
||||
import java.util.function.BiPredicate;
|
||||
|
||||
public interface BiomePredicate {
|
||||
|
||||
boolean test(BiomeData data, Biome biome);
|
||||
|
||||
default boolean test(BiomeData data) {
|
||||
return test(data, (Biome) data.reference);
|
||||
}
|
||||
|
||||
default BiomePredicate and(BiomePredicate other) {
|
||||
return (d, b) -> this.test(d, b) && other.test(d, b);
|
||||
}
|
||||
|
||||
default BiomePredicate not(BiomePredicate other) {
|
||||
return (d, b) -> this.test(d, b) && !other.test(d, b);
|
||||
}
|
||||
|
||||
default BiomePredicate or(BiomePredicate other) {
|
||||
return (d, b) -> this.test(d, b) || other.test(d, b);
|
||||
}
|
||||
|
||||
static BiomePredicate name(String... name) {
|
||||
return (d, b) -> anyMatch(BiomeHelper.getId(b), name, String::contains);
|
||||
}
|
||||
|
||||
static BiomePredicate type(Biome.Category... categories) {
|
||||
return (d, b) -> anyMatch(b.getCategory(), categories, (c1, c2) -> c1 == c2);
|
||||
}
|
||||
|
||||
static BiomePredicate rain(double min, double max) {
|
||||
return (d, b) -> d.rainfall >= min && d.rainfall <= max;
|
||||
}
|
||||
|
||||
static BiomePredicate rainType(Biome.Precipitation ... rainTypes) {
|
||||
return (d, b) -> anyMatch(b.getPrecipitation(), rainTypes, (c1, c2) -> c1 == c2);
|
||||
}
|
||||
|
||||
static BiomePredicate temp(double min, double max) {
|
||||
return (d, b) -> d.temperature >= min && d.temperature <= max;
|
||||
}
|
||||
|
||||
static BiomePredicate depth(double min, double max) {
|
||||
return (d, b) -> b.getDepth() >= min && b.getDepth() <= max;
|
||||
}
|
||||
|
||||
static <T> boolean anyMatch(T value, T[] test, BiPredicate<T, T> tester) {
|
||||
for (T t : test) {
|
||||
if (tester.test(value, t)) {
|
||||
return true;
|
||||
}
|
||||
}
|
||||
return false;
|
||||
}
|
||||
|
||||
BiomePredicate COAST = type(Biome.Category.BEACH);
|
||||
BiomePredicate WETLAND = type(Biome.Category.SWAMP);
|
||||
BiomePredicate DESERT = type(Biome.Category.DESERT).or(temp(0.9, 2).and(rain(-1, 0.2)));
|
||||
BiomePredicate SAVANNA = type(Biome.Category.SAVANNA).or(temp(0.8, 2).and(rain(-1, 0.4)));
|
||||
BiomePredicate MESA = type(Biome.Category.MESA);
|
||||
BiomePredicate STEPPE = name("steppe").and(temp(0.3, 1));
|
||||
BiomePredicate COLD_STEPPE = name("steppe").and(temp(-1, 0.3));
|
||||
BiomePredicate GRASSLAND = type(Biome.Category.PLAINS);
|
||||
BiomePredicate TEMPERATE_FOREST = type(Biome.Category.FOREST).and(rain(-1, 0.81));
|
||||
BiomePredicate TEMPERATE_RAINFOREST = type(Biome.Category.FOREST).and(rain(0.8, 2));
|
||||
BiomePredicate TROPICAL_RAINFOREST = type(Biome.Category.JUNGLE);
|
||||
BiomePredicate TAIGA = type(Biome.Category.TAIGA).or(temp(0.19, 0.35)).not(rainType(Biome.Precipitation.SNOW));
|
||||
BiomePredicate TUNDRA = type(Biome.Category.ICY).or(temp(-1, 0.21).and(rainType(Biome.Precipitation.SNOW)));
|
||||
BiomePredicate MOUNTAIN = type(Biome.Category.EXTREME_HILLS).or(name("mountain"));
|
||||
}
|
@ -0,0 +1,12 @@
|
||||
package com.terraforged.mod.biome.map;
|
||||
|
||||
import net.minecraft.world.biome.Biome;
|
||||
|
||||
public interface DefaultBiome {
|
||||
|
||||
Biome getBiome(float temperature);
|
||||
|
||||
default Biome getDefaultBiome(float temperature) {
|
||||
return getBiome(temperature).delegate.get();
|
||||
}
|
||||
}
|
@ -0,0 +1,128 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.biome.map;
|
||||
|
||||
import com.google.gson.JsonArray;
|
||||
import com.google.gson.JsonObject;
|
||||
import com.terraforged.core.util.grid.FixedList;
|
||||
import com.terraforged.core.util.grid.MappedList;
|
||||
import com.terraforged.core.world.biome.BiomeType;
|
||||
import net.minecraft.util.registry.Registry;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
import net.minecraft.world.biome.Biomes;
|
||||
|
||||
import java.util.Collections;
|
||||
import java.util.HashSet;
|
||||
import java.util.LinkedList;
|
||||
import java.util.List;
|
||||
import java.util.Set;
|
||||
|
||||
public class GridBiomeMap extends AbstractBiomeMap {
|
||||
|
||||
private final BiomeGroup[] biomeTypes;
|
||||
|
||||
GridBiomeMap(BiomeMapBuilder builder) {
|
||||
super(builder);
|
||||
biomeTypes = builder.biomeGroups();
|
||||
}
|
||||
|
||||
@Override
|
||||
public Biome getBiome(BiomeType type, float temperature, float moisture, float shape) {
|
||||
BiomeGroup group = biomeTypes[type.ordinal()];
|
||||
if (group == null) {
|
||||
return Biomes.NETHER;
|
||||
}
|
||||
return group.biomes.get(moisture, temperature, shape);
|
||||
}
|
||||
|
||||
@Override
|
||||
public List<Biome> getAllBiomes(BiomeType type) {
|
||||
BiomeGroup group = biomeTypes[type.ordinal()];
|
||||
if (group == null) {
|
||||
return Collections.emptyList();
|
||||
}
|
||||
List<Biome> biomes = new LinkedList<>();
|
||||
for (MappedList<FixedList<Biome>> row : group.biomes) {
|
||||
for (FixedList<Biome> cell : row) {
|
||||
for (Biome biome : cell) {
|
||||
biomes.add(biome);
|
||||
}
|
||||
}
|
||||
}
|
||||
return biomes;
|
||||
}
|
||||
|
||||
@Override
|
||||
public Set<Biome> getBiomes(BiomeType type) {
|
||||
BiomeGroup group = biomeTypes[type.ordinal()];
|
||||
if (group == null) {
|
||||
return Collections.emptySet();
|
||||
}
|
||||
Set<Biome> biomes = new HashSet<>();
|
||||
for (MappedList<FixedList<Biome>> row : group.biomes) {
|
||||
for (FixedList<Biome> cell : row) {
|
||||
for (Biome biome : cell) {
|
||||
biomes.add(biome);
|
||||
}
|
||||
}
|
||||
}
|
||||
return biomes;
|
||||
}
|
||||
|
||||
@Override
|
||||
public JsonObject toJson() {
|
||||
JsonObject root = new JsonObject();
|
||||
for (BiomeType type : BiomeType.values()) {
|
||||
BiomeGroup group = biomeTypes[type.ordinal()];
|
||||
JsonObject grid = new JsonObject();
|
||||
if (group != null) {
|
||||
int rowCount = 0;
|
||||
float maxRow = group.biomes.size() - 1;
|
||||
for (MappedList<FixedList<Biome>> row : group.biomes) {
|
||||
int colCount = 0;
|
||||
float maxCol = row.size() - 1;
|
||||
|
||||
JsonObject rowJson = new JsonObject();
|
||||
for (FixedList<Biome> cell : row) {
|
||||
JsonArray colJson = new JsonArray();
|
||||
for (Biome biome : cell.uniqueValues()) {
|
||||
colJson.add(String.valueOf(Registry.BIOME.getId(biome)));
|
||||
}
|
||||
float colId = colCount / maxCol;
|
||||
rowJson.add("" + colId, colJson);
|
||||
colCount++;
|
||||
}
|
||||
|
||||
float rowId = rowCount / maxRow;
|
||||
grid.add("" + rowId, rowJson);
|
||||
rowCount++;
|
||||
}
|
||||
}
|
||||
root.add(type.name(), grid);
|
||||
}
|
||||
return root;
|
||||
}
|
||||
}
|
@ -0,0 +1,67 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.biome.modifier;
|
||||
|
||||
import com.terraforged.core.cell.Cell;
|
||||
import com.terraforged.core.util.Seed;
|
||||
import com.terraforged.core.world.climate.Climate;
|
||||
import com.terraforged.core.world.terrain.Terrain;
|
||||
import me.dags.noise.Module;
|
||||
import me.dags.noise.Source;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
|
||||
public abstract class AbstractMaxHeightModifier extends AbstractOffsetModifier {
|
||||
|
||||
private final float minHeight;
|
||||
private final float maxHeight;
|
||||
private final float range;
|
||||
private final Module variance;
|
||||
|
||||
public AbstractMaxHeightModifier(Seed seed, Climate climate, int scale, int octaves, float variance, float minHeight, float maxHeight) {
|
||||
super(climate);
|
||||
this.minHeight = minHeight;
|
||||
this.maxHeight = maxHeight;
|
||||
this.range = maxHeight - minHeight;
|
||||
this.variance = Source.perlin(seed.next(), scale, octaves).scale(variance);
|
||||
}
|
||||
|
||||
@Override
|
||||
protected final Biome modify(Biome in, Cell<Terrain> cell, int x, int z, float ox, float oz) {
|
||||
float var = variance.getValue(x, z);
|
||||
float value = cell.value + var;
|
||||
if (value < minHeight) {
|
||||
return in;
|
||||
}
|
||||
if (value > maxHeight) {
|
||||
return getModifiedBiome(in, cell, x, z, ox, oz);
|
||||
}
|
||||
float alpha = (value - minHeight) / range;
|
||||
cell.biomeEdge *= alpha;
|
||||
return in;
|
||||
}
|
||||
|
||||
protected abstract Biome getModifiedBiome(Biome in, Cell<Terrain> cell, int x, int z, float ox, float oz);
|
||||
}
|
@ -0,0 +1,50 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.biome.modifier;
|
||||
|
||||
import com.terraforged.api.biome.modifier.BiomeModifier;
|
||||
import com.terraforged.core.cell.Cell;
|
||||
import com.terraforged.core.world.climate.Climate;
|
||||
import com.terraforged.core.world.terrain.Terrain;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
|
||||
public abstract class AbstractOffsetModifier implements BiomeModifier {
|
||||
|
||||
private final Climate climate;
|
||||
|
||||
public AbstractOffsetModifier(Climate climate) {
|
||||
this.climate = climate;
|
||||
}
|
||||
|
||||
@Override
|
||||
public Biome modify(Biome in, Cell<Terrain> cell, int x, int z) {
|
||||
float dx = climate.getOffsetX(x, z, 50);
|
||||
float dz = climate.getOffsetX(x, z, 50);
|
||||
return modify(in, cell, x, z, x + dx, z + dz);
|
||||
}
|
||||
|
||||
protected abstract Biome modify(Biome in, Cell<Terrain> cell, int x, int z, float ox, float oz);
|
||||
}
|
@ -0,0 +1,62 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.biome.modifier;
|
||||
|
||||
import com.terraforged.api.biome.modifier.BiomeModifier;
|
||||
import com.terraforged.core.cell.Cell;
|
||||
import com.terraforged.core.world.terrain.Terrain;
|
||||
import com.terraforged.core.world.terrain.Terrains;
|
||||
import com.terraforged.mod.biome.map.BiomeMap;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
|
||||
public class BeachModifier implements BiomeModifier {
|
||||
|
||||
private final Terrains terrain;
|
||||
private final BiomeMap biomeMap;
|
||||
|
||||
public BeachModifier(BiomeMap biomeMap, Terrains terrain) {
|
||||
this.terrain = terrain;
|
||||
this.biomeMap = biomeMap;
|
||||
}
|
||||
|
||||
@Override
|
||||
public int priority() {
|
||||
return 0;
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean test(Biome biome) {
|
||||
return true;
|
||||
}
|
||||
|
||||
@Override
|
||||
public Biome modify(Biome in, Cell<Terrain> cell, int x, int z) {
|
||||
if (cell.tag == terrain.beach) {
|
||||
return biomeMap.getBeach(cell.temperature, cell.moisture, cell.biome);
|
||||
}
|
||||
return in;
|
||||
}
|
||||
}
|
@ -0,0 +1,90 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.biome.modifier;
|
||||
|
||||
import com.terraforged.api.biome.modifier.BiomeModifier;
|
||||
import com.terraforged.api.biome.modifier.ModifierManager;
|
||||
import com.terraforged.core.cell.Cell;
|
||||
import com.terraforged.core.world.biome.BiomeType;
|
||||
import com.terraforged.core.world.terrain.Terrain;
|
||||
import com.terraforged.mod.biome.map.BiomeMap;
|
||||
import com.terraforged.mod.biome.provider.DesertBiomes;
|
||||
import com.terraforged.mod.chunk.TerraContext;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
|
||||
import java.util.ArrayList;
|
||||
import java.util.Collections;
|
||||
import java.util.List;
|
||||
|
||||
public class BiomeModifierManager implements BiomeModifier, ModifierManager {
|
||||
|
||||
private final DesertBiomes desertBiomes;
|
||||
private final List<BiomeModifier> biomeModifiers;
|
||||
|
||||
public BiomeModifierManager(TerraContext context, BiomeMap biomes) {
|
||||
desertBiomes = new DesertBiomes(context.materials, biomes.getAllBiomes(BiomeType.DESERT));
|
||||
List<BiomeModifier> modifiers = new ArrayList<>();
|
||||
modifiers.add(new BeachModifier(biomes, context.terrain));
|
||||
modifiers.add(new DesertColorModifier(desertBiomes));
|
||||
modifiers.add(new SandBiomeModifier(
|
||||
context.seed,
|
||||
context.factory.getClimate(),
|
||||
context.levels
|
||||
));
|
||||
Collections.sort(modifiers);
|
||||
this.biomeModifiers = modifiers;
|
||||
}
|
||||
|
||||
@Override
|
||||
public void register(BiomeModifier modifier) {
|
||||
biomeModifiers.add(modifier);
|
||||
Collections.sort(biomeModifiers);
|
||||
}
|
||||
|
||||
public DesertBiomes getDesertBiomes() {
|
||||
return desertBiomes;
|
||||
}
|
||||
|
||||
@Override
|
||||
public int priority() {
|
||||
return -1;
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean test(Biome biome) {
|
||||
return true;
|
||||
}
|
||||
|
||||
@Override
|
||||
public Biome modify(Biome biome, Cell<Terrain> cell, int x, int z) {
|
||||
for (BiomeModifier modifier : biomeModifiers) {
|
||||
if (modifier.test(biome)) {
|
||||
biome = modifier.modify(biome, cell, x, z);
|
||||
}
|
||||
}
|
||||
return biome;
|
||||
}
|
||||
}
|
@ -0,0 +1,63 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.biome.modifier;
|
||||
|
||||
import com.terraforged.api.biome.modifier.BiomeModifier;
|
||||
import com.terraforged.core.cell.Cell;
|
||||
import com.terraforged.core.world.terrain.Terrain;
|
||||
import com.terraforged.mod.biome.provider.DesertBiomes;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
|
||||
public class DesertColorModifier implements BiomeModifier {
|
||||
|
||||
private final DesertBiomes biomes;
|
||||
|
||||
public DesertColorModifier(DesertBiomes biomes) {
|
||||
this.biomes = biomes;
|
||||
}
|
||||
|
||||
@Override
|
||||
public int priority() {
|
||||
return 0;
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean test(Biome biome) {
|
||||
return biome.getCategory() == Biome.Category.DESERT;
|
||||
}
|
||||
|
||||
@Override
|
||||
public Biome modify(Biome in, Cell<Terrain> cell, int x, int z) {
|
||||
if (biomes.isRedDesert(in)) {
|
||||
if (cell.continent <= 0.5F) {
|
||||
return biomes.getWhiteDesert(cell.biome);
|
||||
}
|
||||
} else if (cell.continent > 0.5F) {
|
||||
return biomes.getRedDesert(cell.biome);
|
||||
}
|
||||
return in;
|
||||
}
|
||||
}
|
@ -0,0 +1,67 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.biome.modifier;
|
||||
|
||||
import com.terraforged.core.cell.Cell;
|
||||
import com.terraforged.core.util.Seed;
|
||||
import com.terraforged.core.world.climate.Climate;
|
||||
import com.terraforged.core.world.heightmap.Levels;
|
||||
import com.terraforged.core.world.terrain.Terrain;
|
||||
import com.terraforged.mod.material.MaterialHelper;
|
||||
import net.minecraft.util.registry.Registry;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
import net.minecraft.world.biome.Biomes;
|
||||
|
||||
import java.util.Set;
|
||||
import java.util.stream.Collectors;
|
||||
|
||||
// prevents deserts forming at high levels
|
||||
public class SandBiomeModifier extends AbstractMaxHeightModifier {
|
||||
|
||||
private final Set<Biome> biomes;
|
||||
|
||||
public SandBiomeModifier(Seed seed, Climate climate, Levels levels) {
|
||||
super(seed, climate, 50, 2, levels.scale(8), levels.ground(5), levels.ground(25));
|
||||
this.biomes = Registry.BIOME.stream()
|
||||
.filter(biome -> MaterialHelper.isSand(biome.getSurfaceConfig().getTopMaterial().getBlock()))
|
||||
.collect(Collectors.toSet());
|
||||
}
|
||||
|
||||
@Override
|
||||
public int priority() {
|
||||
return 1;
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean test(Biome biome) {
|
||||
return biome.getCategory() == Biome.Category.DESERT || biomes.contains(biome);
|
||||
}
|
||||
|
||||
@Override
|
||||
protected Biome getModifiedBiome(Biome in, Cell<Terrain> cell, int x, int z, float ox, float oz) {
|
||||
return Biomes.BADLANDS;
|
||||
}
|
||||
}
|
@ -0,0 +1,55 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.biome.provider;
|
||||
|
||||
import com.google.common.collect.ImmutableSet;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
import net.minecraft.world.biome.Biomes;
|
||||
import net.minecraft.world.biome.source.BiomeSource;
|
||||
|
||||
import java.util.Set;
|
||||
|
||||
public abstract class AbstractBiomeProvider extends BiomeSource {
|
||||
|
||||
protected static final Set<Biome> defaultBiomes = ImmutableSet
|
||||
.of(Biomes.OCEAN, Biomes.PLAINS, Biomes.DESERT, Biomes.MOUNTAINS, Biomes.FOREST, Biomes.TAIGA, Biomes.SWAMP, Biomes.RIVER,
|
||||
Biomes.FROZEN_OCEAN, Biomes.FROZEN_RIVER, Biomes.SNOWY_TUNDRA, Biomes.SNOWY_MOUNTAINS, Biomes.MUSHROOM_FIELDS,
|
||||
Biomes.MUSHROOM_FIELD_SHORE, Biomes.BEACH, Biomes.DESERT_HILLS, Biomes.WOODED_HILLS, Biomes.TAIGA_HILLS, Biomes.MOUNTAIN_EDGE,
|
||||
Biomes.JUNGLE, Biomes.JUNGLE_HILLS, Biomes.JUNGLE_EDGE, Biomes.DEEP_OCEAN, Biomes.STONE_SHORE, Biomes.SNOWY_BEACH,
|
||||
Biomes.BIRCH_FOREST, Biomes.BIRCH_FOREST_HILLS, Biomes.DARK_FOREST, Biomes.SNOWY_TAIGA, Biomes.SNOWY_TAIGA_HILLS,
|
||||
Biomes.GIANT_TREE_TAIGA, Biomes.GIANT_TREE_TAIGA_HILLS, Biomes.WOODED_MOUNTAINS, Biomes.SAVANNA, Biomes.SAVANNA_PLATEAU,
|
||||
Biomes.BADLANDS, Biomes.WOODED_BADLANDS_PLATEAU, Biomes.BADLANDS_PLATEAU, Biomes.WARM_OCEAN, Biomes.LUKEWARM_OCEAN,
|
||||
Biomes.COLD_OCEAN, Biomes.DEEP_WARM_OCEAN, Biomes.DEEP_LUKEWARM_OCEAN, Biomes.DEEP_COLD_OCEAN, Biomes.DEEP_FROZEN_OCEAN,
|
||||
Biomes.SUNFLOWER_PLAINS, Biomes.DESERT_LAKES, Biomes.GRAVELLY_MOUNTAINS, Biomes.FLOWER_FOREST, Biomes.TAIGA_MOUNTAINS,
|
||||
Biomes.SWAMP_HILLS, Biomes.ICE_SPIKES, Biomes.MODIFIED_JUNGLE, Biomes.MODIFIED_JUNGLE_EDGE, Biomes.TALL_BIRCH_FOREST,
|
||||
Biomes.TALL_BIRCH_HILLS, Biomes.DARK_FOREST_HILLS, Biomes.SNOWY_TAIGA_MOUNTAINS, Biomes.GIANT_SPRUCE_TAIGA,
|
||||
Biomes.GIANT_SPRUCE_TAIGA_HILLS, Biomes.MODIFIED_GRAVELLY_MOUNTAINS, Biomes.SHATTERED_SAVANNA, Biomes.SHATTERED_SAVANNA_PLATEAU,
|
||||
Biomes.ERODED_BADLANDS, Biomes.MODIFIED_WOODED_BADLANDS_PLATEAU, Biomes.MODIFIED_BADLANDS_PLATEAU);
|
||||
|
||||
protected AbstractBiomeProvider() {
|
||||
super(defaultBiomes);
|
||||
}
|
||||
}
|
@ -0,0 +1,234 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.biome.provider;
|
||||
|
||||
import com.terraforged.core.settings.BiomeSettings;
|
||||
import com.terraforged.core.world.biome.BiomeData;
|
||||
import com.terraforged.core.world.biome.BiomeType;
|
||||
import com.terraforged.mod.biome.ModBiomes;
|
||||
import com.terraforged.mod.biome.map.BiomeMap;
|
||||
import com.terraforged.mod.biome.map.BiomeMapBuilder;
|
||||
import com.terraforged.mod.biome.map.BiomePredicate;
|
||||
import me.dags.noise.util.Vec2f;
|
||||
import net.minecraft.util.Identifier;
|
||||
import net.minecraft.util.registry.Registry;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
import net.minecraft.world.biome.Biomes;
|
||||
import net.minecraft.world.gen.feature.Feature;
|
||||
import net.minecraft.world.gen.feature.OceanRuinFeature;
|
||||
import net.minecraft.world.gen.feature.OceanRuinFeatureConfig;
|
||||
|
||||
import java.util.Collection;
|
||||
import java.util.HashMap;
|
||||
import java.util.HashSet;
|
||||
import java.util.LinkedList;
|
||||
import java.util.List;
|
||||
import java.util.Map;
|
||||
import java.util.Set;
|
||||
import java.util.function.Function;
|
||||
import java.util.stream.Collectors;
|
||||
|
||||
public class BiomeHelper {
|
||||
|
||||
private static final Map<BiomeType, BiomePredicate> PREDICATES = new HashMap<BiomeType, BiomePredicate>() {{
|
||||
put(BiomeType.TROPICAL_RAINFOREST, BiomePredicate.TROPICAL_RAINFOREST);
|
||||
put(BiomeType.SAVANNA,
|
||||
BiomePredicate.SAVANNA.or(BiomePredicate.MESA).not(BiomePredicate.DESERT).not(BiomePredicate.STEPPE).not(BiomePredicate.COAST)
|
||||
.not(BiomePredicate.MOUNTAIN).not(BiomePredicate.WETLAND));
|
||||
put(BiomeType.DESERT,
|
||||
BiomePredicate.DESERT.or(BiomePredicate.MESA).not(BiomePredicate.COAST).not(BiomePredicate.MOUNTAIN).not(BiomePredicate.WETLAND));
|
||||
put(BiomeType.TEMPERATE_RAINFOREST, BiomePredicate.TEMPERATE_RAINFOREST.not(BiomePredicate.COAST).not(BiomePredicate.MOUNTAIN));
|
||||
put(BiomeType.TEMPERATE_FOREST,
|
||||
BiomePredicate.TEMPERATE_FOREST.not(BiomePredicate.COAST).not(BiomePredicate.MOUNTAIN).not(BiomePredicate.WETLAND));
|
||||
put(BiomeType.GRASSLAND, BiomePredicate.GRASSLAND.not(BiomePredicate.WETLAND).not(BiomePredicate.COAST).not(BiomePredicate.MOUNTAIN));
|
||||
put(BiomeType.COLD_STEPPE, BiomePredicate.COLD_STEPPE.not(BiomePredicate.COAST).not(BiomePredicate.MOUNTAIN));
|
||||
put(BiomeType.STEPPE, BiomePredicate.STEPPE.not(BiomePredicate.COAST).not(BiomePredicate.MOUNTAIN));
|
||||
put(BiomeType.TAIGA, BiomePredicate.TAIGA.not(BiomePredicate.TUNDRA).not(BiomePredicate.COLD_STEPPE).not(BiomePredicate.COAST)
|
||||
.not(BiomePredicate.MOUNTAIN));
|
||||
put(BiomeType.TUNDRA, BiomePredicate.TUNDRA.not(BiomePredicate.TAIGA).not(BiomePredicate.COAST).not(BiomePredicate.MOUNTAIN));
|
||||
put(BiomeType.ALPINE, BiomePredicate.MOUNTAIN);
|
||||
}};
|
||||
|
||||
public static BiomeMap.Builder getBuilder(List<BiomeData> biomes) {
|
||||
return BiomeMapBuilder.basic(biomes);
|
||||
}
|
||||
|
||||
public static BiomeMap getDefaultBiomeMap() {
|
||||
List<BiomeData> biomes = getAllBiomeData();
|
||||
BiomeMap.Builder builder = getBuilder(biomes);
|
||||
for (BiomeData data : biomes) {
|
||||
int weight = 10;
|
||||
Biome biome = (Biome) data.reference;
|
||||
|
||||
if (biome.hasParent() && getId(biome).contains("hills")) {
|
||||
continue;
|
||||
}
|
||||
|
||||
if (biome.getCategory() == Biome.Category.FOREST) {
|
||||
weight = 5;
|
||||
}
|
||||
|
||||
if (biome.getCategory() == Biome.Category.MUSHROOM) {
|
||||
weight = 1;
|
||||
}
|
||||
|
||||
// don't use BiomeDictionary with transient biomes todo detect rare
|
||||
// if (ForgeRegistries.BIOMES.containsKey(biome.getRegistryName())) {
|
||||
// if (BiomeDictionary.getTypes(biome).contains(BiomeDictionary.Type.RARE)) {
|
||||
// weight = 1;
|
||||
// }
|
||||
// }
|
||||
|
||||
if (biome.getCategory() == Biome.Category.OCEAN) {
|
||||
builder.addOcean(biome, weight);
|
||||
} else if (biome.getCategory() == Biome.Category.RIVER) {
|
||||
builder.addRiver(biome, weight);
|
||||
} else if (biome.getCategory() == Biome.Category.BEACH || biome == Biomes.STONE_SHORE) {
|
||||
builder.addBeach(biome, weight);
|
||||
} else if (biome.getCategory() == Biome.Category.SWAMP) {
|
||||
builder.addWetland(biome, weight);
|
||||
} else {
|
||||
Collection<BiomeType> types = getTypes(data, biome);
|
||||
for (BiomeType type : types) {
|
||||
builder.addBiome(type, biome, weight);
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
builder.addBiome(BiomeType.TEMPERATE_RAINFOREST, Biomes.PLAINS, 10);
|
||||
builder.addBiome(BiomeType.TEMPERATE_FOREST, Biomes.FLOWER_FOREST, 3);
|
||||
builder.addBiome(BiomeType.TEMPERATE_FOREST, Biomes.PLAINS, 10);
|
||||
builder.addBiome(BiomeType.TUNDRA, ModBiomes.SNOWY_TAIGA_SCRUB, 5);
|
||||
builder.addBiome(BiomeType.TAIGA, ModBiomes.TAIGA_SCRUB, 5);
|
||||
|
||||
return builder.build();
|
||||
}
|
||||
|
||||
public static BiomeMap getBiomeMap(BiomeSettings settings) {
|
||||
List<BiomeData> biomes = getAllBiomeData();
|
||||
BiomeMap.Builder builder = getBuilder(biomes);
|
||||
Map<String, BiomeData> biomeMap = biomes.stream().collect(Collectors.toMap(d -> d.name, d -> d));
|
||||
Map<BiomeType, BiomeSettings.BiomeGroup> groupMap = settings.asMap();
|
||||
for (Map.Entry<BiomeType, BiomeSettings.BiomeGroup> e : groupMap.entrySet()) {
|
||||
for (BiomeSettings.BiomeWeight biomeWeight : e.getValue().biomes) {
|
||||
BiomeData data = biomeMap.get(biomeWeight.id);
|
||||
if (data == null) {
|
||||
continue;
|
||||
}
|
||||
builder.addBiome(e.getKey(), (Biome) data.reference, biomeWeight.weight);
|
||||
}
|
||||
}
|
||||
for (BiomeData data : biomes) {
|
||||
Biome biome = (Biome) data.reference;
|
||||
if (biome.getCategory() == Biome.Category.OCEAN) {
|
||||
builder.addOcean(biome, 10);
|
||||
} else if (biome.getCategory() == Biome.Category.RIVER) {
|
||||
builder.addRiver(biome, 10);
|
||||
}
|
||||
}
|
||||
return builder.build();
|
||||
}
|
||||
|
||||
public static Biome.TemperatureGroup getTemperatureGroup(Biome biome) {
|
||||
// vanilla ocean biome properties are not at all helpful for determining temperature
|
||||
if (biome.getCategory() == Biome.Category.OCEAN) {
|
||||
// warm & luke_warm oceans get OceanRuinStructure.Type.WARM
|
||||
OceanRuinFeatureConfig config = biome.getStructureFeatureConfig(Feature.OCEAN_RUIN);
|
||||
if (config != null) {
|
||||
if (config.biomeType == OceanRuinFeature.BiomeType.WARM) {
|
||||
return Biome.TemperatureGroup.WARM;
|
||||
}
|
||||
}
|
||||
|
||||
// if the id contains the world cold or frozen, assume it's cold
|
||||
if (getId(biome).contains("cold") || getId(biome).contains("frozen")) {
|
||||
return Biome.TemperatureGroup.COLD;
|
||||
}
|
||||
|
||||
// the rest we categorize as medium
|
||||
return Biome.TemperatureGroup.MEDIUM;
|
||||
}
|
||||
// hopefully biomes otherwise have a sensible category
|
||||
return biome.getTemperatureGroup();
|
||||
}
|
||||
|
||||
public static String getId(Biome biome) {
|
||||
Identifier name = Registry.BIOME.getId(biome);
|
||||
if (name == null) {
|
||||
return "unknown";
|
||||
}
|
||||
return name.toString();
|
||||
}
|
||||
|
||||
public static Collection<BiomeType> getTypes(BiomeData data, Biome biome) {
|
||||
Set<BiomeType> types = new HashSet<>();
|
||||
for (Map.Entry<BiomeType, BiomePredicate> entry : PREDICATES.entrySet()) {
|
||||
if (entry.getValue().test(data, biome)) {
|
||||
types.add(entry.getKey());
|
||||
}
|
||||
}
|
||||
return types;
|
||||
}
|
||||
|
||||
public static List<BiomeData> getAllBiomeData() {
|
||||
Collection<Biome> biomes = TerraBiomeRegistry.getInstance().getAll(BiomeHelper::filter);
|
||||
Vec2f tempRange = getRange(biomes, Biome::getTemperature);
|
||||
Vec2f moistRange = getRange(biomes, Biome::getRainfall);
|
||||
List<BiomeData> list = new LinkedList<>();
|
||||
for (Biome biome : biomes) {
|
||||
String name = getId(biome);
|
||||
float moisture = (biome.getRainfall() - moistRange.x) / (moistRange.y - moistRange.x);
|
||||
float temperature = (biome.getTemperature() - tempRange.x) / (tempRange.y - tempRange.x);
|
||||
int color = biome.getSurfaceConfig().getTopMaterial().getMaterial().getColor().color;
|
||||
list.add(new BiomeData(name, biome, color, moisture, temperature));
|
||||
}
|
||||
return list;
|
||||
}
|
||||
|
||||
private static boolean filter(Biome biome) {
|
||||
if (biome.getCategory() == Biome.Category.NONE) {
|
||||
return true;
|
||||
}
|
||||
if (biome.getCategory() == Biome.Category.THEEND) {
|
||||
return true;
|
||||
}
|
||||
if (biome.getCategory() == Biome.Category.NETHER) {
|
||||
return true;
|
||||
}
|
||||
return false; //!BiomeDictionary.getTypes(biome).contains(BiomeDictionary.Type.OVERWORLD); todo dict
|
||||
}
|
||||
|
||||
private static Vec2f getRange(Collection<Biome> biomes, Function<Biome, Float> getter) {
|
||||
float min = Float.MAX_VALUE;
|
||||
float max = Float.MIN_VALUE;
|
||||
for (Biome biome : biomes) {
|
||||
float value = getter.apply(biome);
|
||||
min = Math.min(min, value);
|
||||
max = Math.max(max, value);
|
||||
}
|
||||
return new Vec2f(min, max);
|
||||
}
|
||||
}
|
@ -0,0 +1,187 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.biome.provider;
|
||||
|
||||
import com.google.common.collect.Sets;
|
||||
import com.terraforged.core.cell.Cell;
|
||||
import com.terraforged.core.region.chunk.ChunkReader;
|
||||
import com.terraforged.core.world.decorator.Decorator;
|
||||
import com.terraforged.core.world.heightmap.WorldLookup;
|
||||
import com.terraforged.core.world.terrain.Terrain;
|
||||
import com.terraforged.mod.biome.map.BiomeMap;
|
||||
import com.terraforged.mod.biome.modifier.BiomeModifierManager;
|
||||
import com.terraforged.mod.chunk.TerraBiomeArray;
|
||||
import com.terraforged.mod.chunk.TerraContext;
|
||||
import com.terraforged.mod.util.setup.SetupHooks;
|
||||
import net.minecraft.block.BlockState;
|
||||
import net.minecraft.util.math.BlockPos;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
import net.minecraft.world.gen.feature.StructureFeature;
|
||||
|
||||
import java.util.Collections;
|
||||
import java.util.HashMap;
|
||||
import java.util.HashSet;
|
||||
import java.util.List;
|
||||
import java.util.Map;
|
||||
import java.util.Random;
|
||||
import java.util.Set;
|
||||
|
||||
public class BiomeProvider extends AbstractBiomeProvider {
|
||||
|
||||
private final BiomeMap biomeMap;
|
||||
private final TerraContext context;
|
||||
private final WorldLookup worldLookup;
|
||||
private final BiomeModifierManager modifierManager;
|
||||
private final Map<Biome, List<Decorator>> decorators = new HashMap<>();
|
||||
|
||||
public BiomeProvider(TerraContext context) {
|
||||
this.context = context;
|
||||
this.biomeMap = BiomeHelper.getDefaultBiomeMap();
|
||||
this.worldLookup = new WorldLookup(context.factory, context);
|
||||
this.modifierManager = SetupHooks.setup(new BiomeModifierManager(context, biomeMap), context.copy());
|
||||
}
|
||||
|
||||
public Cell<Terrain> lookupPos(int x, int z) {
|
||||
return worldLookup.getCell(x, z);
|
||||
}
|
||||
|
||||
@Override
|
||||
public Biome getBiomeForNoiseGen(int x, int y, int z) {
|
||||
x = (x << 2);
|
||||
z = (z << 2);
|
||||
return getBiome(lookupPos(x, z), x, z);
|
||||
}
|
||||
|
||||
@Override
|
||||
public Set<Biome> getBiomesInArea(int centerX, int centerY, int centerZ, int sideLength) {
|
||||
int minX = centerX - (sideLength >> 2);
|
||||
int minZ = centerZ - (sideLength >> 2);
|
||||
int maxX = centerX + (sideLength >> 2);
|
||||
int maxZ = centerZ + (sideLength >> 2);
|
||||
Set<Biome> biomes = Sets.newHashSet();
|
||||
context.heightmap.visit(minX, minZ, maxX, maxZ, (cell, x, z) -> {
|
||||
Biome biome = getBiome(cell, minX + x, minZ + z);
|
||||
biomes.add(biome);
|
||||
});
|
||||
return biomes;
|
||||
}
|
||||
|
||||
@Override
|
||||
public BlockPos locateBiome(int centerX, int centerY, int centerZ, int range, List<Biome> biomes, Random random) {
|
||||
int minX = centerX - (range >> 2);
|
||||
int minZ = centerZ - (range >> 2);
|
||||
int maxX = centerX + (range >> 2);
|
||||
int maxZ = centerZ + (range >> 2);
|
||||
Set<Biome> matchBiomes = new HashSet<>(biomes);
|
||||
SearchContext search = new SearchContext();
|
||||
context.heightmap.visit(minX, minZ, maxX, maxZ, (cell, x, z) -> {
|
||||
Biome biome = getBiome(cell, minX + x, minZ + z);
|
||||
if (matchBiomes.contains(biome)) {
|
||||
if (search.first || random.nextInt(search.count + 1) == 0) {
|
||||
search.first = false;
|
||||
search.pos.set(minX + x, 0, minZ + z);
|
||||
}
|
||||
++search.count;
|
||||
}
|
||||
});
|
||||
return search.pos;
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean hasStructureFeature(StructureFeature<?> structureIn) {
|
||||
return this.structureFeatures.computeIfAbsent(structureIn, (p_205006_1_) -> {
|
||||
for (Biome biome : defaultBiomes) {
|
||||
if (biome.hasStructureFeature(p_205006_1_)) {
|
||||
return true;
|
||||
}
|
||||
}
|
||||
return false;
|
||||
});
|
||||
}
|
||||
|
||||
@Override
|
||||
public Set<BlockState> getTopMaterials() {
|
||||
if (this.topMaterials.isEmpty()) {
|
||||
for (Biome biome : defaultBiomes) {
|
||||
this.topMaterials.add(biome.getSurfaceConfig().getTopMaterial());
|
||||
}
|
||||
}
|
||||
return this.topMaterials;
|
||||
}
|
||||
|
||||
public BiomeModifierManager getModifierManager() {
|
||||
return modifierManager;
|
||||
}
|
||||
|
||||
public List<Decorator> getDecorators(Biome biome) {
|
||||
return decorators.getOrDefault(biome, Collections.emptyList());
|
||||
}
|
||||
|
||||
public TerraBiomeArray createBiomeContainer(ChunkReader chunkReader) {
|
||||
TerraBiomeArray.Builder builder = TerraBiomeArray.builder();
|
||||
chunkReader.iterate((cell, dx, dz) -> {
|
||||
Biome biome = getBiome(cell, chunkReader.getBlockX() + dx, chunkReader.getBlockZ() + dz);
|
||||
builder.set(dx, dz, biome);
|
||||
});
|
||||
return builder.build(chunkReader);
|
||||
}
|
||||
|
||||
public Biome getBiome(Cell<Terrain> cell, int x, int z) {
|
||||
if (cell.tag == context.terrain.wetlands) {
|
||||
return biomeMap.getWetland(cell.temperature, cell.moisture, cell.biome);
|
||||
}
|
||||
|
||||
if (cell.value > context.levels.water) {
|
||||
return getModifierManager().modify(biomeMap.getBiome(cell), cell, x, z);
|
||||
}
|
||||
|
||||
if (cell.tag == context.terrain.river || cell.tag == context.terrain.riverBanks) {
|
||||
Biome biome = biomeMap.getBiome(cell);
|
||||
if (overridesRiver(biome)) {
|
||||
return biome;
|
||||
}
|
||||
|
||||
return biomeMap.getRiver(cell.temperature, cell.moisture, cell.biome);
|
||||
}
|
||||
|
||||
if (cell.tag == context.terrain.ocean) {
|
||||
return biomeMap.getOcean(cell.temperature, cell.moisture, cell.biome);
|
||||
}
|
||||
|
||||
return biomeMap.getDeepOcean(cell.temperature, cell.moisture, cell.biome);
|
||||
}
|
||||
|
||||
private static boolean overridesRiver(Biome biome) {
|
||||
return biome.getCategory() == Biome.Category.SWAMP || biome.getCategory() == Biome.Category.JUNGLE;
|
||||
}
|
||||
|
||||
private static class SearchContext {
|
||||
|
||||
private int count = 0;
|
||||
private boolean first = true;
|
||||
private final BlockPos.Mutable pos = new BlockPos.Mutable();
|
||||
}
|
||||
}
|
@ -0,0 +1,117 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.biome.provider;
|
||||
|
||||
import com.terraforged.api.material.layer.LayerManager;
|
||||
import com.terraforged.api.material.layer.LayerMaterial;
|
||||
import com.terraforged.core.util.concurrent.ObjectPool;
|
||||
import com.terraforged.mod.material.Materials;
|
||||
import com.terraforged.mod.util.DummyBlockReader;
|
||||
import com.terraforged.mod.util.ListUtils;
|
||||
import net.minecraft.block.Block;
|
||||
import net.minecraft.block.BlockState;
|
||||
import net.minecraft.block.Blocks;
|
||||
import net.minecraft.block.MaterialColor;
|
||||
import net.minecraft.util.math.BlockPos;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
import net.minecraft.world.biome.Biomes;
|
||||
|
||||
import java.awt.Color;
|
||||
import java.util.ArrayList;
|
||||
import java.util.Comparator;
|
||||
import java.util.HashSet;
|
||||
import java.util.LinkedList;
|
||||
import java.util.List;
|
||||
import java.util.Set;
|
||||
|
||||
public class DesertBiomes {
|
||||
|
||||
private final Set<Biome> reds;
|
||||
private final Set<Biome> whites;
|
||||
private final List<Biome> redSand;
|
||||
private final List<Biome> whiteSand;
|
||||
private final LayerManager layerManager;
|
||||
|
||||
private final int maxRedIndex;
|
||||
private final int maxWhiteIndex;
|
||||
|
||||
public DesertBiomes(Materials materials, List<Biome> deserts) {
|
||||
List<Biome> white = new LinkedList<>();
|
||||
List<Biome> red = new LinkedList<>();
|
||||
try (ObjectPool.Item<DummyBlockReader> reader = DummyBlockReader.pooled()) {
|
||||
for (Biome biome : deserts) {
|
||||
BlockState top = biome.getSurfaceConfig().getTopMaterial();
|
||||
MaterialColor color = top.getTopMaterialColor(reader.getValue().set(top), BlockPos.ORIGIN);
|
||||
int whiteDist2 = distance2(color, MaterialColor.SAND);
|
||||
int redDist2 = distance2(color, MaterialColor.ORANGE);
|
||||
if (whiteDist2 < redDist2) {
|
||||
white.add(biome);
|
||||
} else {
|
||||
red.add(biome);
|
||||
}
|
||||
}
|
||||
}
|
||||
this.layerManager = materials.getLayerManager();
|
||||
this.whiteSand = new ArrayList<>(white);
|
||||
this.redSand = new ArrayList<>(red);
|
||||
this.whites = new HashSet<>(white);
|
||||
this.reds = new HashSet<>(red);
|
||||
this.whiteSand.sort(Comparator.comparing(BiomeHelper::getId));
|
||||
this.redSand.sort(Comparator.comparing(BiomeHelper::getId));
|
||||
this.maxRedIndex = red.size() - 1;
|
||||
this.maxWhiteIndex = white.size() - 1;
|
||||
}
|
||||
|
||||
public boolean isRedDesert(Biome biome) {
|
||||
return reds.contains(biome);
|
||||
}
|
||||
|
||||
public boolean isWhiteDesert(Biome biome) {
|
||||
return whites.contains(biome);
|
||||
}
|
||||
|
||||
public Biome getRedDesert(float shape) {
|
||||
return ListUtils.get(redSand, maxRedIndex, shape, Biomes.MODIFIED_BADLANDS_PLATEAU);
|
||||
}
|
||||
|
||||
public Biome getWhiteDesert(float shape) {
|
||||
return ListUtils.get(whiteSand, maxWhiteIndex, shape, Biomes.DESERT);
|
||||
}
|
||||
|
||||
public LayerMaterial getSandLayers(Biome biome) {
|
||||
Block top = biome.getSurfaceConfig().getTopMaterial().getBlock();
|
||||
return layerManager.getMaterial(Blocks.SAND);
|
||||
}
|
||||
|
||||
private static int distance2(MaterialColor mc1, MaterialColor mc2) {
|
||||
Color c1 = new Color(mc1.color);
|
||||
Color c2 = new Color(mc2.color);
|
||||
int dr = c1.getRed() - c2.getRed();
|
||||
int dg = c1.getGreen() - c2.getGreen();
|
||||
int db = c1.getBlue() - c2.getBlue();
|
||||
return (dr * dr) + (dg * dg) + (db * db);
|
||||
}
|
||||
}
|
@ -0,0 +1,52 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.biome.provider;
|
||||
|
||||
import net.minecraft.util.Identifier;
|
||||
import net.minecraft.util.registry.Registry;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
|
||||
import java.util.Collection;
|
||||
import java.util.Optional;
|
||||
import java.util.function.Predicate;
|
||||
import java.util.stream.Collectors;
|
||||
|
||||
public class TerraBiomeRegistry {
|
||||
|
||||
private static final TerraBiomeRegistry instance = new TerraBiomeRegistry();
|
||||
|
||||
public Optional<Biome> getBiome(Identifier name) {
|
||||
return Registry.BIOME.getOrEmpty(name);
|
||||
}
|
||||
|
||||
public Collection<Biome> getAll(Predicate<Biome> filter) {
|
||||
return Registry.BIOME.stream().filter(filter.negate()).collect(Collectors.toList());
|
||||
}
|
||||
|
||||
public static TerraBiomeRegistry getInstance() {
|
||||
return instance;
|
||||
}
|
||||
}
|
@ -0,0 +1,70 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.biome.tag;
|
||||
|
||||
import com.terraforged.api.biome.BiomeTags;
|
||||
import com.terraforged.mod.Log;
|
||||
import net.fabricmc.fabric.api.resource.IdentifiableResourceReloadListener;
|
||||
import net.minecraft.resource.ResourceManager;
|
||||
import net.minecraft.tag.TagContainer;
|
||||
import net.minecraft.util.Identifier;
|
||||
import net.minecraft.util.profiler.Profiler;
|
||||
import net.minecraft.util.registry.Registry;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
|
||||
import java.util.concurrent.CompletableFuture;
|
||||
import java.util.concurrent.Executor;
|
||||
|
||||
public class BiomeTagManager implements IdentifiableResourceReloadListener {
|
||||
|
||||
private static final boolean RETAIN_ORDER = false;
|
||||
private static final Identifier ID = new Identifier("terraforged", "biome_tags");
|
||||
|
||||
private final TagContainer<Biome> collection = new TagContainer<>(
|
||||
Registry.BIOME::getOrEmpty,
|
||||
"tags/biomes",
|
||||
BiomeTagManager.RETAIN_ORDER,
|
||||
"biomes"
|
||||
);
|
||||
|
||||
@Override
|
||||
public Identifier getFabricId() {
|
||||
return ID;
|
||||
}
|
||||
|
||||
@Override
|
||||
public CompletableFuture<Void> reload(Synchronizer synchronizer, ResourceManager manager, Profiler prepareProfiler, Profiler applyProfiler,
|
||||
Executor prepareExecutor, Executor applyExecutor) {
|
||||
Log.debug("Reloading biome tag collection");
|
||||
return collection.prepareReload(manager, prepareExecutor)
|
||||
.thenCompose(synchronizer::whenPrepared)
|
||||
.thenAcceptAsync(collection::applyReload, applyExecutor)
|
||||
.thenRun(() -> {
|
||||
BiomeTags.setCollection(collection);
|
||||
Log.debug("Biome tag reload complete");
|
||||
});
|
||||
}
|
||||
}
|
@ -0,0 +1,35 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.chunk;
|
||||
|
||||
import com.terraforged.mod.biome.provider.BiomeProvider;
|
||||
import net.minecraft.world.gen.chunk.ChunkGenerator;
|
||||
import net.minecraft.world.gen.chunk.OverworldChunkGeneratorConfig;
|
||||
|
||||
public interface ChunkGeneratorFactory<T extends ChunkGenerator<?>> {
|
||||
|
||||
T create(TerraContext context, BiomeProvider biomeProvider, OverworldChunkGeneratorConfig settings);
|
||||
}
|
@ -0,0 +1,12 @@
|
||||
package com.terraforged.mod.chunk;
|
||||
|
||||
import com.terraforged.api.chunk.column.DecoratorContext;
|
||||
import com.terraforged.core.region.chunk.ChunkReader;
|
||||
import net.minecraft.util.math.ChunkPos;
|
||||
|
||||
public interface ChunkProcessor {
|
||||
|
||||
void preProcess(ChunkPos pos, ChunkReader chunk, TerraBiomeArray container);
|
||||
|
||||
void postProcess(ChunkReader chunk, TerraBiomeArray container, DecoratorContext context);
|
||||
}
|
@ -0,0 +1,128 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.chunk;
|
||||
|
||||
import net.minecraft.entity.EntityCategory;
|
||||
import net.minecraft.server.world.ServerWorld;
|
||||
import net.minecraft.util.math.BlockPos;
|
||||
import net.minecraft.util.math.noise.NoiseSampler;
|
||||
import net.minecraft.util.math.noise.OctavePerlinNoiseSampler;
|
||||
import net.minecraft.village.ZombieSiegeManager;
|
||||
import net.minecraft.world.ChunkRegion;
|
||||
import net.minecraft.world.Heightmap;
|
||||
import net.minecraft.world.IWorld;
|
||||
import net.minecraft.world.SpawnHelper;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
import net.minecraft.world.biome.source.BiomeSource;
|
||||
import net.minecraft.world.chunk.Chunk;
|
||||
import net.minecraft.world.gen.CatSpawner;
|
||||
import net.minecraft.world.gen.ChunkRandom;
|
||||
import net.minecraft.world.gen.PhantomSpawner;
|
||||
import net.minecraft.world.gen.PillagerSpawner;
|
||||
import net.minecraft.world.gen.chunk.ChunkGenerator;
|
||||
import net.minecraft.world.gen.chunk.ChunkGeneratorConfig;
|
||||
import net.minecraft.world.gen.feature.Feature;
|
||||
|
||||
import java.util.List;
|
||||
|
||||
public abstract class ObfHelperChunkGenerator<T extends ChunkGeneratorConfig> extends ChunkGenerator<T> {
|
||||
|
||||
private final CatSpawner catSpawner = new CatSpawner();
|
||||
private final PillagerSpawner patrolSpawner = new PillagerSpawner();
|
||||
private final PhantomSpawner phantomSpawner = new PhantomSpawner();
|
||||
private final ZombieSiegeManager zombieSiegeManager = new ZombieSiegeManager();
|
||||
private final NoiseSampler surfaceNoise;
|
||||
|
||||
public ObfHelperChunkGenerator(IWorld world, BiomeSource biomeSource, T settings) {
|
||||
super(world, biomeSource, settings);
|
||||
ChunkRandom random = new ChunkRandom(world.getSeed());
|
||||
this.surfaceNoise = new OctavePerlinNoiseSampler(random, 3, 0);
|
||||
}
|
||||
|
||||
@Override
|
||||
public List<Biome.SpawnEntry> getEntitySpawnList(EntityCategory category, BlockPos pos) {
|
||||
if (Feature.SWAMP_HUT.method_14029(this.world, pos)) {
|
||||
if (category == EntityCategory.MONSTER) {
|
||||
return Feature.SWAMP_HUT.getMonsterSpawns();
|
||||
}
|
||||
|
||||
if (category == EntityCategory.CREATURE) {
|
||||
return Feature.SWAMP_HUT.getCreatureSpawns();
|
||||
}
|
||||
} else if (category == EntityCategory.MONSTER) {
|
||||
if (Feature.PILLAGER_OUTPOST.isInsideStructure(this.world, pos)) {
|
||||
return Feature.PILLAGER_OUTPOST.getMonsterSpawns();
|
||||
}
|
||||
|
||||
if (Feature.OCEAN_MONUMENT.isInsideStructure(this.world, pos)) {
|
||||
return Feature.OCEAN_MONUMENT.getMonsterSpawns();
|
||||
}
|
||||
}
|
||||
return super.getEntitySpawnList(category, pos);
|
||||
}
|
||||
|
||||
@Override
|
||||
public void spawnEntities(ServerWorld worldIn, boolean spawnHostileMobs, boolean spawnPeacefulMobs) {
|
||||
phantomSpawner.spawn(worldIn, spawnHostileMobs, spawnPeacefulMobs);
|
||||
patrolSpawner.spawn(worldIn, spawnHostileMobs, spawnPeacefulMobs);
|
||||
catSpawner.spawn(worldIn, spawnHostileMobs, spawnPeacefulMobs);
|
||||
zombieSiegeManager.spawn(worldIn, spawnHostileMobs, spawnPeacefulMobs);
|
||||
}
|
||||
|
||||
@Override
|
||||
public void populateEntities(ChunkRegion region) {
|
||||
int chunkX = region.getCenterChunkX();
|
||||
int chunkZ = region.getCenterChunkZ();
|
||||
Biome biome = region.getChunk(chunkX, chunkZ).getBiomeArray().getBiomeForNoiseGen(0, 0, 0);
|
||||
ChunkRandom random = new ChunkRandom();
|
||||
random.setSeed(region.getSeed(), chunkX << 4, chunkZ << 4);
|
||||
SpawnHelper.populateEntities(region, biome, chunkX, chunkZ, random);
|
||||
}
|
||||
|
||||
@Override
|
||||
public final int getHeightInGround(int x, int z, Heightmap.Type type) {
|
||||
int level = sampleHeight(x, z, type) + 1;
|
||||
if (type == Heightmap.Type.OCEAN_FLOOR || type == Heightmap.Type.OCEAN_FLOOR_WG) {
|
||||
return level;
|
||||
}
|
||||
return Math.max(getSeaLevel(), level);
|
||||
}
|
||||
|
||||
public final double getSurfaceNoise(int x, int z) {
|
||||
double scale = 0.0625D;
|
||||
double noiseX = x * scale;
|
||||
double noiseZ = z * scale;
|
||||
double unusedValue1 = scale;
|
||||
double unusedValue2 = (x & 15) * scale;
|
||||
return surfaceNoise.sample(noiseX, noiseZ, unusedValue1, unusedValue2);
|
||||
}
|
||||
|
||||
public abstract int sampleHeight(int x, int z, Heightmap.Type type);
|
||||
|
||||
public abstract void populateNoise(IWorld world, Chunk chunk);
|
||||
|
||||
public abstract void buildSurface(ChunkRegion world, Chunk chunk);
|
||||
}
|
@ -0,0 +1,127 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.chunk;
|
||||
|
||||
import com.terraforged.core.cell.Cell;
|
||||
import com.terraforged.core.region.chunk.ChunkReader;
|
||||
import com.terraforged.core.util.PosIterator;
|
||||
import com.terraforged.core.world.terrain.Terrain;
|
||||
import net.minecraft.util.math.MathHelper;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
import net.minecraft.world.biome.source.BiomeArray;
|
||||
|
||||
// holds a 1:1 map of biomes in the chunk
|
||||
// also holds the chunk's view on the heightmap for convenience
|
||||
public class TerraBiomeArray extends BiomeArray {
|
||||
|
||||
private static final int BITS_WIDTH = (int) Math.round(Math.log(16.0D) / Math.log(2.0D)) - 2;
|
||||
private static final int ZOOM_VERT = (int) Math.round(Math.log(256.0D) / Math.log(2.0D)) - 2;
|
||||
public static final int SIZE = 1 << BITS_WIDTH + BITS_WIDTH + ZOOM_VERT;
|
||||
public static final int MASK_HORIZ = (1 << BITS_WIDTH) - 1;
|
||||
public static final int MASK_VERT = (1 << ZOOM_VERT) - 1;
|
||||
|
||||
private final Biome[] biomes;
|
||||
private final Biome[] surface;
|
||||
private final ChunkReader chunkReader;
|
||||
|
||||
public TerraBiomeArray(Builder builder, ChunkReader chunkReader) {
|
||||
super(builder.biomes);
|
||||
this.chunkReader = chunkReader;
|
||||
this.biomes = builder.biomes;
|
||||
this.surface = builder.surfaceBiomeCache;
|
||||
}
|
||||
|
||||
public Biome getBiome(int x, int z) {
|
||||
return surface[indexOf(x, z)];
|
||||
}
|
||||
|
||||
@Override
|
||||
public Biome getBiomeForNoiseGen(int x, int y, int z) {
|
||||
return super.getBiomeForNoiseGen(x, y, z);
|
||||
}
|
||||
|
||||
public Biome getFeatureBiome() {
|
||||
PosIterator iterator = PosIterator.area(0, 0, 16, 16);
|
||||
while (iterator.next()) {
|
||||
Cell<Terrain> cell = chunkReader.getCell(iterator.x(), iterator.z());
|
||||
if (cell.biomeType.isExtreme()) {
|
||||
return getBiome(iterator.x(), iterator.z());
|
||||
}
|
||||
}
|
||||
return getBiome(8, 8);
|
||||
}
|
||||
|
||||
public BiomeArray bakeBiomes() {
|
||||
return new BiomeArray(biomes);
|
||||
}
|
||||
|
||||
public ChunkReader getChunkReader() {
|
||||
return chunkReader;
|
||||
}
|
||||
|
||||
private static int indexOf(int x, int z) {
|
||||
x &= 15;
|
||||
z &= 15;
|
||||
return (z << 4) + x;
|
||||
}
|
||||
|
||||
private static int indexOf(int x, int y, int z) {
|
||||
x &= MASK_HORIZ;
|
||||
y = MathHelper.clamp(y, 0, MASK_VERT);
|
||||
z &= MASK_HORIZ;
|
||||
return y << BITS_WIDTH + BITS_WIDTH | z << BITS_WIDTH | x;
|
||||
}
|
||||
|
||||
public static Builder builder() {
|
||||
return new Builder();
|
||||
}
|
||||
|
||||
public static class Builder {
|
||||
|
||||
private final Biome[] biomes = new Biome[SIZE];
|
||||
private final Biome[] surfaceBiomeCache = new Biome[256];
|
||||
|
||||
public void set(int x, int z, Biome biome) {
|
||||
surfaceBiomeCache[indexOf(x, z)] = biome;
|
||||
}
|
||||
|
||||
public TerraBiomeArray build(ChunkReader chunkReader) {
|
||||
// biome storage format is 1 biome pos == 4x4x4 blocks, stored in an 4x64x4 (xyz) array
|
||||
// sample the 1:1 surfaceBiomeCache every 4 blocks with a 2 block offset (roughly center of the 4x4 area)
|
||||
for (int dy = 0; dy < 64; dy++) {
|
||||
for (int dz = 0; dz < 4; dz++) {
|
||||
for (int dx = 0; dx < 4; dx++) {
|
||||
int x = dx * 4;
|
||||
int z = dz * 4;
|
||||
int index = indexOf(dx, dy, dz);
|
||||
biomes[index] = surfaceBiomeCache[indexOf(x, z)];
|
||||
}
|
||||
}
|
||||
}
|
||||
return new TerraBiomeArray(this, chunkReader);
|
||||
}
|
||||
}
|
||||
}
|
@ -0,0 +1,385 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.chunk;
|
||||
|
||||
import com.terraforged.api.chunk.column.ColumnDecorator;
|
||||
import com.terraforged.api.chunk.column.DecoratorContext;
|
||||
import com.terraforged.api.chunk.surface.ChunkSurfaceBuffer;
|
||||
import com.terraforged.api.chunk.surface.SurfaceContext;
|
||||
import com.terraforged.api.chunk.surface.SurfaceManager;
|
||||
import com.terraforged.api.material.layer.LayerManager;
|
||||
import com.terraforged.core.cell.Cell;
|
||||
import com.terraforged.core.region.RegionCache;
|
||||
import com.terraforged.core.region.RegionGenerator;
|
||||
import com.terraforged.core.region.Size;
|
||||
import com.terraforged.core.region.chunk.ChunkReader;
|
||||
import com.terraforged.core.util.concurrent.ThreadPool;
|
||||
import com.terraforged.core.world.decorator.Decorator;
|
||||
import com.terraforged.feature.FeatureManager;
|
||||
import com.terraforged.feature.matcher.dynamic.DynamicMatcher;
|
||||
import com.terraforged.feature.matcher.feature.FeatureMatcher;
|
||||
import com.terraforged.feature.modifier.FeatureModifierLoader;
|
||||
import com.terraforged.feature.modifier.FeatureModifiers;
|
||||
import com.terraforged.feature.predicate.DeepWater;
|
||||
import com.terraforged.feature.predicate.FeaturePredicate;
|
||||
import com.terraforged.feature.predicate.MinHeight;
|
||||
import com.terraforged.feature.template.type.FeatureTypes;
|
||||
import com.terraforged.mod.Log;
|
||||
import com.terraforged.mod.biome.provider.BiomeProvider;
|
||||
import com.terraforged.mod.chunk.fix.ChunkCarverFix;
|
||||
import com.terraforged.mod.chunk.fix.RegionFix;
|
||||
import com.terraforged.mod.decorator.ChunkPopulator;
|
||||
import com.terraforged.mod.decorator.base.BedrockDecorator;
|
||||
import com.terraforged.mod.decorator.base.CoastDecorator;
|
||||
import com.terraforged.mod.decorator.base.ErosionDecorator;
|
||||
import com.terraforged.mod.decorator.base.GeologyDecorator;
|
||||
import com.terraforged.mod.decorator.feature.LayerDecorator;
|
||||
import com.terraforged.mod.decorator.feature.SnowEroder;
|
||||
import com.terraforged.mod.decorator.surface.FrozenOcean;
|
||||
import com.terraforged.mod.feature.Matchers;
|
||||
import com.terraforged.mod.feature.TerrainHelper;
|
||||
import com.terraforged.mod.feature.predicate.TreeLine;
|
||||
import com.terraforged.mod.material.Materials;
|
||||
import com.terraforged.mod.material.geology.GeoManager;
|
||||
import com.terraforged.mod.util.setup.SetupHooks;
|
||||
import net.minecraft.structure.StructureManager;
|
||||
import net.minecraft.util.math.BlockPos;
|
||||
import net.minecraft.util.math.ChunkPos;
|
||||
import net.minecraft.world.ChunkRegion;
|
||||
import net.minecraft.world.Heightmap;
|
||||
import net.minecraft.world.IWorld;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
import net.minecraft.world.biome.Biomes;
|
||||
import net.minecraft.world.biome.source.BiomeAccess;
|
||||
import net.minecraft.world.chunk.Chunk;
|
||||
import net.minecraft.world.chunk.ProtoChunk;
|
||||
import net.minecraft.world.gen.GenerationStep;
|
||||
import net.minecraft.world.gen.chunk.ChunkGenerator;
|
||||
import net.minecraft.world.gen.chunk.ChunkGeneratorConfig;
|
||||
import net.minecraft.world.gen.feature.AbstractTreeFeature;
|
||||
import net.minecraft.world.gen.feature.Feature;
|
||||
|
||||
import java.util.ArrayList;
|
||||
import java.util.List;
|
||||
|
||||
public class TerraChunkGenerator extends ObfHelperChunkGenerator<ChunkGeneratorConfig> implements ChunkProcessor {
|
||||
|
||||
private final TerraContext context;
|
||||
private final BiomeProvider biomeProvider;
|
||||
private final TerrainHelper terrainHelper;
|
||||
|
||||
private final GeoManager geologyManager;
|
||||
private final FeatureManager featureManager;
|
||||
private final SurfaceManager surfaceManager;
|
||||
private final List<ColumnDecorator> baseDecorators;
|
||||
private final List<ColumnDecorator> postProcessors;
|
||||
|
||||
private final RegionCache regionCache;
|
||||
|
||||
public TerraChunkGenerator(TerraContext context, BiomeProvider biomeProvider, ChunkGeneratorConfig settings) {
|
||||
super(context.world, biomeProvider, settings);
|
||||
this.context = context;
|
||||
this.biomeProvider = biomeProvider;
|
||||
this.surfaceManager = SetupHooks.setup(createSurfaceManager(), context.copy());
|
||||
this.geologyManager = SetupHooks.setup(createGeologyManager(context), context.copy());
|
||||
this.baseDecorators = createBaseDecorators(context);
|
||||
this.postProcessors = createFeatureDecorators(context);
|
||||
this.terrainHelper = new TerrainHelper((int) world.getSeed(), 0.8F);
|
||||
this.featureManager = createFeatureManager(context);
|
||||
this.regionCache = createRegionCache(context);
|
||||
SetupHooks.setup(getLayerManager(), context.copy());
|
||||
SetupHooks.setup(baseDecorators, postProcessors, context.copy());
|
||||
}
|
||||
|
||||
@Override
|
||||
public void setStructureStarts(BiomeAccess biomeAccess, Chunk chunk, ChunkGenerator<?> chunkGenerator, StructureManager structureManager) {
|
||||
super.setStructureStarts(biomeAccess, chunk, this, structureManager);
|
||||
}
|
||||
|
||||
@Override
|
||||
public final void populateBiomes(Chunk chunk) {
|
||||
ChunkPos pos = chunk.getPos();
|
||||
ChunkReader reader = getChunkReader(pos.x, pos.z);
|
||||
TerraBiomeArray container = getBiomeSource().createBiomeContainer(reader);
|
||||
((ProtoChunk) chunk).method_22405(container);
|
||||
// apply chunk-local heightmap modifications
|
||||
preProcess(pos, reader, container);
|
||||
}
|
||||
|
||||
@Override
|
||||
public final void preProcess(ChunkPos pos, ChunkReader chunk, TerraBiomeArray container) {
|
||||
chunk.iterate((cell, dx, dz) -> {
|
||||
Biome biome = container.getBiome(dx, dz);
|
||||
for (Decorator decorator : getBiomeSource().getDecorators(biome)) {
|
||||
if (decorator.apply(cell, pos.getStartX() + dx, pos.getStartZ() + dz)) {
|
||||
return;
|
||||
}
|
||||
}
|
||||
});
|
||||
}
|
||||
|
||||
@Override
|
||||
public final void populateNoise(IWorld world, Chunk chunk) {
|
||||
DecoratorContext context = new DecoratorContext(chunk, getContext().levels, getContext().terrain, getContext().factory.getClimate());
|
||||
TerraBiomeArray container = getBiomeContainer(chunk);
|
||||
container.getChunkReader().iterate((cell, dx, dz) -> {
|
||||
int px = context.blockX + dx;
|
||||
int pz = context.blockZ + dz;
|
||||
int py = (int) (cell.value * getMaxY());
|
||||
context.cell = cell;
|
||||
context.biome = container.getBiome(dx, dz);
|
||||
ChunkPopulator.INSTANCE.decorate(chunk, context, px, py, pz);
|
||||
});
|
||||
terrainHelper.flatten(world, chunk, context.blockX, context.blockZ);
|
||||
}
|
||||
|
||||
@Override
|
||||
public final void buildSurface(ChunkRegion world, Chunk chunk) {
|
||||
ChunkSurfaceBuffer buffer = new ChunkSurfaceBuffer(chunk);
|
||||
SurfaceContext context = getContext().surface(buffer, getConfig());
|
||||
TerraBiomeArray container = getBiomeContainer(chunk);
|
||||
container.getChunkReader().iterate((cell, dx, dz) -> {
|
||||
int px = context.blockX + dx;
|
||||
int pz = context.blockZ + dz;
|
||||
int top = chunk.sampleHeightmap(Heightmap.Type.WORLD_SURFACE_WG, dx, dz) + 1;
|
||||
|
||||
buffer.setSurfaceLevel(top);
|
||||
|
||||
context.cell = cell;
|
||||
context.biome = container.getBiome(dx, dz);
|
||||
context.noise = getSurfaceNoise(px, pz) * 15D;
|
||||
|
||||
getSurfaceManager().getSurface(context).buildSurface(px, pz, top, context);
|
||||
|
||||
int py = (int) (cell.value * getMaxY());
|
||||
for (ColumnDecorator processor : getBaseDecorators()) {
|
||||
processor.decorate(buffer, context, px, py, pz);
|
||||
}
|
||||
});
|
||||
}
|
||||
|
||||
@Override
|
||||
public void carve(BiomeAccess biomeAccess, Chunk chunk, GenerationStep.Carver carver) {
|
||||
super.carve(biomeAccess, new ChunkCarverFix(chunk, context.materials), carver);
|
||||
}
|
||||
|
||||
@Override
|
||||
public void generateFeatures(ChunkRegion region) {
|
||||
int chunkX = region.getCenterChunkX();
|
||||
int chunkZ = region.getCenterChunkZ();
|
||||
Chunk chunk = region.getChunk(chunkX, chunkZ);
|
||||
TerraBiomeArray container = getBiomeContainer(chunk);
|
||||
Biome biome = container.getFeatureBiome();
|
||||
DecoratorContext context = getContext().decorator(chunk);
|
||||
|
||||
IWorld regionFix = new RegionFix(region, this);
|
||||
BlockPos pos = new BlockPos(context.blockX, 0, context.blockZ);
|
||||
|
||||
// place biome features
|
||||
featureManager.decorate(this, regionFix, chunk, biome, pos);
|
||||
|
||||
// run post processes on chunk
|
||||
postProcess(container.getChunkReader(), container, context);
|
||||
|
||||
// bake biome array & discard gen data
|
||||
((ProtoChunk) chunk).method_22405(container.bakeBiomes());
|
||||
}
|
||||
|
||||
@Override
|
||||
public final void postProcess(ChunkReader chunk, TerraBiomeArray container, DecoratorContext context) {
|
||||
chunk.iterate((cell, dx, dz) -> {
|
||||
int px = context.blockX + dx;
|
||||
int pz = context.blockZ + dz;
|
||||
int py = context.chunk.sampleHeightmap(Heightmap.Type.WORLD_SURFACE_WG, dx, dz);
|
||||
context.cell = cell;
|
||||
context.biome = container.getBiome(dx, dz);
|
||||
for (ColumnDecorator decorator : getPostProcessors()) {
|
||||
decorator.decorate(context.chunk, context, px, py, pz);
|
||||
}
|
||||
});
|
||||
}
|
||||
|
||||
@Override
|
||||
public int getHeightOnGround(int x, int z, Heightmap.Type heightmapType) {
|
||||
return this.sampleHeight(x, z, heightmapType); // todo may be wrong
|
||||
}
|
||||
|
||||
@Override
|
||||
public int sampleHeight(int x, int z, Heightmap.Type type) {
|
||||
int chunkX = Size.blockToChunk(x);
|
||||
int chunkZ = Size.blockToChunk(z);
|
||||
ChunkReader chunk = getChunkReader(chunkX, chunkZ);
|
||||
Cell<?> cell = chunk.getCell(x, z);
|
||||
return (int) (cell.value * getMaxY());
|
||||
}
|
||||
|
||||
@Override
|
||||
public BiomeProvider getBiomeSource() {
|
||||
return biomeProvider;
|
||||
}
|
||||
|
||||
@Override
|
||||
public final int getMaxY() {
|
||||
return getContext().levels.worldHeight;
|
||||
}
|
||||
|
||||
@Override
|
||||
public final int getSeaLevel() {
|
||||
return getContext().levels.waterLevel;
|
||||
}
|
||||
|
||||
@Override
|
||||
public int getSpawnHeight() {
|
||||
return getContext().levels.groundLevel;
|
||||
}
|
||||
|
||||
public final TerraContext getContext() {
|
||||
return context;
|
||||
}
|
||||
|
||||
public final Materials getMaterials() {
|
||||
return context.materials;
|
||||
}
|
||||
|
||||
public final GeoManager getGeologyManager() {
|
||||
return geologyManager;
|
||||
}
|
||||
|
||||
public final LayerManager getLayerManager() {
|
||||
return context.materials.getLayerManager();
|
||||
}
|
||||
|
||||
public final SurfaceManager getSurfaceManager() {
|
||||
return surfaceManager;
|
||||
}
|
||||
|
||||
public final List<ColumnDecorator> getBaseDecorators() {
|
||||
return baseDecorators;
|
||||
}
|
||||
|
||||
public final List<ColumnDecorator> getPostProcessors() {
|
||||
return postProcessors;
|
||||
}
|
||||
|
||||
protected TerraBiomeArray getBiomeContainer(Chunk chunk) {
|
||||
if (chunk.getBiomeArray() instanceof TerraBiomeArray) {
|
||||
return (TerraBiomeArray) chunk.getBiomeArray();
|
||||
}
|
||||
|
||||
ChunkReader view = getChunkReader(chunk.getPos().x, chunk.getPos().z);
|
||||
TerraBiomeArray container = getBiomeSource().createBiomeContainer(view);
|
||||
if (chunk instanceof ProtoChunk) {
|
||||
((ProtoChunk) chunk).method_22405(container);
|
||||
}
|
||||
|
||||
return container;
|
||||
}
|
||||
|
||||
protected FeatureManager createFeatureManager(TerraContext context) {
|
||||
FeatureModifiers modifiers;
|
||||
if (context.terraSettings.features.customBiomeFeatures) {
|
||||
Log.info(" - Custom biome features enabled");
|
||||
modifiers = FeatureModifierLoader.load();
|
||||
} else {
|
||||
modifiers = new FeatureModifiers();
|
||||
}
|
||||
|
||||
// block ugly features
|
||||
modifiers.getPredicates().add(Matchers.STONE_BLOBS, FeaturePredicate.DENY);
|
||||
modifiers.getPredicates().add(FeatureMatcher.of(Feature.DISK), FeaturePredicate.DENY);
|
||||
modifiers.getPredicates().add(FeatureMatcher.of(Feature.LAKE), FeaturePredicate.DENY);
|
||||
modifiers.getPredicates().add(FeatureMatcher.of(Feature.SPRING_FEATURE), FeaturePredicate.DENY);
|
||||
|
||||
// limit to deep oceans
|
||||
modifiers.getPredicates().add(FeatureMatcher.of(Feature.SHIPWRECK), DeepWater.INSTANCE);
|
||||
modifiers.getPredicates().add(FeatureMatcher.of(Feature.OCEAN_RUIN), DeepWater.INSTANCE);
|
||||
modifiers.getPredicates().add(FeatureMatcher.of(Feature.OCEAN_MONUMENT), DeepWater.INSTANCE);
|
||||
|
||||
// prevent mineshafts above ground
|
||||
modifiers.getPredicates().add(FeatureMatcher.of(Feature.MINESHAFT), MinHeight.HEIGHT80);
|
||||
|
||||
// prevent trees/bamboo growing too high up
|
||||
TreeLine treeLine = new TreeLine(context);
|
||||
modifiers.getPredicates().add(FeatureTypes.TREE.matcher(), treeLine);
|
||||
modifiers.getPredicates().add(FeatureMatcher.of(Feature.BAMBOO), treeLine);
|
||||
modifiers.getDynamic().add(DynamicMatcher.feature(AbstractTreeFeature.class), treeLine);
|
||||
|
||||
return FeatureManager.create(context.world, SetupHooks.setup(modifiers, context.copy()));
|
||||
}
|
||||
|
||||
protected GeoManager createGeologyManager(TerraContext context) {
|
||||
return new GeoManager(context);
|
||||
}
|
||||
|
||||
protected SurfaceManager createSurfaceManager() {
|
||||
SurfaceManager manager = new SurfaceManager();
|
||||
manager.replace(Biomes.FROZEN_OCEAN, new FrozenOcean(context, 20, 15));
|
||||
manager.replace(Biomes.DEEP_FROZEN_OCEAN, new FrozenOcean(context, 30, 30));
|
||||
return manager;
|
||||
}
|
||||
|
||||
protected List<ColumnDecorator> createBaseDecorators(TerraContext context) {
|
||||
List<ColumnDecorator> processors = new ArrayList<>();
|
||||
if (context.terraSettings.features.strataDecorator) {
|
||||
Log.info(" - Geology decorator enabled");
|
||||
processors.add(new GeologyDecorator(geologyManager));
|
||||
}
|
||||
if (context.terraSettings.features.erosionDecorator) {
|
||||
Log.info(" - Erosion decorator enabled");
|
||||
processors.add(new ErosionDecorator(context));
|
||||
}
|
||||
processors.add(new CoastDecorator(context));
|
||||
processors.add(new BedrockDecorator());
|
||||
return processors;
|
||||
}
|
||||
|
||||
protected List<ColumnDecorator> createFeatureDecorators(TerraContext context) {
|
||||
List<ColumnDecorator> processors = new ArrayList<>();
|
||||
if (context.terraSettings.features.naturalSnowDecorator) {
|
||||
Log.info(" - Natural snow decorator enabled");
|
||||
processors.add(new SnowEroder(context));
|
||||
}
|
||||
if (context.terraSettings.features.smoothLayerDecorator) {
|
||||
Log.info(" - Smooth layer decorator enabled");
|
||||
processors.add(new LayerDecorator(context.materials.getLayerManager()));
|
||||
}
|
||||
return processors;
|
||||
}
|
||||
|
||||
protected RegionCache createRegionCache(TerraContext context) {
|
||||
return RegionGenerator.builder()
|
||||
.legacy(context.terraSettings.version == 0)
|
||||
.pool(ThreadPool.getFixed())
|
||||
.factory(context.factory)
|
||||
.size(3, 2)
|
||||
.build()
|
||||
.toCache();
|
||||
}
|
||||
|
||||
public ChunkReader getChunkReader(int chunkX, int chunkZ) {
|
||||
return regionCache.getChunk(chunkX, chunkZ);
|
||||
}
|
||||
}
|
@ -0,0 +1,68 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.chunk;
|
||||
|
||||
import com.terraforged.api.chunk.column.DecoratorContext;
|
||||
import com.terraforged.api.chunk.surface.SurfaceContext;
|
||||
import com.terraforged.core.world.GeneratorContext;
|
||||
import com.terraforged.core.world.WorldGeneratorFactory;
|
||||
import com.terraforged.core.world.heightmap.Heightmap;
|
||||
import com.terraforged.core.world.terrain.Terrains;
|
||||
import com.terraforged.mod.material.Materials;
|
||||
import com.terraforged.mod.settings.TerraSettings;
|
||||
import net.minecraft.item.ItemStack;
|
||||
import net.minecraft.item.Items;
|
||||
import net.minecraft.world.IWorld;
|
||||
import net.minecraft.world.chunk.Chunk;
|
||||
import net.minecraft.world.gen.chunk.ChunkGeneratorConfig;
|
||||
|
||||
public class TerraContext extends GeneratorContext {
|
||||
|
||||
public final IWorld world;
|
||||
public final Heightmap heightmap;
|
||||
public final Materials materials;
|
||||
public final WorldGeneratorFactory factory;
|
||||
public final TerraSettings terraSettings;
|
||||
|
||||
public TerraContext(IWorld world, Terrains terrain, TerraSettings settings) {
|
||||
super(terrain, settings, TerraTerrainProvider::new);
|
||||
this.world = world;
|
||||
this.materials = new Materials();
|
||||
this.terraSettings = settings;
|
||||
this.factory = new WorldGeneratorFactory(this);
|
||||
this.heightmap = factory.getHeightmap();
|
||||
ItemStack stack = new ItemStack(Items.COBBLESTONE);
|
||||
stack.getMaxCount();
|
||||
}
|
||||
|
||||
public DecoratorContext decorator(Chunk chunk) {
|
||||
return new DecoratorContext(chunk, levels, terrain, factory.getClimate());
|
||||
}
|
||||
|
||||
public SurfaceContext surface(Chunk chunk, ChunkGeneratorConfig settings) {
|
||||
return new SurfaceContext(chunk, levels, terrain, factory.getClimate(), settings, world.getSeed());
|
||||
}
|
||||
}
|
@ -0,0 +1,41 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.chunk;
|
||||
|
||||
import com.terraforged.mod.settings.StructureSettings;
|
||||
import net.minecraft.world.gen.chunk.OverworldChunkGeneratorConfig;
|
||||
|
||||
public class TerraGenSettings extends OverworldChunkGeneratorConfig {
|
||||
|
||||
public TerraGenSettings(StructureSettings settings) {
|
||||
super.villageDistance *= settings.villageDistance;
|
||||
super.mansionDistance *= settings.mansionDistance;
|
||||
super.strongholdDistance *= settings.strongholdDistance;
|
||||
super.templeDistance *= settings.biomeStructureDistance;
|
||||
super.oceanMonumentSpacing *= settings.oceanMonumentSpacing;
|
||||
super.oceanMonumentSeparation *= settings.oceanMonumentSeparation;
|
||||
}
|
||||
}
|
@ -0,0 +1,45 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.chunk;
|
||||
|
||||
import com.terraforged.core.cell.Populator;
|
||||
import com.terraforged.core.world.GeneratorContext;
|
||||
import com.terraforged.core.world.heightmap.RegionConfig;
|
||||
import com.terraforged.core.world.terrain.provider.StandardTerrainProvider;
|
||||
import com.terraforged.mod.util.setup.SetupHooks;
|
||||
|
||||
public class TerraTerrainProvider extends StandardTerrainProvider {
|
||||
|
||||
public TerraTerrainProvider(GeneratorContext context, RegionConfig config, Populator defaultPopulator) {
|
||||
super(context, config, defaultPopulator);
|
||||
}
|
||||
|
||||
@Override
|
||||
public void init() {
|
||||
super.init();
|
||||
SetupHooks.setup(this, getContext().copy());
|
||||
}
|
||||
}
|
@ -0,0 +1,74 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.chunk.fix;
|
||||
|
||||
import com.terraforged.api.chunk.ChunkDelegate;
|
||||
import com.terraforged.api.material.state.States;
|
||||
import com.terraforged.mod.material.MaterialHelper;
|
||||
import com.terraforged.mod.material.Materials;
|
||||
import net.minecraft.block.BlockState;
|
||||
import net.minecraft.util.math.BlockPos;
|
||||
import net.minecraft.world.chunk.Chunk;
|
||||
|
||||
public class ChunkCarverFix implements ChunkDelegate {
|
||||
|
||||
private final Chunk delegate;
|
||||
private final Materials materials;
|
||||
|
||||
public ChunkCarverFix(Chunk chunk, Materials materials) {
|
||||
this.delegate = chunk;
|
||||
this.materials = materials;
|
||||
}
|
||||
|
||||
@Override
|
||||
public Chunk getDelegate() {
|
||||
return delegate;
|
||||
}
|
||||
|
||||
@Override
|
||||
public BlockState getBlockState(BlockPos pos) {
|
||||
BlockState state = getDelegate().getBlockState(pos);
|
||||
if (MaterialHelper.isAir(state.getBlock())) {
|
||||
return state;
|
||||
}
|
||||
if (MaterialHelper.isGrass(state.getBlock())) {
|
||||
return States.GRASS_BLOCK.get();
|
||||
}
|
||||
if (materials.isStone(state.getBlock())) {
|
||||
return States.STONE.get();
|
||||
}
|
||||
if (materials.isEarth(state.getBlock())) {
|
||||
return States.DIRT.get();
|
||||
}
|
||||
if (materials.isClay(state.getBlock())) {
|
||||
return States.DIRT.get();
|
||||
}
|
||||
if (materials.isSediment(state.getBlock())) {
|
||||
return States.SAND.get();
|
||||
}
|
||||
return state;
|
||||
}
|
||||
}
|
@ -0,0 +1,589 @@
|
||||
package com.terraforged.mod.chunk.fix;
|
||||
|
||||
import net.fabricmc.api.EnvType;
|
||||
import net.fabricmc.api.Environment;
|
||||
import net.minecraft.block.Block;
|
||||
import net.minecraft.block.BlockState;
|
||||
import net.minecraft.block.entity.BlockEntity;
|
||||
import net.minecraft.entity.Entity;
|
||||
import net.minecraft.entity.EntityContext;
|
||||
import net.minecraft.entity.LivingEntity;
|
||||
import net.minecraft.entity.ai.TargetPredicate;
|
||||
import net.minecraft.entity.player.PlayerEntity;
|
||||
import net.minecraft.fluid.Fluid;
|
||||
import net.minecraft.fluid.FluidState;
|
||||
import net.minecraft.particle.ParticleEffect;
|
||||
import net.minecraft.server.world.ServerWorld;
|
||||
import net.minecraft.sound.SoundCategory;
|
||||
import net.minecraft.sound.SoundEvent;
|
||||
import net.minecraft.util.hit.BlockHitResult;
|
||||
import net.minecraft.util.math.BlockPos;
|
||||
import net.minecraft.util.math.Box;
|
||||
import net.minecraft.util.math.Direction;
|
||||
import net.minecraft.util.math.Vec3d;
|
||||
import net.minecraft.util.shape.VoxelShape;
|
||||
import net.minecraft.world.BlockView;
|
||||
import net.minecraft.world.ChunkRegion;
|
||||
import net.minecraft.world.Difficulty;
|
||||
import net.minecraft.world.Heightmap;
|
||||
import net.minecraft.world.LightType;
|
||||
import net.minecraft.world.LocalDifficulty;
|
||||
import net.minecraft.world.RayTraceContext;
|
||||
import net.minecraft.world.TickScheduler;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
import net.minecraft.world.biome.source.BiomeAccess;
|
||||
import net.minecraft.world.border.WorldBorder;
|
||||
import net.minecraft.world.chunk.Chunk;
|
||||
import net.minecraft.world.chunk.ChunkManager;
|
||||
import net.minecraft.world.chunk.ChunkStatus;
|
||||
import net.minecraft.world.chunk.light.LightingProvider;
|
||||
import net.minecraft.world.dimension.Dimension;
|
||||
import net.minecraft.world.level.ColorResolver;
|
||||
import net.minecraft.world.level.LevelProperties;
|
||||
|
||||
import java.util.Collections;
|
||||
import java.util.List;
|
||||
import java.util.Random;
|
||||
import java.util.Set;
|
||||
import java.util.UUID;
|
||||
import java.util.function.Predicate;
|
||||
import java.util.stream.Stream;
|
||||
|
||||
public class RegionDelegate extends ChunkRegion {
|
||||
|
||||
protected final ChunkRegion region;
|
||||
|
||||
// ChunkRegion init notes:
|
||||
// - list of chunks passed in must be square (ie (sqrt(size) * sqrt(size) == size)
|
||||
// - list of chunks must not be empty due to: "list.get(list.size() / 2).getPos()"
|
||||
public RegionDelegate(ServerWorld world, ChunkRegion region) {
|
||||
super(world, Collections.singletonList(getMainChunk(region)));
|
||||
this.region = region;
|
||||
}
|
||||
|
||||
@Override
|
||||
public int getCenterChunkX() {
|
||||
return region.getCenterChunkX();
|
||||
}
|
||||
|
||||
@Override
|
||||
public int getCenterChunkZ() {
|
||||
return region.getCenterChunkZ();
|
||||
}
|
||||
|
||||
@Override
|
||||
public Chunk getChunk(int chunkX, int chunkZ) {
|
||||
return region.getChunk(chunkX, chunkZ);
|
||||
}
|
||||
|
||||
@Override
|
||||
|
||||
public Chunk getChunk(int x, int z, ChunkStatus requiredStatus, boolean nonnull) {
|
||||
return region.getChunk(x, z, requiredStatus, nonnull);
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean isChunkLoaded(int chunkX, int chunkZ) {
|
||||
return region.isChunkLoaded(chunkX, chunkZ);
|
||||
}
|
||||
|
||||
@Override
|
||||
public BlockState getBlockState(BlockPos pos) {
|
||||
return region.getBlockState(pos);
|
||||
}
|
||||
|
||||
@Override
|
||||
public FluidState getFluidState(BlockPos pos) {
|
||||
return region.getFluidState(pos);
|
||||
}
|
||||
|
||||
@Override
|
||||
|
||||
public PlayerEntity getClosestPlayer(double x, double y, double z, double distance, Predicate<Entity> predicate) {
|
||||
return region.getClosestPlayer(x, y, z, distance, predicate);
|
||||
}
|
||||
|
||||
@Override
|
||||
public int getAmbientDarkness() {
|
||||
return region.getAmbientDarkness();
|
||||
}
|
||||
|
||||
@Override
|
||||
public BiomeAccess getBiomeAccess() {
|
||||
return region.getBiomeAccess();
|
||||
}
|
||||
|
||||
@Override
|
||||
public Biome getBiomeForNoiseGen(int biomeX, int biomeY, int biomeZ) {
|
||||
return region.getBiomeForNoiseGen(biomeX, biomeY, biomeZ);
|
||||
}
|
||||
|
||||
|
||||
@Override
|
||||
public LightingProvider getLightingProvider() {
|
||||
return region.getLightingProvider();
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean breakBlock(BlockPos pos, boolean drop, Entity breakingEntity) {
|
||||
return region.breakBlock(pos, drop, breakingEntity);
|
||||
}
|
||||
|
||||
@Override
|
||||
public BlockEntity getBlockEntity(BlockPos pos) {
|
||||
return region.getBlockEntity(pos);
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean setBlockState(BlockPos pos, BlockState newState, int flags) {
|
||||
return region.setBlockState(pos, newState, flags);
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean spawnEntity(Entity entityIn) {
|
||||
return region.spawnEntity(entityIn);
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean removeBlock(BlockPos pos, boolean isMoving) {
|
||||
return region.removeBlock(pos, isMoving);
|
||||
}
|
||||
|
||||
@Override
|
||||
public WorldBorder getWorldBorder() {
|
||||
return region.getWorldBorder();
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean isClient() {
|
||||
return region.isClient();
|
||||
}
|
||||
|
||||
@Override
|
||||
@Deprecated
|
||||
public ServerWorld getWorld() {
|
||||
return region.getWorld();
|
||||
}
|
||||
|
||||
|
||||
@Override
|
||||
public LevelProperties getLevelProperties() {
|
||||
return region.getLevelProperties();
|
||||
}
|
||||
|
||||
@Override
|
||||
public LocalDifficulty getLocalDifficulty(BlockPos pos) {
|
||||
return region.getLocalDifficulty(pos);
|
||||
}
|
||||
|
||||
@Override
|
||||
public ChunkManager getChunkManager() {
|
||||
return region.getChunkManager();
|
||||
}
|
||||
|
||||
@Override
|
||||
public long getSeed() {
|
||||
return region.getSeed();
|
||||
}
|
||||
|
||||
@Override
|
||||
public TickScheduler<Block> getBlockTickScheduler() {
|
||||
return region.getBlockTickScheduler();
|
||||
}
|
||||
|
||||
@Override
|
||||
public TickScheduler<Fluid> getFluidTickScheduler() {
|
||||
return region.getFluidTickScheduler();
|
||||
}
|
||||
|
||||
@Override
|
||||
public int getSeaLevel() {
|
||||
return region.getSeaLevel();
|
||||
}
|
||||
|
||||
@Override
|
||||
public Random getRandom() {
|
||||
return region.getRandom();
|
||||
}
|
||||
|
||||
@Override
|
||||
public void updateNeighbors(BlockPos pos, Block blockIn) {
|
||||
region.updateNeighbors(pos, blockIn);
|
||||
}
|
||||
|
||||
@Override
|
||||
public int getTopY(Heightmap.Type heightmapType, int x, int z) {
|
||||
return region.getTopY(heightmapType, x, z);
|
||||
}
|
||||
|
||||
@Override
|
||||
public void playSound(PlayerEntity player, BlockPos pos, SoundEvent soundIn, SoundCategory category, float volume, float pitch) {
|
||||
region.playSound(player, pos, soundIn, category, volume, pitch);
|
||||
}
|
||||
|
||||
@Override
|
||||
public void addParticle(ParticleEffect particleData, double x, double y, double z, double xSpeed, double ySpeed, double zSpeed) {
|
||||
region.addParticle(particleData, x, y, z, xSpeed, ySpeed, zSpeed);
|
||||
}
|
||||
|
||||
@Override
|
||||
public void playLevelEvent(PlayerEntity player, int type, BlockPos pos, int data) {
|
||||
region.playLevelEvent(player, type, pos, data);
|
||||
}
|
||||
|
||||
@Override
|
||||
@Environment(EnvType.CLIENT)
|
||||
public BlockPos getSpawnPos() {
|
||||
return region.getSpawnPos();
|
||||
}
|
||||
|
||||
@Override
|
||||
public Dimension getDimension() {
|
||||
return region.getDimension();
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean testBlockState(BlockPos p_217375_1_, Predicate<BlockState> p_217375_2_) {
|
||||
return region.testBlockState(p_217375_1_, p_217375_2_);
|
||||
}
|
||||
|
||||
@Override
|
||||
public <T extends Entity> List<T> getEntities(Class<? extends T> clazz, Box aabb, Predicate<? super T> filter) {
|
||||
return region.getEntities(clazz, aabb, filter);
|
||||
}
|
||||
|
||||
@Override
|
||||
public List<Entity> getEntities(Entity entityIn, Box boundingBox, Predicate<? super Entity> predicate) {
|
||||
return region.getEntities(entityIn, boundingBox, predicate);
|
||||
}
|
||||
|
||||
@Override
|
||||
public List<PlayerEntity> getPlayers() {
|
||||
return region.getPlayers();
|
||||
}
|
||||
|
||||
@Override
|
||||
public float getMoonSize() {
|
||||
return region.getMoonSize();
|
||||
}
|
||||
|
||||
@Override
|
||||
public float getSkyAngle(float partialTicks) {
|
||||
return region.getSkyAngle(partialTicks);
|
||||
}
|
||||
|
||||
@Override
|
||||
@Environment(EnvType.CLIENT)
|
||||
public int getMoonPhase() {
|
||||
return region.getMoonPhase();
|
||||
}
|
||||
|
||||
@Override
|
||||
public Difficulty getDifficulty() {
|
||||
return region.getDifficulty();
|
||||
}
|
||||
|
||||
@Override
|
||||
public void playLevelEvent(int type, BlockPos pos, int data) {
|
||||
region.playLevelEvent(type, pos, data);
|
||||
}
|
||||
|
||||
@Override
|
||||
public Stream<VoxelShape> getEntityCollisions(Entity entityIn, Box aabb, Set<Entity> entitiesToIgnore) {
|
||||
return region.getEntityCollisions(entityIn, aabb, entitiesToIgnore);
|
||||
}
|
||||
|
||||
|
||||
@Override
|
||||
public boolean intersectsEntities(Entity entityIn, VoxelShape shape) {
|
||||
return region.intersectsEntities(entityIn, shape);
|
||||
}
|
||||
|
||||
@Override
|
||||
public BlockPos getTopPosition(Heightmap.Type heightmapType, BlockPos pos) {
|
||||
return region.getTopPosition(heightmapType, pos);
|
||||
}
|
||||
|
||||
@Override
|
||||
public <T extends Entity> List<T> getEntitiesIncludingUngeneratedChunks(Class<? extends T> entityClass, Box box, Predicate<? super T> predicate) {
|
||||
return region.getEntitiesIncludingUngeneratedChunks(entityClass, box, predicate);
|
||||
}
|
||||
|
||||
@Override
|
||||
public List<Entity> getEntities(Entity entityIn, Box bb) {
|
||||
return region.getEntities(entityIn, bb);
|
||||
}
|
||||
|
||||
@Override
|
||||
public <T extends Entity> List<T> getNonSpectatingEntities(Class<? extends T> p_217357_1_, Box p_217357_2_) {
|
||||
return region.getNonSpectatingEntities(p_217357_1_, p_217357_2_);
|
||||
}
|
||||
|
||||
@Override
|
||||
public <T extends Entity> List<T> getEntitiesIncludingUngeneratedChunks(Class<? extends T> p_225317_1_, Box p_225317_2_) {
|
||||
return region.getEntitiesIncludingUngeneratedChunks(p_225317_1_, p_225317_2_);
|
||||
}
|
||||
|
||||
@Override
|
||||
|
||||
public PlayerEntity getClosestPlayer(Entity entityIn, double distance) {
|
||||
return region.getClosestPlayer(entityIn, distance);
|
||||
}
|
||||
|
||||
@Override
|
||||
public PlayerEntity getClosestPlayer(double x, double y, double z, double distance, boolean creativePlayers) {
|
||||
return region.getClosestPlayer(x, y, z, distance, creativePlayers);
|
||||
}
|
||||
|
||||
@Override
|
||||
public PlayerEntity getClosestPlayer(double x, double y, double z) {
|
||||
return region.getClosestPlayer(x, y, z);
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean isPlayerInRange(double x, double y, double z, double distance) {
|
||||
return region.isPlayerInRange(x, y, z, distance);
|
||||
}
|
||||
|
||||
@Override
|
||||
public PlayerEntity getClosestPlayer(TargetPredicate predicate, LivingEntity target) {
|
||||
return region.getClosestPlayer(predicate, target);
|
||||
}
|
||||
|
||||
@Override
|
||||
public PlayerEntity getClosestPlayer(TargetPredicate predicate, LivingEntity target, double p_217372_3_, double p_217372_5_, double p_217372_7_) {
|
||||
return region.getClosestPlayer(predicate, target, p_217372_3_, p_217372_5_, p_217372_7_);
|
||||
}
|
||||
|
||||
@Override
|
||||
public PlayerEntity getClosestPlayer(TargetPredicate predicate, double x, double y, double z) {
|
||||
return region.getClosestPlayer(predicate, x, y, z);
|
||||
}
|
||||
|
||||
@Override
|
||||
public <T extends LivingEntity> T getClosestEntity(Class<? extends T> entityClazz, TargetPredicate p_217360_2_, LivingEntity target,
|
||||
double x, double y, double z, Box boundingBox) {
|
||||
return region.getClosestEntity(entityClazz, p_217360_2_, target, x, y, z, boundingBox);
|
||||
}
|
||||
|
||||
@Override
|
||||
public <T extends LivingEntity> T getClosestEntityIncludingUngeneratedChunks(Class<? extends T> p_225318_1_, TargetPredicate p_225318_2_,
|
||||
LivingEntity p_225318_3_,
|
||||
double p_225318_4_, double p_225318_6_, double p_225318_8_, Box p_225318_10_) {
|
||||
return region.getClosestEntityIncludingUngeneratedChunks(p_225318_1_, p_225318_2_, p_225318_3_, p_225318_4_, p_225318_6_, p_225318_8_,
|
||||
p_225318_10_);
|
||||
}
|
||||
|
||||
@Override
|
||||
|
||||
public <T extends LivingEntity> T getClosestEntity(List<? extends T> entities, TargetPredicate predicate, LivingEntity target, double x, double y,
|
||||
double z) {
|
||||
return region.getClosestEntity(entities, predicate, target, x, y, z);
|
||||
}
|
||||
|
||||
@Override
|
||||
public List<PlayerEntity> getPlayers(TargetPredicate predicate, LivingEntity target, Box box) {
|
||||
return region.getPlayers(predicate, target, box);
|
||||
}
|
||||
|
||||
@Override
|
||||
public <T extends LivingEntity> List<T> getTargets(Class<? extends T> p_217374_1_, TargetPredicate p_217374_2_,
|
||||
LivingEntity p_217374_3_, Box p_217374_4_) {
|
||||
return region.getTargets(p_217374_1_, p_217374_2_, p_217374_3_, p_217374_4_);
|
||||
}
|
||||
|
||||
@Override
|
||||
|
||||
public PlayerEntity getPlayerByUuid(UUID uniqueIdIn) {
|
||||
return region.getPlayerByUuid(uniqueIdIn);
|
||||
}
|
||||
|
||||
@Override
|
||||
public Biome getBiome(BlockPos p_226691_1_) {
|
||||
return region.getBiome(p_226691_1_);
|
||||
}
|
||||
|
||||
@Override
|
||||
@Environment(EnvType.CLIENT)
|
||||
public int getColor(BlockPos blockPosIn, ColorResolver colorResolverIn) {
|
||||
return region.getColor(blockPosIn, colorResolverIn);
|
||||
}
|
||||
|
||||
@Override
|
||||
public Biome getGeneratorStoredBiome(int biomeX, int biomeY, int biomeZ) {
|
||||
return region.getGeneratorStoredBiome(biomeX, biomeY, biomeZ);
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean isAir(BlockPos pos) {
|
||||
return region.isAir(pos);
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean isSkyVisible(BlockPos pos) {
|
||||
return region.isSkyVisible(pos);
|
||||
}
|
||||
|
||||
@Override
|
||||
@Deprecated
|
||||
public float getBrightness(BlockPos pos) {
|
||||
return region.getBrightness(pos);
|
||||
}
|
||||
|
||||
@Override
|
||||
public int getStrongRedstonePower(BlockPos pos, Direction direction) {
|
||||
return region.getStrongRedstonePower(pos, direction);
|
||||
}
|
||||
|
||||
@Override
|
||||
public Chunk getChunk(BlockPos pos) {
|
||||
return region.getChunk(pos);
|
||||
}
|
||||
|
||||
@Override
|
||||
public Chunk getChunk(int chunkX, int chunkZ, ChunkStatus requiredStatus) {
|
||||
return region.getChunk(chunkX, chunkZ, requiredStatus);
|
||||
}
|
||||
|
||||
@Override
|
||||
public BlockView getExistingChunk(int p_225522_1_, int p_225522_2_) {
|
||||
return region.getExistingChunk(p_225522_1_, p_225522_2_);
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean isWater(BlockPos pos) {
|
||||
return region.isWater(pos);
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean containsFluid(Box bb) {
|
||||
return region.containsFluid(bb);
|
||||
}
|
||||
|
||||
@Override
|
||||
public int getLightLevel(BlockPos pos) {
|
||||
return region.getLightLevel(pos);
|
||||
}
|
||||
|
||||
@Override
|
||||
public int getBaseLightLevel(BlockPos pos, int amount) {
|
||||
return region.getBaseLightLevel(pos, amount);
|
||||
}
|
||||
|
||||
@Override
|
||||
@Deprecated
|
||||
public boolean isChunkLoaded(BlockPos pos) {
|
||||
return region.isChunkLoaded(pos);
|
||||
}
|
||||
|
||||
|
||||
// @Override todo lol what is this
|
||||
// public boolean isRegionLoaded(BlockPos center, int range) {
|
||||
// return region.isRegionLoaded(center, range);
|
||||
// }
|
||||
|
||||
@Override
|
||||
@Deprecated
|
||||
public boolean isRegionLoaded(BlockPos from, BlockPos to) {
|
||||
return region.isRegionLoaded(from, to);
|
||||
}
|
||||
|
||||
@Override
|
||||
@Deprecated
|
||||
public boolean isRegionLoaded(int minX, int minY, int minZ, int maxX, int maxY, int maxZ) {
|
||||
return region.isRegionLoaded(minX, minY, minZ, maxX, maxY, maxZ);
|
||||
}
|
||||
|
||||
@Override
|
||||
public int getLightLevel(LightType lightTypeIn, BlockPos blockPosIn) {
|
||||
return region.getLightLevel(lightTypeIn, blockPosIn);
|
||||
}
|
||||
|
||||
@Override
|
||||
public int getLightLevel(BlockPos blockPosIn, int amount) {
|
||||
return region.getLightLevel(blockPosIn, amount);
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean isSkyVisibleAllowingSea(BlockPos pos) {
|
||||
return region.isSkyVisibleAllowingSea(pos);
|
||||
}
|
||||
|
||||
@Override
|
||||
public int getLuminance(BlockPos pos) {
|
||||
return region.getLuminance(pos);
|
||||
}
|
||||
|
||||
@Override
|
||||
public int getMaxLightLevel() {
|
||||
return region.getMaxLightLevel();
|
||||
}
|
||||
|
||||
@Override
|
||||
public int getHeight() {
|
||||
return region.getHeight();
|
||||
}
|
||||
|
||||
@Override
|
||||
public BlockHitResult rayTrace(RayTraceContext context) {
|
||||
return region.rayTrace(context);
|
||||
}
|
||||
|
||||
@Override
|
||||
public BlockHitResult rayTraceBlock(Vec3d p_217296_1_, Vec3d p_217296_2_, BlockPos p_217296_3_, VoxelShape p_217296_4_,
|
||||
BlockState p_217296_5_) {
|
||||
return region.rayTraceBlock(p_217296_1_, p_217296_2_, p_217296_3_, p_217296_4_, p_217296_5_);
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean canPlace(BlockState state, BlockPos pos, EntityContext context) {
|
||||
return region.canPlace(state, pos, context);
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean intersectsEntities(Entity entity) {
|
||||
return region.intersectsEntities(entity);
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean doesNotCollide(Box box) {
|
||||
return region.doesNotCollide(box);
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean doesNotCollide(Entity entity) {
|
||||
return region.doesNotCollide(entity);
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean doesNotCollide(Entity entity, Box box) {
|
||||
return region.doesNotCollide(entity, box);
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean doesNotCollide(Entity entity, Box entityBoundingBox, Set<Entity> otherEntities) {
|
||||
return region.doesNotCollide(entity, entityBoundingBox, otherEntities);
|
||||
}
|
||||
|
||||
@Override
|
||||
public Stream<VoxelShape> getCollisions(Entity entity, Box box, Set<Entity> excluded) {
|
||||
return region.getCollisions(entity, box, excluded);
|
||||
}
|
||||
|
||||
@Override
|
||||
public Stream<VoxelShape> getBlockCollisions(Entity entity, Box box) {
|
||||
return region.getBlockCollisions(entity, box);
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean breakBlock(BlockPos pos, boolean drop) {
|
||||
return region.breakBlock(pos, drop);
|
||||
}
|
||||
|
||||
|
||||
private static Chunk getMainChunk(ChunkRegion region) {
|
||||
int x = region.getCenterChunkX();
|
||||
int z = region.getCenterChunkZ();
|
||||
return region.getChunk(x, z);
|
||||
}
|
||||
}
|
@ -0,0 +1,50 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.chunk.fix;
|
||||
|
||||
import net.minecraft.world.ChunkRegion;
|
||||
import net.minecraft.world.gen.chunk.ChunkGenerator;
|
||||
|
||||
// fixes hard-coded water and world height values
|
||||
public class RegionFix extends RegionDelegate {
|
||||
|
||||
private final ChunkGenerator<?> generator;
|
||||
|
||||
public RegionFix(ChunkRegion region, ChunkGenerator<?> generator) {
|
||||
super(region.getWorld(), region);
|
||||
this.generator = generator;
|
||||
}
|
||||
|
||||
@Override
|
||||
public int getSeaLevel() {
|
||||
return generator.getSeaLevel();
|
||||
}
|
||||
|
||||
@Override
|
||||
public int getHeight() {
|
||||
return generator.getMaxY();
|
||||
}
|
||||
}
|
@ -0,0 +1,44 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.chunk.test;
|
||||
|
||||
import com.terraforged.core.world.terrain.Terrain;
|
||||
import com.terraforged.core.world.terrain.Terrains;
|
||||
import com.terraforged.mod.biome.ModBiomes;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
|
||||
public class Test {
|
||||
|
||||
public static boolean fixedBiome = true;
|
||||
|
||||
public static Terrain getTerrainType(Terrains terrains) {
|
||||
return terrains.mountains;
|
||||
}
|
||||
|
||||
public static Biome getBiome() {
|
||||
return ModBiomes.TAIGA_SCRUB;
|
||||
}
|
||||
}
|
@ -0,0 +1,44 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.chunk.test;
|
||||
|
||||
import com.terraforged.core.cell.Cell;
|
||||
import com.terraforged.core.world.terrain.Terrain;
|
||||
import com.terraforged.mod.biome.provider.BiomeProvider;
|
||||
import com.terraforged.mod.chunk.TerraContext;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
|
||||
public class TestBiomeProvider extends BiomeProvider {
|
||||
|
||||
public TestBiomeProvider(TerraContext chunkContext) {
|
||||
super(chunkContext);
|
||||
}
|
||||
|
||||
@Override
|
||||
public Biome getBiome(Cell<Terrain> cell, int x, int z) {
|
||||
return Test.getBiome();
|
||||
}
|
||||
}
|
@ -0,0 +1,86 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.chunk.test;
|
||||
|
||||
import com.terraforged.core.cell.Cell;
|
||||
import com.terraforged.core.cell.Populator;
|
||||
import com.terraforged.core.region.RegionCache;
|
||||
import com.terraforged.core.region.RegionGenerator;
|
||||
import com.terraforged.core.util.concurrent.ThreadPool;
|
||||
import com.terraforged.core.world.GeneratorContext;
|
||||
import com.terraforged.core.world.WorldGeneratorFactory;
|
||||
import com.terraforged.core.world.heightmap.WorldHeightmap;
|
||||
import com.terraforged.core.world.terrain.Terrain;
|
||||
import com.terraforged.mod.biome.provider.BiomeProvider;
|
||||
import com.terraforged.mod.chunk.TerraChunkGenerator;
|
||||
import com.terraforged.mod.chunk.TerraContext;
|
||||
import net.minecraft.world.gen.chunk.ChunkGeneratorConfig;
|
||||
|
||||
public class TestChunkGenerator extends TerraChunkGenerator {
|
||||
|
||||
private final BiomeProvider biomeProvider;
|
||||
|
||||
public TestChunkGenerator(TerraContext context, BiomeProvider biomeProvider, ChunkGeneratorConfig settings) {
|
||||
super(context, biomeProvider, settings);
|
||||
this.biomeProvider = new TestBiomeProvider(context);
|
||||
}
|
||||
|
||||
@Override
|
||||
protected RegionCache createRegionCache(TerraContext context) {
|
||||
return RegionGenerator.builder()
|
||||
.factory(new WorldGeneratorFactory(context, new TestHeightMap(context)))
|
||||
.pool(ThreadPool.getFixed())
|
||||
.size(3, 2)
|
||||
.build()
|
||||
.toCache(true);
|
||||
}
|
||||
|
||||
@Override
|
||||
public BiomeProvider getBiomeSource() {
|
||||
return biomeProvider;
|
||||
}
|
||||
|
||||
private static class TestHeightMap extends WorldHeightmap {
|
||||
|
||||
private final Populator populator;
|
||||
|
||||
public TestHeightMap(GeneratorContext context) {
|
||||
super(context);
|
||||
this.populator = getPopulator(Test.getTerrainType(context.terrain));
|
||||
}
|
||||
|
||||
@Override
|
||||
public void apply(Cell<Terrain> cell, float x, float y) {
|
||||
super.apply(cell, x, y);
|
||||
populator.apply(cell, x, y);
|
||||
}
|
||||
|
||||
@Override
|
||||
public void tag(Cell<Terrain> cell, float x, float y) {
|
||||
populator.tag(cell, x, y);
|
||||
}
|
||||
}
|
||||
}
|
@ -0,0 +1,272 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.command;
|
||||
|
||||
import com.mojang.brigadier.Command;
|
||||
import com.mojang.brigadier.CommandDispatcher;
|
||||
import com.mojang.brigadier.builder.LiteralArgumentBuilder;
|
||||
import com.mojang.brigadier.context.CommandContext;
|
||||
import com.mojang.brigadier.exceptions.CommandSyntaxException;
|
||||
import com.mojang.brigadier.exceptions.SimpleCommandExceptionType;
|
||||
import com.terraforged.core.cell.Cell;
|
||||
import com.terraforged.core.world.WorldGenerator;
|
||||
import com.terraforged.core.world.terrain.Terrain;
|
||||
import com.terraforged.core.world.terrain.Terrains;
|
||||
import com.terraforged.mod.biome.provider.BiomeProvider;
|
||||
import com.terraforged.mod.chunk.TerraChunkGenerator;
|
||||
import com.terraforged.mod.chunk.TerraContext;
|
||||
import com.terraforged.mod.command.arg.BiomeArgType;
|
||||
import com.terraforged.mod.command.arg.TerrainArgType;
|
||||
import com.terraforged.mod.command.search.BiomeSearchTask;
|
||||
import com.terraforged.mod.command.search.BothSearchTask;
|
||||
import com.terraforged.mod.command.search.Search;
|
||||
import com.terraforged.mod.command.search.TerrainSearchTask;
|
||||
import com.terraforged.mod.data.DataGen;
|
||||
import net.minecraft.entity.player.PlayerEntity;
|
||||
import net.minecraft.server.MinecraftServer;
|
||||
import net.minecraft.server.command.CommandManager;
|
||||
import net.minecraft.server.command.ServerCommandSource;
|
||||
import net.minecraft.server.network.ServerPlayerEntity;
|
||||
import net.minecraft.server.world.ServerWorld;
|
||||
import net.minecraft.text.ClickEvent;
|
||||
import net.minecraft.text.HoverEvent;
|
||||
import net.minecraft.text.LiteralText;
|
||||
import net.minecraft.text.Text;
|
||||
import net.minecraft.text.Texts;
|
||||
import net.minecraft.text.TranslatableText;
|
||||
import net.minecraft.util.Formatting;
|
||||
import net.minecraft.util.math.BlockPos;
|
||||
import net.minecraft.util.registry.Registry;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
import net.minecraft.world.biome.source.BiomeSource;
|
||||
import net.minecraft.world.biome.source.HorizontalVoronoiBiomeAccessType;
|
||||
import net.minecraft.world.dimension.DimensionType;
|
||||
import net.minecraft.world.gen.chunk.ChunkGenerator;
|
||||
|
||||
import java.util.Optional;
|
||||
import java.util.UUID;
|
||||
import java.util.concurrent.CompletableFuture;
|
||||
import java.util.function.Supplier;
|
||||
|
||||
public class TerraCommand {
|
||||
|
||||
public static void register(CommandDispatcher<ServerCommandSource> dispatcher) {
|
||||
dispatcher.register(command());
|
||||
}
|
||||
|
||||
private static LiteralArgumentBuilder<ServerCommandSource> command() {
|
||||
return CommandManager.literal("terra")
|
||||
.requires(source -> source.hasPermissionLevel(2))
|
||||
.then(CommandManager.literal("query")
|
||||
.executes(TerraCommand::query))
|
||||
.then(CommandManager.literal("data")
|
||||
.then(CommandManager.literal("dump")
|
||||
.executes(TerraCommand::dump)))
|
||||
.then(CommandManager.literal("debug")
|
||||
.executes(TerraCommand::debugBiome))
|
||||
.then(CommandManager.literal("locate")
|
||||
.then(CommandManager.argument("biome", BiomeArgType.biome())
|
||||
.executes(TerraCommand::findBiome)
|
||||
.then(CommandManager.argument("terrain", TerrainArgType.terrain())
|
||||
.executes(TerraCommand::findTerrainAndBiome)))
|
||||
.then(CommandManager.argument("terrain", TerrainArgType.terrain())
|
||||
.executes(TerraCommand::findTerrain)
|
||||
.then(CommandManager.argument("biome", BiomeArgType.biome())
|
||||
.executes(TerraCommand::findTerrainAndBiome))));
|
||||
}
|
||||
|
||||
private static int query(CommandContext<ServerCommandSource> context) throws CommandSyntaxException {
|
||||
getContext(context).orElseThrow(() -> createException(
|
||||
"Invalid world type",
|
||||
"This command can only be run in a TerraForged world!"
|
||||
));
|
||||
|
||||
BlockPos pos = context.getSource().getPlayer().getBlockPos();
|
||||
BiomeProvider biomeProvider = getBiomeProvider(context);
|
||||
Cell<Terrain> cell = biomeProvider.lookupPos(pos.getX(), pos.getZ());
|
||||
Biome biome = biomeProvider.getBiome(cell, pos.getX(), pos.getZ());
|
||||
context.getSource().sendFeedback(
|
||||
new LiteralText("Terrain=" + cell.tag.getName() + ", Biome=" + Registry.BIOME.getId(biome)),
|
||||
false
|
||||
);
|
||||
|
||||
return Command.SINGLE_SUCCESS;
|
||||
}
|
||||
|
||||
private static int dump(CommandContext<ServerCommandSource> context) throws CommandSyntaxException {
|
||||
context.getSource().sendFeedback(
|
||||
new LiteralText("Exporting data"),
|
||||
true
|
||||
);
|
||||
DataGen.dumpData();
|
||||
return Command.SINGLE_SUCCESS;
|
||||
}
|
||||
|
||||
private static int debugBiome(CommandContext<ServerCommandSource> context) throws CommandSyntaxException {
|
||||
ServerPlayerEntity player = context.getSource().getPlayer();
|
||||
BlockPos position = player.getBlockPos();
|
||||
int x = position.getX();
|
||||
int y = position.getY();
|
||||
int z = position.getZ();
|
||||
|
||||
long seed = player.getServerWorld().getSeed();
|
||||
Biome actual = player.getServerWorld().getBiome(position);
|
||||
Biome biome2 = HorizontalVoronoiBiomeAccessType.INSTANCE
|
||||
.getBiome(seed, x, 0, z, player.getServerWorld().getChunkManager().getChunkGenerator().getBiomeSource());
|
||||
|
||||
context.getSource().sendFeedback(new LiteralText(
|
||||
"Actual Biome = " + Registry.BIOME.getId(actual)
|
||||
+ "\nLookup Biome = " + Registry.BIOME.getId(biome2)),
|
||||
false
|
||||
);
|
||||
|
||||
return Command.SINGLE_SUCCESS;
|
||||
}
|
||||
|
||||
private static int findTerrain(CommandContext<ServerCommandSource> context) throws CommandSyntaxException {
|
||||
// get the generator's context
|
||||
TerraContext terraContext = getContext(context).orElseThrow(() -> createException(
|
||||
"Invalid world type",
|
||||
"This command can only be run in a TerraForged world!"
|
||||
));
|
||||
|
||||
Terrain terrain = TerrainArgType.getTerrain(context, "terrain");
|
||||
Terrain target = getTerrainInstance(terrain, terraContext.terrain);
|
||||
BlockPos pos = context.getSource().getPlayer().getBlockPos();
|
||||
UUID playerID = context.getSource().getPlayer().getUuid();
|
||||
MinecraftServer server = context.getSource().getMinecraftServer();
|
||||
WorldGenerator worldGenerator = terraContext.factory.get();
|
||||
Search search = new TerrainSearchTask(pos, worldGenerator, target);
|
||||
doSearch(server, playerID, search);
|
||||
context.getSource().sendFeedback(new LiteralText("Searching..."), false);
|
||||
|
||||
return Command.SINGLE_SUCCESS;
|
||||
}
|
||||
|
||||
private static int findBiome(CommandContext<ServerCommandSource> context) throws CommandSyntaxException {
|
||||
// get the generator's context
|
||||
TerraContext terraContext = getContext(context).orElseThrow(() -> createException(
|
||||
"Invalid world type",
|
||||
"This command can only be run in a TerraForged world!"
|
||||
));
|
||||
|
||||
Biome biome = BiomeArgType.getBiome(context, "biome");
|
||||
BlockPos pos = context.getSource().getPlayer().getBlockPos();
|
||||
UUID playerID = context.getSource().getPlayer().getUuid();
|
||||
MinecraftServer server = context.getSource().getMinecraftServer();
|
||||
ServerWorld reader = context.getSource().getPlayer().getServerWorld();
|
||||
Search search = new BiomeSearchTask(pos, reader, biome);
|
||||
doSearch(server, playerID, search);
|
||||
context.getSource().sendFeedback(new LiteralText("Searching..."), false);
|
||||
|
||||
return Command.SINGLE_SUCCESS;
|
||||
}
|
||||
|
||||
private static int findTerrainAndBiome(CommandContext<ServerCommandSource> context) throws CommandSyntaxException {
|
||||
// get the generator's context
|
||||
TerraContext terraContext = getContext(context).orElseThrow(() -> createException(
|
||||
"Invalid world type",
|
||||
"This command can only be run in a TerraForged world!"
|
||||
));
|
||||
|
||||
Terrain terrain = TerrainArgType.getTerrain(context, "terrain");
|
||||
Terrain target = getTerrainInstance(terrain, terraContext.terrain);
|
||||
Biome biome = BiomeArgType.getBiome(context, "biome");
|
||||
BlockPos pos = context.getSource().getPlayer().getBlockPos();
|
||||
ServerWorld world = context.getSource().getPlayer().getServerWorld();
|
||||
UUID playerID = context.getSource().getPlayer().getUuid();
|
||||
MinecraftServer server = context.getSource().getMinecraftServer();
|
||||
WorldGenerator worldGenerator = terraContext.factory.get();
|
||||
Search biomeSearch = new BiomeSearchTask(pos, world, biome);
|
||||
Search terrainSearch = new TerrainSearchTask(pos, worldGenerator, target);
|
||||
Search search = new BothSearchTask(pos, biomeSearch, terrainSearch);
|
||||
doSearch(server, playerID, search);
|
||||
context.getSource().sendFeedback(new LiteralText("Searching..."), false);
|
||||
|
||||
return Command.SINGLE_SUCCESS;
|
||||
}
|
||||
|
||||
private static void doSearch(MinecraftServer server, UUID userId, Supplier<BlockPos> supplier) {
|
||||
CompletableFuture.supplyAsync(supplier).thenAcceptAsync(pos -> {
|
||||
PlayerEntity player = server.getPlayerManager().getPlayer(userId);
|
||||
if (player == null) {
|
||||
return;
|
||||
}
|
||||
|
||||
if (pos.getX() == 0 && pos.getZ() == 0) {
|
||||
player.sendMessage(new LiteralText("Location not found :["));
|
||||
return;
|
||||
}
|
||||
|
||||
Text result = new LiteralText("Nearest match: ")
|
||||
.append(createTeleportMessage(pos));
|
||||
|
||||
player.sendMessage(result);
|
||||
}, server);
|
||||
}
|
||||
|
||||
private static Optional<TerraContext> getContext(CommandContext<ServerCommandSource> context) throws CommandSyntaxException {
|
||||
MinecraftServer server = context.getSource().getMinecraftServer();
|
||||
DimensionType dimension = context.getSource().getPlayer().dimension;
|
||||
ChunkGenerator<?> generator = server.getWorld(dimension).getChunkManager().getChunkGenerator();
|
||||
if (generator instanceof TerraChunkGenerator) {
|
||||
TerraChunkGenerator gen = (TerraChunkGenerator) generator;
|
||||
return Optional.of(gen.getContext());
|
||||
}
|
||||
return Optional.empty();
|
||||
}
|
||||
|
||||
// the terrain parsed from the command will not be the same instance as used in the
|
||||
// world generator, so find the matching instance by name
|
||||
private static Terrain getTerrainInstance(Terrain find, Terrains terrains) {
|
||||
for (Terrain t : terrains.index) {
|
||||
if (t.getName().equals(find.getName())) {
|
||||
return t;
|
||||
}
|
||||
}
|
||||
return find;
|
||||
}
|
||||
|
||||
private static BiomeProvider getBiomeProvider(CommandContext<ServerCommandSource> context) {
|
||||
return (BiomeProvider) context.getSource().getWorld().getChunkManager().getChunkGenerator().getBiomeSource();
|
||||
}
|
||||
|
||||
private static CommandSyntaxException createException(String type, String message, Object... args) {
|
||||
return new CommandSyntaxException(
|
||||
new SimpleCommandExceptionType(new LiteralText(type)),
|
||||
new LiteralText(String.format(message, args))
|
||||
);
|
||||
}
|
||||
|
||||
private static Text createTeleportMessage(BlockPos pos) {
|
||||
return Texts.bracketed(new TranslatableText(
|
||||
"chat.coordinates", pos.getX(), "~", pos.getZ()
|
||||
)).styled((style) -> style.setColor(Formatting.GREEN)
|
||||
.setClickEvent(new ClickEvent(ClickEvent.Action.SUGGEST_COMMAND, "/tp @s " + pos.getX() + " ~ " + pos.getZ()))
|
||||
.setHoverEvent(new HoverEvent(HoverEvent.Action.SHOW_TEXT, new TranslatableText("chat.coordinates.tooltip")))
|
||||
);
|
||||
}
|
||||
}
|
@ -0,0 +1,71 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.command.arg;
|
||||
|
||||
import com.mojang.brigadier.StringReader;
|
||||
import com.mojang.brigadier.arguments.ArgumentType;
|
||||
import com.mojang.brigadier.context.CommandContext;
|
||||
import com.mojang.brigadier.exceptions.CommandSyntaxException;
|
||||
import com.mojang.brigadier.exceptions.SimpleCommandExceptionType;
|
||||
import com.mojang.brigadier.suggestion.Suggestions;
|
||||
import com.mojang.brigadier.suggestion.SuggestionsBuilder;
|
||||
import net.minecraft.server.command.CommandSource;
|
||||
import net.minecraft.text.LiteralText;
|
||||
import net.minecraft.util.Identifier;
|
||||
import net.minecraft.util.registry.Registry;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
|
||||
import java.util.concurrent.CompletableFuture;
|
||||
|
||||
public class BiomeArgType implements ArgumentType<Biome> {
|
||||
|
||||
@Override
|
||||
public Biome parse(StringReader reader) throws CommandSyntaxException {
|
||||
Identifier resourcelocation = Identifier.fromCommandInput(reader);
|
||||
return Registry.BIOME.getOrEmpty(resourcelocation)
|
||||
.orElseThrow(() -> createException("Invalid biome", "%s is not a valid biome", resourcelocation));
|
||||
}
|
||||
|
||||
@Override
|
||||
public <S> CompletableFuture<Suggestions> listSuggestions(CommandContext<S> context, SuggestionsBuilder suggestions) {
|
||||
return CommandSource.suggestIdentifiers(Registry.BIOME.getIds(), suggestions);
|
||||
}
|
||||
|
||||
private static CommandSyntaxException createException(String type, String message, Object... args) {
|
||||
return new CommandSyntaxException(
|
||||
new SimpleCommandExceptionType(new LiteralText(type)),
|
||||
new LiteralText(String.format(message, args))
|
||||
);
|
||||
}
|
||||
|
||||
public static ArgumentType<Biome> biome() {
|
||||
return new BiomeArgType();
|
||||
}
|
||||
|
||||
public static <S> Biome getBiome(CommandContext<S> context, String name) {
|
||||
return context.getArgument(name, Biome.class);
|
||||
}
|
||||
}
|
@ -0,0 +1,116 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.command.arg;
|
||||
|
||||
import com.mojang.brigadier.StringReader;
|
||||
import com.mojang.brigadier.arguments.ArgumentType;
|
||||
import com.mojang.brigadier.context.CommandContext;
|
||||
import com.mojang.brigadier.exceptions.CommandSyntaxException;
|
||||
import com.mojang.brigadier.exceptions.SimpleCommandExceptionType;
|
||||
import com.mojang.brigadier.suggestion.Suggestions;
|
||||
import com.mojang.brigadier.suggestion.SuggestionsBuilder;
|
||||
import com.terraforged.core.settings.Settings;
|
||||
import com.terraforged.core.world.terrain.Terrain;
|
||||
import com.terraforged.core.world.terrain.Terrains;
|
||||
import net.minecraft.server.command.CommandSource;
|
||||
import net.minecraft.text.LiteralText;
|
||||
|
||||
import java.util.ArrayList;
|
||||
import java.util.List;
|
||||
import java.util.concurrent.CompletableFuture;
|
||||
|
||||
public class TerrainArgType implements ArgumentType<Terrain> {
|
||||
|
||||
private final List<Terrain> terrains = getTerrains();
|
||||
|
||||
@Override
|
||||
public Terrain parse(StringReader reader) throws CommandSyntaxException {
|
||||
int cursor = reader.getCursor();
|
||||
String name = reader.readString();
|
||||
for (Terrain terrain : terrains) {
|
||||
if (terrain.getName().equalsIgnoreCase(name)) {
|
||||
return terrain;
|
||||
}
|
||||
}
|
||||
reader.setCursor(cursor);
|
||||
throw createException("Invalid terrain", "%s is not a valid terrain type", name);
|
||||
}
|
||||
|
||||
@Override
|
||||
public <S> CompletableFuture<Suggestions> listSuggestions(CommandContext<S> context, SuggestionsBuilder suggestions) {
|
||||
return CommandSource.suggestMatching(terrains.stream().map(Terrain::getName), suggestions);
|
||||
}
|
||||
|
||||
public static ArgumentType<Terrain> terrain() {
|
||||
return new TerrainArgType();
|
||||
}
|
||||
|
||||
public static <S> Terrain getTerrain(CommandContext<S> context, String name) {
|
||||
return context.getArgument(name, Terrain.class);
|
||||
}
|
||||
|
||||
private static CommandSyntaxException createException(String type, String message, Object... args) {
|
||||
return new CommandSyntaxException(
|
||||
new SimpleCommandExceptionType(new LiteralText(type)),
|
||||
new LiteralText(String.format(message, args))
|
||||
);
|
||||
}
|
||||
|
||||
private static List<Terrain> getTerrains() {
|
||||
Terrains terrains = Terrains.create(new Settings());
|
||||
List<Terrain> result = new ArrayList<>();
|
||||
|
||||
for (int i = 0; i < terrains.index.size(); i++) {
|
||||
Terrain terrain = terrains.index.get(i);
|
||||
result.add(terrain);
|
||||
if (dontMix(terrain, terrains)) {
|
||||
continue;
|
||||
}
|
||||
for (int j = i + 1; j < terrains.index.size(); j++) {
|
||||
Terrain other = terrains.index.get(j);
|
||||
if (dontMix(other, terrains)) {
|
||||
continue;
|
||||
}
|
||||
Terrain mix = new Terrain(terrain.getName() + "-" + other.getName(), -1);
|
||||
result.add(mix);
|
||||
}
|
||||
}
|
||||
|
||||
return result;
|
||||
}
|
||||
|
||||
private static boolean dontMix(Terrain terrain, Terrains terrains) {
|
||||
return terrain == terrains.ocean
|
||||
|| terrain == terrains.deepOcean
|
||||
|| terrain == terrains.river
|
||||
|| terrain == terrains.riverBanks
|
||||
|| terrain == terrains.beach
|
||||
|| terrain == terrains.coast
|
||||
|| terrain == terrains.volcano
|
||||
|| terrain == terrains.volcanoPipe
|
||||
|| terrain == terrains.lake;
|
||||
}
|
||||
}
|
@ -0,0 +1,27 @@
|
||||
package com.terraforged.mod.command.search;
|
||||
|
||||
import net.minecraft.util.math.BlockPos;
|
||||
import net.minecraft.world.WorldView;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
|
||||
public class BiomeSearchTask extends Search {
|
||||
|
||||
private final Biome biome;
|
||||
private final WorldView reader;
|
||||
|
||||
public BiomeSearchTask(BlockPos center, WorldView reader, Biome biome) {
|
||||
super(center, 128);
|
||||
this.reader = reader;
|
||||
this.biome = biome;
|
||||
}
|
||||
|
||||
@Override
|
||||
public int getSpacing() {
|
||||
return 10;
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean test(BlockPos pos) {
|
||||
return reader.getGeneratorStoredBiome(pos.getX() >> 2, pos.getY(), pos.getZ() >> 2) == biome;
|
||||
}
|
||||
}
|
@ -0,0 +1,27 @@
|
||||
package com.terraforged.mod.command.search;
|
||||
|
||||
import net.minecraft.util.math.BlockPos;
|
||||
|
||||
public class BothSearchTask extends Search {
|
||||
|
||||
private final int spacing;
|
||||
private final Search a;
|
||||
private final Search b;
|
||||
|
||||
public BothSearchTask(BlockPos center, Search a, Search b) {
|
||||
super(center, Math.min(a.getMinRadius(), b.getMinRadius()));
|
||||
this.spacing = Math.min(a.getSpacing(), b.getSpacing());
|
||||
this.a = a;
|
||||
this.b = b;
|
||||
}
|
||||
|
||||
@Override
|
||||
public int getSpacing() {
|
||||
return spacing;
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean test(BlockPos pos) {
|
||||
return a.test(pos) && b.test(pos);
|
||||
}
|
||||
}
|
@ -0,0 +1,84 @@
|
||||
package com.terraforged.mod.command.search;
|
||||
|
||||
import net.minecraft.util.math.BlockPos;
|
||||
|
||||
import java.util.concurrent.TimeUnit;
|
||||
import java.util.function.Supplier;
|
||||
|
||||
public abstract class Search implements Supplier<BlockPos> {
|
||||
|
||||
protected static final int MIN_RADIUS = 128;
|
||||
protected static final int MAX_RADIUS = 24000;
|
||||
|
||||
private final BlockPos center;
|
||||
private final int minRadius;
|
||||
private final int maxRadius;
|
||||
private final double minRadius2;
|
||||
|
||||
public Search(BlockPos center) {
|
||||
this(center, MIN_RADIUS);
|
||||
}
|
||||
|
||||
public Search(BlockPos center, int minRadius) {
|
||||
this(center, minRadius, MAX_RADIUS);
|
||||
}
|
||||
|
||||
public Search(BlockPos center, int minRadius, int maxRadius) {
|
||||
this.center = center;
|
||||
this.minRadius = minRadius;
|
||||
this.minRadius2 = minRadius * minRadius;
|
||||
this.maxRadius = Math.min(maxRadius, MAX_RADIUS);
|
||||
}
|
||||
|
||||
public int getMinRadius() {
|
||||
return minRadius;
|
||||
}
|
||||
|
||||
public int getSpacing() {
|
||||
return 16;
|
||||
}
|
||||
|
||||
@Override
|
||||
public BlockPos get() {
|
||||
int radius = maxRadius;
|
||||
|
||||
int x = 0;
|
||||
int z = 0;
|
||||
int dx = 0;
|
||||
int dz = -1;
|
||||
int size = radius + 1 + radius;
|
||||
long max = (long) size * (long) size;
|
||||
long timeOut = System.currentTimeMillis() + TimeUnit.SECONDS.toMillis(30);
|
||||
BlockPos.Mutable pos = new BlockPos.Mutable();
|
||||
|
||||
for (long i = 0; i < max; i++) {
|
||||
if (System.currentTimeMillis() > timeOut) {
|
||||
break;
|
||||
}
|
||||
|
||||
if ((-radius <= x) && (x <= radius) && (-radius <= z) && (z <= radius)) {
|
||||
pos.set(center.getX() + (x * getSpacing()), center.getY(), center.getZ() + (z * getSpacing()));
|
||||
if (center.getSquaredDistance(pos) >= minRadius2) {
|
||||
if (test(pos)) {
|
||||
return pos.toImmutable();
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
if ((x == z) || ((x < 0) && (x == -z)) || ((x > 0) && (x == 1 - z))) {
|
||||
size = dx;
|
||||
dx = -dz;
|
||||
dz = size;
|
||||
}
|
||||
|
||||
x += dx;
|
||||
z += dz;
|
||||
}
|
||||
|
||||
System.out.println("LAST POS: " + pos);
|
||||
|
||||
return BlockPos.ORIGIN;
|
||||
}
|
||||
|
||||
public abstract boolean test(BlockPos pos);
|
||||
}
|
@ -0,0 +1,30 @@
|
||||
package com.terraforged.mod.command.search;
|
||||
|
||||
import com.terraforged.core.cell.Cell;
|
||||
import com.terraforged.core.world.WorldGenerator;
|
||||
import com.terraforged.core.world.terrain.Terrain;
|
||||
import net.minecraft.util.math.BlockPos;
|
||||
|
||||
public class TerrainSearchTask extends Search {
|
||||
|
||||
private final Terrain type;
|
||||
private final WorldGenerator generator;
|
||||
private final Cell<Terrain> cell = new Cell<>();
|
||||
|
||||
public TerrainSearchTask(BlockPos center, WorldGenerator generator, Terrain type) {
|
||||
super(center, 256);
|
||||
this.type = type;
|
||||
this.generator = generator;
|
||||
}
|
||||
|
||||
@Override
|
||||
public int getSpacing() {
|
||||
return 20;
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean test(BlockPos pos) {
|
||||
generator.getHeightmap().apply(cell, pos.getX(), pos.getZ());
|
||||
return cell.tag == type;
|
||||
}
|
||||
}
|
@ -0,0 +1,72 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.data;
|
||||
|
||||
import com.google.gson.GsonBuilder;
|
||||
import com.google.gson.JsonElement;
|
||||
import net.minecraft.util.Identifier;
|
||||
|
||||
import java.io.BufferedWriter;
|
||||
import java.io.File;
|
||||
import java.io.FileWriter;
|
||||
import java.io.IOException;
|
||||
import java.io.Writer;
|
||||
|
||||
public class DataGen {
|
||||
|
||||
public static void dumpData() {
|
||||
File dataDir = new File("data");
|
||||
WorldGenBiomes.genBiomeMap(dataDir);
|
||||
WorldGenBlocks.genBlockTags(dataDir);
|
||||
WorldGenFeatures.genBiomeFeatures(dataDir);
|
||||
}
|
||||
|
||||
protected static void write(File file, IOConsumer consumer) {
|
||||
if (file.getParentFile().exists() || file.getParentFile().mkdirs()) {
|
||||
try (Writer writer = new BufferedWriter(new FileWriter(file))) {
|
||||
consumer.accept(writer);
|
||||
} catch (IOException e) {
|
||||
e.printStackTrace();
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
protected static void write(JsonElement json, Writer writer) {
|
||||
new GsonBuilder().setPrettyPrinting().create().toJson(json, writer);
|
||||
}
|
||||
|
||||
protected static String getJsonPath(String type, Identifier location) {
|
||||
if (location == null) {
|
||||
return "unknown";
|
||||
}
|
||||
return location.getNamespace() + "/" + type + "/" + location.getPath() + ".json";
|
||||
}
|
||||
|
||||
public interface IOConsumer {
|
||||
|
||||
void accept(Writer writer) throws IOException;
|
||||
}
|
||||
}
|
@ -0,0 +1,83 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.data;
|
||||
|
||||
import com.google.gson.GsonBuilder;
|
||||
import com.google.gson.JsonArray;
|
||||
import com.google.gson.JsonObject;
|
||||
import com.terraforged.core.world.biome.BiomeType;
|
||||
import com.terraforged.mod.biome.map.BiomeMap;
|
||||
import com.terraforged.mod.biome.provider.BiomeHelper;
|
||||
import net.minecraft.util.Identifier;
|
||||
import net.minecraft.util.registry.Registry;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
|
||||
import java.io.BufferedWriter;
|
||||
import java.io.File;
|
||||
import java.io.FileWriter;
|
||||
import java.io.IOException;
|
||||
import java.io.Writer;
|
||||
import java.util.Set;
|
||||
|
||||
public class WorldGenBiomes extends DataGen {
|
||||
|
||||
public static void genBiomeMap(File dataDir) {
|
||||
BiomeMap map = BiomeHelper.getDefaultBiomeMap();
|
||||
for (BiomeType type : BiomeType.values()) {
|
||||
genBiomes(dataDir, type, map);
|
||||
}
|
||||
|
||||
try (Writer writer = new BufferedWriter(new FileWriter(new File("biome_map.json")))) {
|
||||
new GsonBuilder().setPrettyPrinting().create().toJson(map.toJson(), writer);
|
||||
} catch (IOException e) {
|
||||
e.printStackTrace();
|
||||
}
|
||||
}
|
||||
|
||||
private static void genBiomes(File dir, BiomeType type, BiomeMap map) {
|
||||
String path = getJsonPath("tags/biomes", getName(type));
|
||||
|
||||
write(new File(dir, path), writer -> {
|
||||
Set<Biome> biomes = map.getBiomes(type);
|
||||
JsonObject root = new JsonObject();
|
||||
JsonArray values = new JsonArray();
|
||||
|
||||
root.addProperty("replaceable", false);
|
||||
root.add("values", values);
|
||||
|
||||
biomes.stream()
|
||||
.map(b -> String.valueOf(Registry.BIOME.getId(b)))
|
||||
.sorted()
|
||||
.forEach(values::add);
|
||||
|
||||
write(root, writer);
|
||||
});
|
||||
}
|
||||
|
||||
private static Identifier getName(BiomeType type) {
|
||||
return new Identifier("terraforged", type.name().toLowerCase());
|
||||
}
|
||||
}
|
@ -0,0 +1,64 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.data;
|
||||
|
||||
import com.google.gson.JsonArray;
|
||||
import com.google.gson.JsonObject;
|
||||
import com.terraforged.mod.material.Materials;
|
||||
import net.minecraft.block.Block;
|
||||
import net.minecraft.util.Identifier;
|
||||
import net.minecraft.util.registry.Registry;
|
||||
|
||||
import java.io.File;
|
||||
import java.util.Collection;
|
||||
|
||||
public class WorldGenBlocks extends DataGen {
|
||||
|
||||
public static void genBlockTags(File dataDir) {
|
||||
if (dataDir.exists() || dataDir.mkdirs()) {
|
||||
Materials materials = new Materials();
|
||||
printMaterials(dataDir, "stone", materials.getStone());
|
||||
printMaterials(dataDir, "dirt", materials.getDirt());
|
||||
printMaterials(dataDir, "clay", materials.getClay());
|
||||
printMaterials(dataDir, "sediment",materials.getSediment());
|
||||
printMaterials(dataDir, "ore", materials.getOre());
|
||||
}
|
||||
}
|
||||
|
||||
private static void printMaterials(File dir, String name, Collection<Block> blocks) {
|
||||
String path = getJsonPath("tags/blocks", new Identifier("terraforged", name));
|
||||
write(new File(dir, path), writer -> {
|
||||
JsonObject root = new JsonObject();
|
||||
JsonArray values = new JsonArray();
|
||||
root.addProperty("replace", false);
|
||||
root.add("values", values);
|
||||
for (Block block : blocks) {
|
||||
values.add(String.valueOf(Registry.BLOCK.getId(block)));
|
||||
}
|
||||
write(root, writer);
|
||||
});
|
||||
}
|
||||
}
|
@ -0,0 +1,68 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.data;
|
||||
|
||||
import com.google.gson.JsonArray;
|
||||
import com.google.gson.JsonElement;
|
||||
import com.google.gson.JsonObject;
|
||||
import com.mojang.datafixers.Dynamic;
|
||||
import com.mojang.datafixers.types.JsonOps;
|
||||
import net.minecraft.util.registry.Registry;
|
||||
import net.minecraft.world.biome.Biome;
|
||||
import net.minecraft.world.gen.GenerationStep;
|
||||
import net.minecraft.world.gen.feature.ConfiguredFeature;
|
||||
|
||||
import java.io.File;
|
||||
|
||||
public class WorldGenFeatures extends DataGen {
|
||||
|
||||
public static void genBiomeFeatures(File dataDir) {
|
||||
if (dataDir.exists() || dataDir.mkdirs()) {
|
||||
for (Biome biome : Registry.BIOME) {
|
||||
genBiomeFeatures(dataDir, biome);
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
private static void genBiomeFeatures(File dir, Biome biome) {
|
||||
write(new File(dir, getJsonPath("features", Registry.BIOME.getId(biome))), writer -> {
|
||||
JsonObject root = new JsonObject();
|
||||
for (GenerationStep.Feature type : GenerationStep.Feature.values()) {
|
||||
JsonArray features = new JsonArray();
|
||||
for (ConfiguredFeature<?, ?> feature : biome.getFeaturesForStep(type)) {
|
||||
try {
|
||||
Dynamic<JsonElement> dynamic = feature.serialize(JsonOps.INSTANCE);
|
||||
features.add(dynamic.getValue());
|
||||
} catch (NullPointerException e) {
|
||||
new NullPointerException("Badly written feature: " + Registry.FEATURE.getId(feature.feature)).printStackTrace();
|
||||
}
|
||||
}
|
||||
root.add(type.getName(), features);
|
||||
}
|
||||
write(root, writer);
|
||||
});
|
||||
}
|
||||
}
|
@ -0,0 +1,49 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.decorator;
|
||||
|
||||
import com.terraforged.api.chunk.column.ColumnDecorator;
|
||||
import com.terraforged.api.chunk.column.DecoratorContext;
|
||||
import com.terraforged.api.material.state.States;
|
||||
import net.minecraft.world.chunk.IChunk;
|
||||
|
||||
public class ChunkPopulator implements ColumnDecorator {
|
||||
|
||||
public static final ChunkPopulator INSTANCE = new ChunkPopulator();
|
||||
|
||||
@Override
|
||||
public void decorate(IChunk chunk, DecoratorContext context, int x, int y, int z) {
|
||||
if (context.cell.tag == context.terrains.volcanoPipe && context.cell.riverMask > 0.25F) {
|
||||
int lavaStart = Math.max(context.levels.waterY + 10, y - 30);
|
||||
int lavaEnd = Math.max(5, context.levels.waterY - 10);
|
||||
fillDown(context, chunk, x, z, lavaStart, lavaEnd, States.LAVA.get());
|
||||
y = lavaEnd;
|
||||
} else if (y < context.levels.waterLevel) {
|
||||
fillDown(context, chunk, x, z, context.levels.waterY, y, States.WATER.get());
|
||||
}
|
||||
fillDown(context, chunk, x, z, y, 0, States.STONE.get());
|
||||
}
|
||||
}
|
@ -0,0 +1,43 @@
|
||||
/*
|
||||
*
|
||||
* MIT License
|
||||
*
|
||||
* Copyright (c) 2020 TerraForged
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
* SOFTWARE.
|
||||
*/
|
||||
|
||||
package com.terraforged.mod.decorator.base;
|
||||
|
||||
import com.terraforged.api.chunk.column.ColumnDecorator;
|
||||
import com.terraforged.api.chunk.column.DecoratorContext;
|
||||
import com.terraforged.api.material.state.States;
|
||||
import net.minecraft.world.chunk.IChunk;
|
||||
|
||||
import java.util.Random;
|
||||
|
||||
public class BedrockDecorator implements ColumnDecorator {
|
||||
|
||||
private final Random random = new Random();
|
||||
|
||||
@Override
|
||||
public void decorate(IChunk chunk, DecoratorContext context, int x, int y, int z) {
|
||||
fillDown(context, chunk, x, z, 1 + random.nextInt(4), -1, States.BEDROCK.get());
|
||||
}
|
||||
}
|
Some files were not shown because too many files have changed in this diff Show More
Loading…
Reference in New Issue
Block a user